Python Pandas How to Read First n Rows of CSV Files
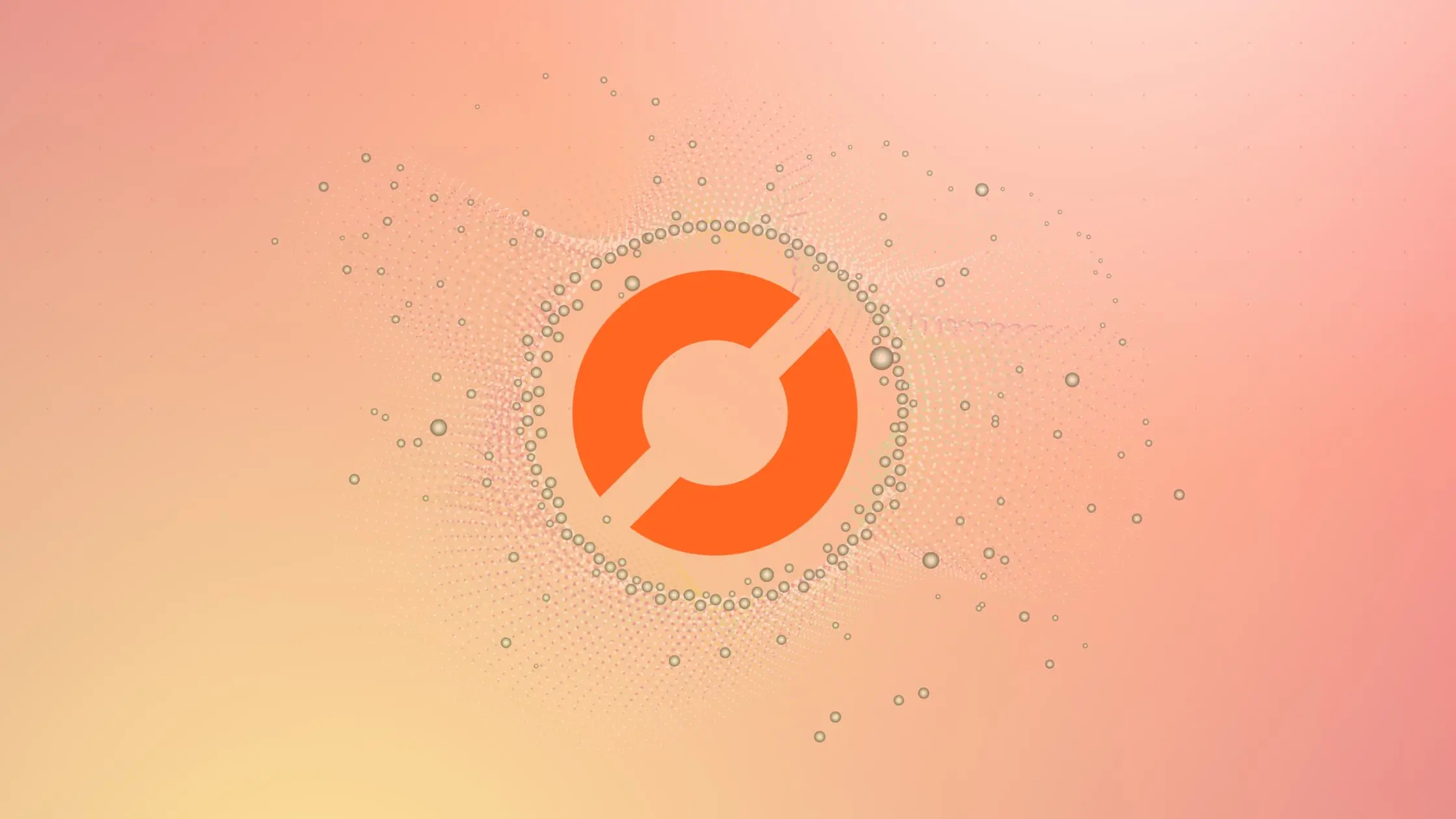
In this article, we will explore how to use Python Pandas to read only the first n rows of a CSV file. We will start by discussing the basics of CSV files and how they are read into Pandas dataframes. Then, we will explain how to use the nrows
parameter to read only the first n rows of a CSV file. Finally, we will provide some examples to demonstrate how this technique can be used in practice.
Understanding CSV Files
CSV stands for “Comma Separated Values”. A CSV file is a plain text file that contains data in a tabular format. Each row in the file represents a record, and each column represents a field within that record. The values in each field are separated by commas (or other delimiters, such as tabs or semicolons).
Here is an example of a simple CSV file:
Name, Age, Gender
Alice, 25, Female
Bob, 30, Male
Charlie, 35, Male
To read this file into a Pandas dataframe, we can use the read_csv()
function:
import pandas as pd
df = pd.read_csv('example.csv')
This will create a dataframe df
that contains all the data from the CSV file.
Reading Only the First n Rows
If we only want to read the first n rows of a CSV file, we can use the nrows
parameter of the read_csv()
function. This parameter specifies the maximum number of rows to read from the file.
Here is an example of how to read only the first two rows of the example.csv
file:
import pandas as pd
df = pd.read_csv('example.csv', nrows=2)
This will create a dataframe df
that contains only the first two rows of the CSV file:
Name Age Gender
0 Alice 25 Female
1 Bob 30 Male
Note that the nrows
parameter does not affect the total number of rows in the CSV file. It only limits the number of rows that are read into the dataframe.
Handling Large CSV Files
Reading only the first n rows of a CSV file can be particularly useful when dealing with large datasets that exceed the available memory of your computer. By limiting the number of rows that are loaded into memory, you can reduce the memory footprint of your program and avoid crashes due to memory errors.
However, it is important to note that reading only the first n rows of a CSV file can also result in loss of information. If the first n rows do not represent a representative sample of the data, you may miss important insights or patterns that are present in the rest of the file.
To mitigate this risk, you can take a random sample of rows from the CSV file instead of reading only the first n rows. This can be done using the sample()
method of the Pandas dataframe:
import pandas as pd
df = pd.read_csv('example.csv').sample(n=1000)
This will create a dataframe df
that contains a random sample of 1000 rows from the CSV file.
Conclusion
In this article, we have explored how to use Python Pandas to read only the first n rows of a CSV file. We have discussed the basics of CSV files and how they are read into Pandas dataframes. We have also explained how to use the nrows
parameter to read only the first n rows of a CSV file and how to handle large CSV files.
By using these techniques, you can efficiently read and manipulate large datasets without running into memory errors or sacrificing important information. As a data scientist or software engineer, this can save you time and help you uncover valuable insights from your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.