Processing .log Files with Pandas: Leveraging Dictionaries and Lists to Create DataFrames
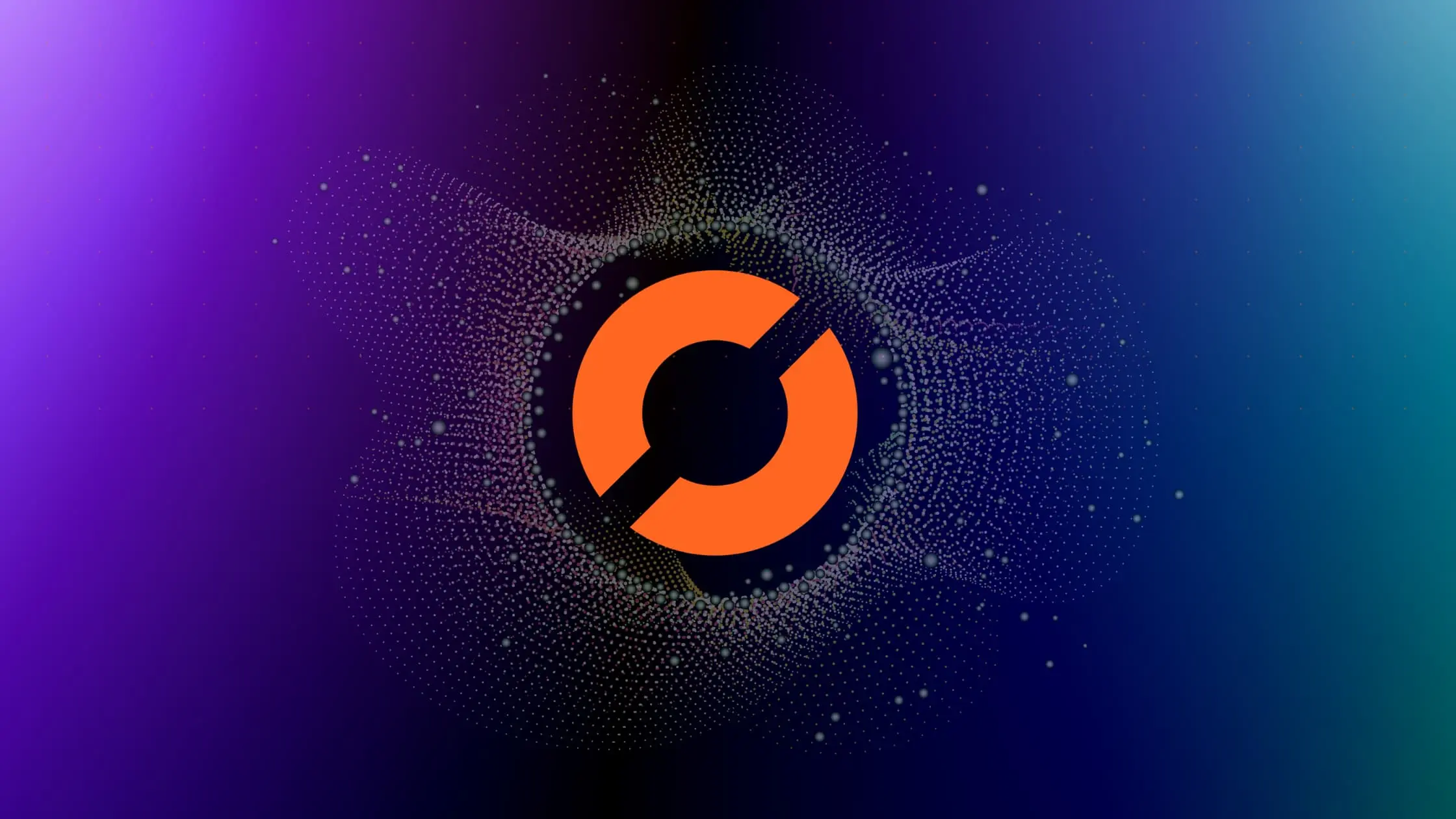
In the realm of data science, we often encounter a variety of data formats. One such format is the .log file, a common file type for storing chronological records of events in a system. Processing these files can be a challenge, but with Python’s Pandas library, we can simplify this task. In this blog post, we’ll explore how to process .log files using Pandas, leveraging dictionaries and lists to create DataFrames.
