How to Remove Space from Columns in Pandas A Data Scientists Guide
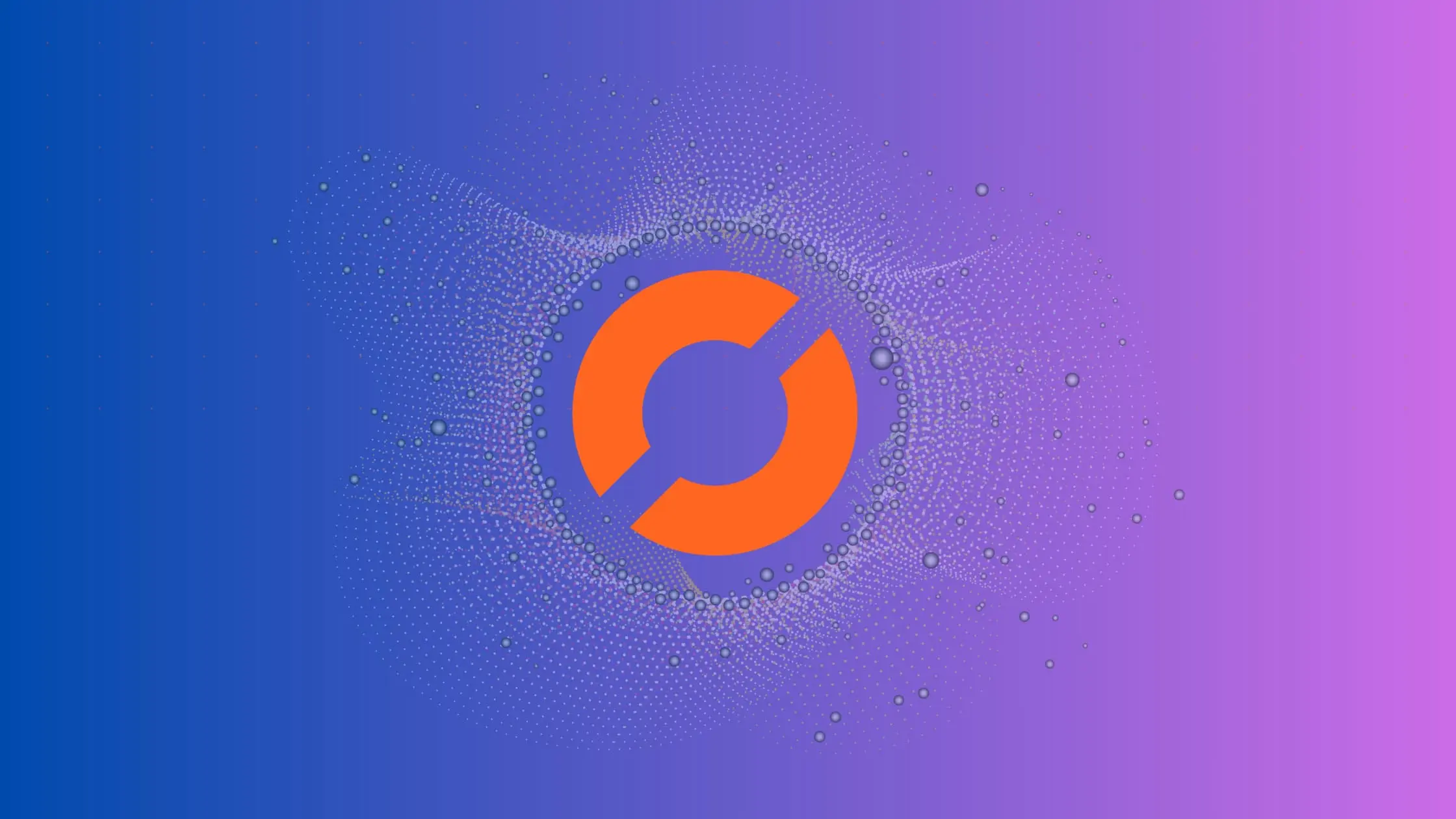
As a data scientist, one of the most common tasks you’ll have to deal with is cleaning and manipulating data. One of the problems you may encounter is dealing with spaces in columns, which can cause errors and inconsistencies in your analysis. In this article, we’ll explore how to remove spaces from columns in pandas, a popular data manipulation library in Python.
