PyTorch DataLoader: Features, Benefits, and How to Use it
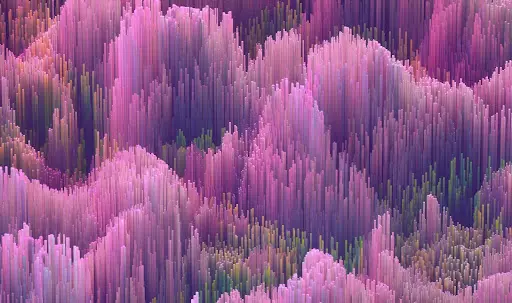
Photo credit: Maxim Berg
Introduction:
Deep learning models require large amounts of data to train effectively. In many cases, the data is stored in separate files, databases, or other external sources that need to be preprocessed and loaded into memory before training.
PyTorch is a popular open-source machine learning library that is widely used in research and industry. It is known for its ease of use, flexibility, and speed, making it a popular choice for building deep learning models.
One of the key features of PyTorch is the DataLoader class, which is used for loading and preprocessing data for deep learning models. In this blog post, we will discuss the PyTorch DataLoader class in detail, including its features, benefits, and how to use it to load and preprocess data for deep learning models.
PyTorch DataLoader:
The PyTorch DataLoader class is a utility class that is used to load data from a dataset and create mini-batches for training deep learning models. It is designed to handle large datasets and perform data augmentation, shuffling, and other preprocessing tasks.
The DataLoader class is part of the PyTorch data loading library, which includes other classes such as Dataset, Sampler, and BatchSampler. These classes work together to create efficient and flexible data loading pipelines for deep learning models.
Why use the PyTorch DataLoader class?
There are several benefits of using the PyTorch DataLoader class:
Efficient data loading: The DataLoader class provides efficient data loading by allowing the user to load data in parallel using multiple CPU cores. This can significantly reduce the data loading time, especially for large datasets.
Data augmentation: The DataLoader class provides data augmentation capabilities, allowing the user to apply various transformations to the data on the fly. This can help increase the size of the training set and improve the generalization of the model.
Flexibility: The DataLoader class is highly flexible and can handle a wide variety of data formats and sources. It supports loading data from files, databases, and other external sources, as well as pre-processing data using custom functions.
Shuffling: The DataLoader class provides shuffling capabilities, allowing the user to shuffle the data for each epoch. This can help prevent the model from overfitting to the training data and improve its generalization..
The basic architecture of PyTorch DataLoader
The PyTorch DataLoader class is built on top of the PyTorch Dataset class, which provides a standard interface for accessing data. The DataLoader class takes in a Dataset object and provides a way to iterate over the data in batches. This allows for efficient processing of large datasets by allowing parallelization of data loading and preprocessing.
The basic architecture of PyTorch DataLoader consists of the following components:
Dataset
A dataset is an abstract class in PyTorch that represents a collection of data. It is responsible for loading and preprocessing data from a source and returning it in the form of a PyTorch tensor.
The Dataset class provides two main methods:
len
: returns the length of the datasetgetitem
: returns a single data point from the dataset at a given index
The getitem
method is where the actual data loading and preprocessing takes place. It takes an index as input and returns a data point, which can be a tensor or a dictionary of tensors. This method is used by the DataLoader class to load and preprocess the data.
DataLoader
The DataLoader is a PyTorch utility class that provides a way to iterate over a Dataset object in batches. It is designed to handle large datasets efficiently and can be configured to load data in parallel, preprocess data on the fly, and shuffle data for each epoch.
The DataLoader takes in a Dataset object and provides a number of configuration options, including batch size, shuffling, and number of worker processes for parallel data loading. The DataLoader class is responsible for batching the data and returning it in a format that can be consumed by the model
Sampler
A Sampler is a PyTorch module that provides an indexing strategy for a dataset. It is responsible for determining which samples from the dataset should be included in each batch.
There are two types of samplers available in PyTorch: SequentialSampler and RandomSampler. The SequentialSampler samples data from the dataset in sequential order, while the RandomSampler samples data randomly from the dataset.
In addition to these two samplers, PyTorch also provides a variety of other samplers that can be used for specific use cases, such as the SubsetRandomSampler, which samples data randomly from a subset of the dataset.