Table of Contents
Prerequisites
Before we dive in, make sure you have the following:
- Python 3.6 or later
- Pandas library installed
- A .log file for processing
Step-by-Step
Step 1: Reading the .log File
Let’s say we have the following .log file:
{"timestamp": "2023-11-20 12:30:45", "severity": "INFO", "message": "Application started"}
{"timestamp": "2023-11-20 12:35:22", "severity": "ERROR", "message": "Unhandled exception occurred"}
{"timestamp": "2023-11-20 12:40:18", "severity": "DEBUG", "message": "Verbose debugging information"}
{"timestamp": "2023-11-20 12:45:55", "severity": "WARNING", "message": "Resource usage exceeded threshold"}
First, we need to read the .log file. Python’s built-in open()
function is perfect for this task. Here’s how you can do it:
with open('saturn.log', 'r') as file:
log_data = file.readlines()
This code opens the .log file in read mode ('r'
) and reads all lines into the log_data
list.
Step 2: Parsing the .log File
Next, we need to parse the .log file. This step can vary depending on the structure of your .log file. For this tutorial, let’s assume each line in the .log file is a JSON object. We can use Python’s json
module to parse these lines:
import json
parsed_data = [json.loads(line) for line in log_data]
Output:
[{'timestamp': '2023-11-20 12:30:45',
'severity': 'INFO',
'message': 'Application started'},
{'timestamp': '2023-11-20 12:35:22',
'severity': 'ERROR',
'message': 'Unhandled exception occurred'},
{'timestamp': '2023-11-20 12:40:18',
'severity': 'DEBUG',
'message': 'Verbose debugging information'},
{'timestamp': '2023-11-20 12:45:55',
'severity': 'WARNING',
'message': 'Resource usage exceeded threshold'}]
This list comprehension iterates over each line in log_data
, parsing it as a JSON object and storing the result in parsed_data
.
Step 3: Creating a Dictionary
Now, we’ll create a dictionary from the parsed data. This dictionary will serve as the basis for our DataFrame. Each key in the dictionary will correspond to a column in the DataFrame, and the values will be lists containing the data for each row.
data_dict = {}
for data in parsed_data:
for key, value in data.items():
if key not in data_dict:
data_dict[key] = [value]
else:
data_dict[key].append(value)
Output:
{'timestamp': ['2023-11-20 12:30:45',
'2023-11-20 12:35:22',
'2023-11-20 12:40:18',
'2023-11-20 12:45:55'],
'severity': ['INFO', 'ERROR', 'DEBUG', 'WARNING'],
'message': ['Application started',
'Unhandled exception occurred',
'Verbose debugging information',
'Resource usage exceeded threshold']}
This code iterates over each item in parsed_data
, then over each key-value pair in the item. If the key is not already in data_dict
, it adds the key with a new list containing the value. If the key is already in data_dict
, it appends the value to the existing list.
Step 4: Creating a DataFrame
Finally, we can create a DataFrame from data_dict
using Pandas' DataFrame
function:
import pandas as pd
df = pd.DataFrame(data_dict)
print(df)
Output:
timestamp severity message
0 2023-11-20 12:30:45 INFO Application started
1 2023-11-20 12:35:22 ERROR Unhandled exception occurred
2 2023-11-20 12:40:18 DEBUG Verbose debugging information
3 2023-11-20 12:45:55 WARNING Resource usage exceeded threshold
This code creates a new DataFrame df
from data_dict
. Each key-value pair in data_dict
becomes a column in df
, with the key as the column name and the values as the column data.
Common Errors and How to Handle Them
Error 1: Malformed Log Entries
If a log entry is not a valid JSON string, json.loads will raise a json.JSONDecodeError. To handle this, consider using a try-except block:
log_entries_list = []
for entry in log_data:
try:
log_entry_dict = json.loads(entry)
log_entries_list.append(log_entry_dict)
except json.JSONDecodeError:
print(f"Skipping malformed entry: {entry}")
Error 2: Missing Keys in Log Entries
If log entries are missing certain keys, it can lead to inconsistencies when creating the DataFrame. Handle this by ensuring the presence of key-value pairs:
for entry in log_entries_list:
entry.setdefault("missing_key", None)
Conclusion
Processing .log files with Pandas is a straightforward process once you understand the steps. By leveraging Python’s built-in functions and the power of Pandas, we can easily convert .log files into DataFrames for further analysis.
Remember, the parsing step may vary depending on the structure of your .log files. Always inspect your .log files to understand their structure before attempting to parse them.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.