What is Pandas?
Pandas is a powerful data manipulation library for Python that provides fast and flexible data structures for working with structured data. It allows you to load, manipulate, and analyze data in a variety of formats, including CSV, Excel, SQL databases, and more.
Why Remove Spaces from Columns?
Spaces in column names can cause problems when working with data. For example, if you have a column name with a space in it, you’ll need to use quotes or brackets when referencing it in code. This can be cumbersome and error-prone, especially if you’re working with many columns.
In addition, spaces in column values can cause problems when performing calculations or comparisons. For example, if you’re trying to sum up values in a column, but some of the values have spaces before or after them, you may get unexpected results.
How to Remove Spaces from Columns in Pandas
Let’s say we have the following DataFrame:
import pandas as pd
data = {
'Name ': ['John Smith', 'Alice Brown', 'Mike Green ', ' Sarah White '],
' Age': [25, 30, 28, 32]
}
df = pd.DataFrame(data)
print(df)
Output:
Name Age
0 John Smith 25
1 Alice Brown 30
2 Mike Green 28
3 Sarah White 32
This DataFrame contains spaces in both column names and column values. Let’s explore how to remove both of them. Removing spaces from columns in pandas is a straightforward process. There are different methods you can use depending on your needs. Here are three common approaches:
1. Using the str.strip()
method
The str.strip()
method removes leading and trailing whitespace from strings in a pandas series or dataframe. You can use this method to remove spaces from column names or column values.
To remove spaces from column names, you can use the rename()
method with a lambda function that applies the str.strip()
method to each column name:
# remove space in column names using strip() function
df.rename(columns=lambda x: x.strip(), inplace=True)
print(df)
Output:
Name Age
0 John Smith 25
1 Alice Brown 30
2 Mike Green 28
3 Sarah White 32
To remove spaces from column values, you can apply the str.strip()
method to the column using the apply()
method:
# remove space in column values using strip() function
df['column_name'] = df['column_name'].apply(lambda x: x.strip())
print(df)
Output:
Name Age
0 John Smith 25
1 Alice Brown 30
2 Mike Green 28
3 Sarah White 32
2. Using the str.replace()
method
The str.replace()
method replaces all occurrences of a substring with another substring in a pandas series or dataframe. You can use this method to replace spaces with an empty string.
To remove spaces from column names, you can use the rename()
method with a dictionary that maps the old column names to the new column names:
# remove space in column names using replace() function
df.rename(columns={'Name ': 'Name'}, inplace=True)
df.rename(columns={' Age': 'Age'}, inplace=True)
print(df)
Output:
Name Age
0 John Smith 25
1 Alice Brown 30
2 Mike Green 28
3 Sarah White 32
To remove spaces from column values, you can apply the str.replace()
method to the column using the apply()
method:
# remove space in column values using replace() function
df['Name'] = df['Name'].apply(lambda x: x.replace(' ', ''))
print(df)
Output:
Name Age
0 JohnSmith 25
1 AliceBrown 30
2 MikeGreen 28
3 SarahWhite 32
3. Using the columns.str.replace()
method
The columns.str.replace()
method is similar to the str.replace()
method, but it applies the replacement to all column names in a pandas dataframe at once.
# remove space in column values using columns.str.replace() function
df.columns = df.columns.str.replace(' ', '')
print(df)
Output:
Name Age
0 John Smith 25
1 Alice Brown 30
2 Mike Green 28
3 Sarah White 32
Conclusion
Removing spaces from columns in pandas is a common task that can be accomplished using a variety of methods. The str.strip()
, str.replace()
, and columns.str.replace()
methods are three common approaches you can use depending on your needs.
Cleaning and manipulating data can be a time-consuming task, but it’s essential for accurate analysis and insights. By using pandas and the techniques described in this article, you can streamline your data cleaning process and focus on the more interesting and valuable parts of your data science work.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.