How to Solve CUDA Out of Memory Error in PyTorch
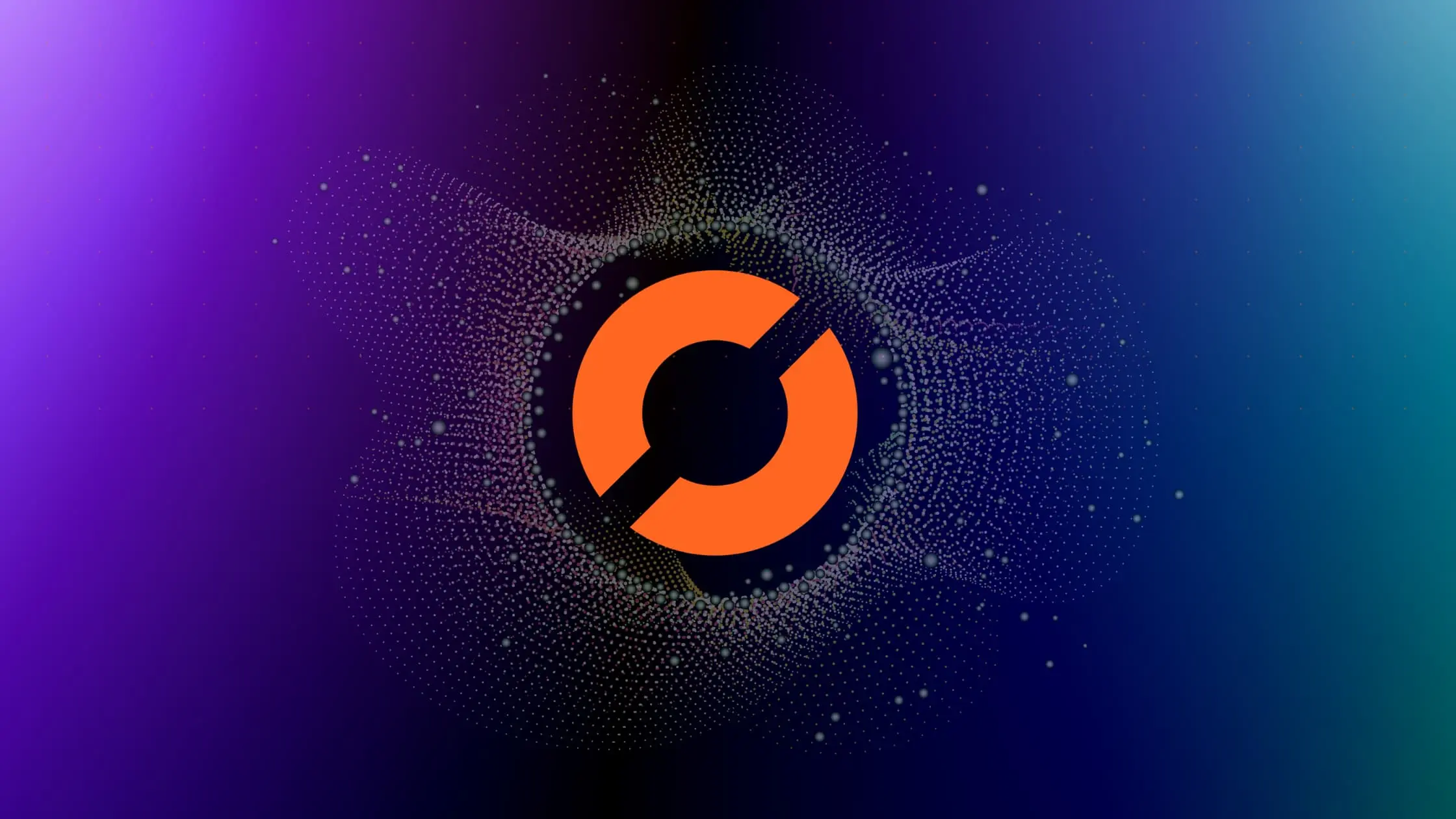
As a software engineer working with data scientists, you may have come across the dreaded 'CUDA out of memory'
error when training your deep learning models. This error occurs when your GPU runs out of memory while trying to allocate memory for your model. In this blog post, we will explore some common causes of this error and how to solve it when using PyTorch.