Transformation
Transformations are a key component of the PyTorch DataLoader architecture. Transformations are applied to the data as it is loaded to preprocess it for use by the model. PyTorch provides a number of built-in transformations, including resizing, cropping, normalization, and data augmentation.
Transformations can be applied to the data either during loading by the Dataset class or during batching by the DataLoader class. Applying transformations during loading allows for more fine-grained control over the preprocessing of the data, while applying transformations during batching can improve performance by reducing the amount of data that needs to be processed.
Batch
The batch is the basic unit of data used by deep learning models. The batch is typically a tensor of shape (batch_size, input_shape)
, where batch_size
is the number of data points in the batch and input_shape is the shape of the input data.
The DataLoader class is responsible for batching the data from the Dataset object and returning it in a format that can be consumed by the model. The batch size can be configured using the batch_size
argument when creating a DataLoader object.
Parallelism
Parallelism is a key feature of the PyTorch DataLoader architecture. The DataLoader class is designed to handle large datasets efficiently by allowing for parallel data loading and preprocessing.
Parallelism can be achieved in two ways:
Using multiple worker processes to load and preprocess data in parallel
Using a multi-threaded or multi-process data loader to load data in parallel
By default, the DataLoader class uses a single worker process to load and preprocess data. However, it can be configured to use multiple worker processes by setting the num_workers argument when creating a DataLoader object.
Shuffling
Shuffling is the process of randomly reordering the data in a dataset. Shuffling is important for training deep learning models because it prevents the model from overfitting to the order of the data. The PyTorch DataLoader class provides a way to shuffle the data for each epoch.
The DataLoader class can be configured to shuffle the data by setting the shuffle argument to True when creating a DataLoader object. When shuffling is enabled, the DataLoader class randomly shuffles the indices of the data points and returns the data in a random order for each epoch.
Caching
Caching is the process of storing data in memory or on disk to improve performance. Caching can be useful when working with large datasets that take a long time to load and preprocess.
The PyTorch DataLoader class provides a way to cache data using the pin_memory argument. When pin_memory is set to True, the DataLoader class caches the data in pinned memory, which is memory that can be accessed by the GPU. This can improve performance by reducing the time it takes to transfer data from CPU to GPU memory.
Iteration
The PyTorch DataLoader class provides a simple interface for iterating over the data in a dataset. To iterate over the data, simply create a DataLoader object and use a for loop to iterate over the batches:
from torch.utils.data import DataLoader
dataset = MyDataset()
dataloader = DataLoader(dataset, batch_size=32, shuffle=True)
for batch in dataloader:
# do something with the batch
PyTorch DataLoader Example:
In this section, we will explore an example of how to use PyTorch DataLoader to load and preprocess the CIFAR-10 dataset for training a convolutional neural network (CNN) model.
Step 1: Import Libraries
The first step is to import the necessary libraries, including PyTorch, torchvision, and NumPy.
import torch
import torchvision
import [numpy](https://saturncloud.io/glossary/numpy) as np
Step 2: Download and Preprocess Dataset
The next step is to download and preprocess the CIFAR-10 dataset. We will use the torchvision.datasets
module to download the dataset and apply some basic preprocessing, such as normalization.
transform = torchvision.transforms.Compose([
torchvision.transforms.ToTensor(),
torchvision.transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5))
])
train_dataset = torchvision.datasets.CIFAR10(root='./data', train=True,
download=True, transform=transform)
test_dataset = torchvision.datasets.CIFAR10(root='./data', train=False,
download=True, transform=transform)
In the code above, we first define a transform function that converts the images to PyTorch tensors and applies normalization. We then use the torchvision.datasets.CIFAR10
class to download the dataset and apply the transform
function. We create two separate datasets for training and testing.
Step 3: Create DataLoaders
The next step is to create DataLoader
objects for our datasets. We will set the batch size to 64 and enable shuffling for the training data.
train_dataloader = torch.utils.data.DataLoader(train_dataset, batch_size=64,
shuffle=True, num_workers=2)
test_dataloader = torch.utils.data.DataLoader(test_dataset, batch_size=64,
shuffle=False, num_workers=2)
In the code above, we create DataLoader objects for both the training and testing datasets. We set the batch size to 64 and enable shuffling for the training data. We also set the number of worker processes to 2, which will allow us to load data in parallel using multiple CPU processes.