Table of Contents
- Understanding the Error
- Common Causes of
‘CUDA out of memory’
Error - Solutions to
‘CUDA out of memory’
Error - Conclusion
Understanding the Error
Before we dive into the solutions, let’s take a moment to understand the error message itself. When you run your PyTorch code and encounter the 'CUDA out of memory'
error, you will see a message that looks something like this:
RuntimeError: CUDA out of memory. Tried to allocate xxx MiB (GPU X; Y MiB total capacity; Z MiB already allocated; A MiB free; B MiB cached)
This error message provides some useful information that can help us diagnose the problem. Let’s break it down:
xxx MiB
: This is the amount of memory that PyTorch tried to allocate but failed to do so because the GPU ran out of memory.GPU X
: This tells us which GPU the error occurred on, in case we have multiple GPUs.Y MiB total capacity
: This tells us the total capacity of the GPU in terms of memory.Z MiB already allocated
: This tells us how much memory is already in use by PyTorch.A MiB free
: This tells us how much memory is available for use by PyTorch.B MiB cached
: This tells us how much memory is being used by the caching mechanism in PyTorch.
Common Causes of 'CUDA out of memory'
Error
Now that we have a better understanding of the error message, let’s explore some common causes of this error.
1. Model size is too large
One of the most common causes of the 'CUDA out of memory'
error is that your model is too large for the available GPU memory. If you have a large model with many layers and parameters, it may not fit into the memory of your GPU.
2. Batch size is too large
Another common cause of the 'CUDA out of memory'
error is that your batch size is too large. When training your model, you typically feed it data in batches. If your batch size is too large, it can quickly consume all the available GPU memory.
3. Data augmentation is too intensive
Data augmentation is a technique used to generate additional training data by applying transformations to your existing data. While data augmentation can be a powerful tool for improving the performance of your model, it can also be memory-intensive. If you are applying too many data augmentation techniques, it can cause the 'CUDA out of memory'
error.
4. Inefficient memory usage
Finally, inefficient memory usage can also cause the 'CUDA out of memory'
error. This can happen if you are not properly releasing memory when it is no longer needed, or if you are not using PyTorch’s caching mechanism effectively.
Solutions to 'CUDA out of memory'
Error
Now that we have a better understanding of the common causes of the 'CUDA out of memory'
error, let’s explore some solutions.
1. Reduce model size
If your model is too large for the available GPU memory, one solution is to reduce its size. This can be done by reducing the number of layers or parameters in your model. Alternatively, you can use a smaller pre-trained model as a starting point and fine-tune it for your specific task.
2. Reduce batch size
If your batch size is too large, you can reduce it to free up some GPU memory. However, keep in mind that a smaller batch size can also lead to slower convergence during training.
# Before
batch_size = 64
# After
batch_size = 32
3. Reduce data augmentation
If you are using too many data augmentation techniques, you can try reducing the number of transformations or using less memory-intensive techniques.
4. Optimize memory usage
To optimize memory usage, you can use PyTorch’s caching mechanism to store intermediate results instead of recomputing them every time. You can also release memory when it is no longer needed by calling torch.cuda.empty_cache()
.
import torch
# Before
some_tensor = torch.randn(1000, 1000).cuda()
# Perform operations
del some_tensor
# After
torch.cuda.empty_cache()
5. Use mixed precision training
Mixed precision training is a technique that uses lower-precision data types for some parts of the computation to reduce memory usage and speed up training. PyTorch provides support for mixed precision training through the torch.cuda.amp
module.
from torch.cuda.amp import autocast, GradScaler
scaler = GradScaler()
# Before
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
loss.backward()
optimizer.step()
# After
with autocast():
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
scaler.scale(loss).backward()
scaler.step(optimizer)
scaler.update()
6. Gradient Accumulation
Gradient accumulation involves accumulating gradients over multiple smaller batches before performing the weight update. This allows the model to process larger effective batch sizes without increasing memory requirements.
# Before
for data in dataloader:
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
loss.backward()
optimizer.step()
# After
accumulation_steps = 4
for i, data in enumerate(dataloader, 1):
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
loss.backward()
if i % accumulation_steps == 0:
optimizer.step()
Conclusion
The 'CUDA out of memory'
error can be frustrating to deal with, but by understanding its common causes and implementing the solutions we have discussed, you can overcome it and train your deep learning models successfully. Remember to keep an eye on your model size, batch size, and data augmentation, and optimize your memory usage to make the most of your available GPU memory. Happy training!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.