Step 4: Define CNN Model
The next step is to define a simple CNN model for classifying the CIFAR-10 dataset.
class CNNModel(torch.nn.Module):
def __init__(self):
super(CNNModel, self).__init__()
self.conv1 = torch.nn.Conv2d(3, 32, kernel_size=3, stride=1, padding=1)
self.conv2 = torch.nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1)
self.pool = torch.nn.MaxPool2d(kernel_size=2, stride=2)
self.fc1 = torch.nn.Linear(64 * 8 * 8, 128)
self.fc2 = torch.nn.Linear(128, 10)
def forward(self, x):
x = torch.nn.functional.relu(self.conv1(x))
x = self.pool(x)
x = torch.nn.functional.relu(self.conv2(x))
x = self.pool(x)
x = x.view(-1, 64 * 8 * 8)
x = torch.nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
model = CNNModel()
In the code above, we define a simple CNN model with two convolutional layers, two max pooling layers, and two fully connected layers. The forward
function defines the forward pass of the model.
Step 5: Define Loss Function and Optimizer
The next step is to define the loss function and optimizer for training the model.
criterion = torch.nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.001, momentum=0.9)
In the code above, we define the cross-entropy loss function and the stochastic gradient descent (SGD) optimizer with a learning rate of 0.001 and momentum of 0.9.
Step 6: Train and Evaluate Model
The final step is to train and evaluate the model using the created DataLoader
objects. We will train the model for 10 epochs and print the training and testing accuracy for each epoch.
for epoch in range(10):
train_loss = 0.0
train_correct = 0
model.train()
for data, target in train_dataloader:
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
loss.backward()
optimizer.step()
train_loss += loss.item() * data.size(0)
_, pred = torch.max(output, 1)
train_correct += (pred == target).sum().item()
train_loss /= len(train_dataloader.dataset)
train_acc = 100.0 * train_correct / len(train_dataloader.dataset)
test_loss = 0.0
test_correct = 0
model.eval()
with torch.no_grad():
for data, target in test_dataloader:
output = model(data)
loss = criterion(output, target)
test_loss += loss.item() * data.size(0)
_, pred = torch.max(output, 1)
test_correct += (pred == target).sum().item()
test_loss /= len(test_dataloader.dataset)
test_acc = 100.0 * test_correct / len(test_dataloader.dataset)
print(f'Epoch {epoch+1}: Train Loss: {train_loss:.4f}, Train Acc: {train_acc:.2f}%, Test Loss: {test_loss:.4f}, Test Acc: {test_acc:.2f}%')
In the code above, we iterate through the dataset for each epoch, computing the loss and accuracy for both the training and testing datasets. We print the results at the end of each epoch.
The model.train()
and model.eval()
functions set the model to training and evaluation mode, respectively. The optimizer.zero_grad()
function clears the gradients of all optimized tensors to prepare for the next iteration. The loss.backward()
function computes the gradients of the loss with respect to the model parameters. The optimizer.step()
function updates the model parameters based on the computed gradients.
At the end of each epoch, we divide the total loss and correct predictions by the total number of data points in the dataset to get the average loss and accuracy. We then print the results.
Conclusion
The PyTorch DataLoader class is a powerful utility class that is essential for loading and preprocessing data for deep learning models. It provides efficient data loading, data augmentation, flexibility, and shuffling capabilities, making it a popular choice for deep learning practitioners.
In this blog post, we have discussed how to use the PyTorch DataLoader
class to load and preprocess data for deep learning models. We have shown how to create DataLoader
objects for both training and testing datasets, and how to use them to train and evaluate a simple CNN model on the CIFAR-10 dataset.
By using DataLoader
objects, we can easily load and preprocess large datasets in parallel, which can greatly improve the efficiency of training deep learning models.
We hope this blog post has been informative and helpful in understanding the PyTorch DataLoader class. Happy coding!
You may also be interested in:
Combining Dask and PyTorch for Better, Faster Transfer Learning
Measuring Model Training with Weights & Biases on Saturn Cloud
[Speeding up Neural Network Training With Multiple GPUs and Dask](https://saturncloud.io/blog/dask-with-gpus/)
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.