How to Merge Multiple Column Values into One Column in Python Pandas
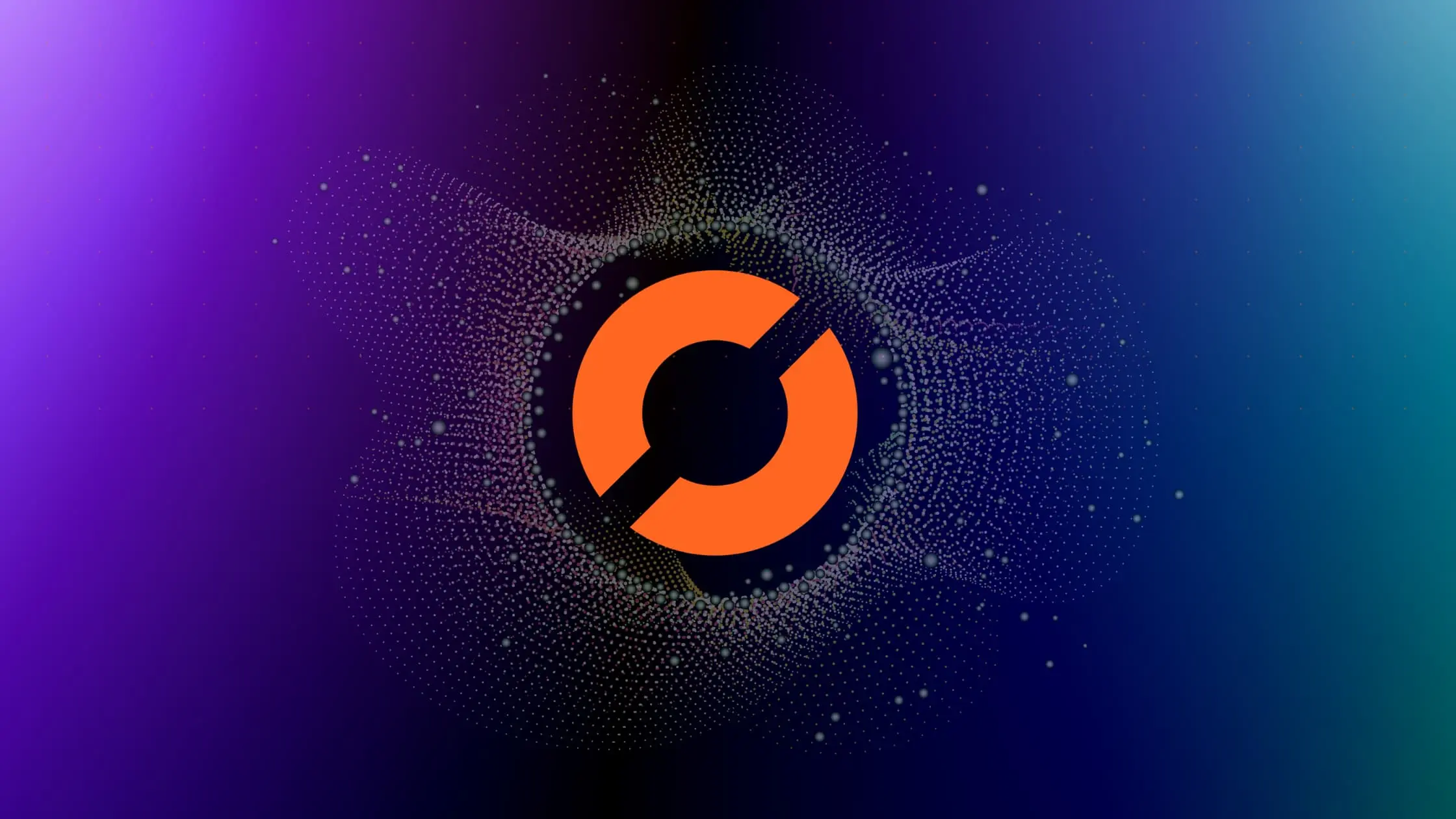
If you are a data scientist or software engineer working with data sets, there may be times when you need to merge the values from multiple columns into one column. This can be useful for various reasons, such as simplifying your data set, creating a new column for analysis, or preparing your data for a machine learning model. In this article, we will explore how to merge multiple column values into one column in Python using the Pandas library.
Table of Contents
- Introduction
- What is Pandas?
- How to Merge Multiple Column Values into One Column
- Common Errors and Solutions
- Best Practices
- Conclusion
What is Pandas?
Pandas is a popular open-source library for data manipulation and analysis in Python. It provides powerful data structures such as DataFrames and Series that allow you to work with structured data in a simple and intuitive way. Pandas is widely used in data science and machine learning projects and can handle various data formats such as CSV, Excel, SQL, and more.
How to Merge Multiple Column Values into One Column
Method 2: Using the lambda
Operator
The objs
parameter is a sequence of pandas objects such as data frames or series that we want to concatenate. The axis
parameter specifies the axis along which we want to concatenate the objects. In our case, we will set axis=1
to concatenate columns. The join
parameter specifies how to handle the intersection of the objects. We will set join='outer'
to include all columns in all data frames. Finally, we will set the ignore_index
parameter to True
to reset the index of the resulting data frame.
Here is an example code snippet that demonstrates how to merge multiple columns into one column:
import pandas as pd
#create a sample data frame
data = {
'Name': ['John', 'Mary', 'Peter'],
'Age': [25, 30, 35],
'Gender': ['Male', 'Female', 'Male']
}
df = pd.DataFrame(data)
#merge multiple columns into one column
merged_column = df.apply(lambda row: ' '.join(map(str, row)), axis=1)
#create a new DataFrame with the merged column
result_df = pd.DataFrame({'Merged_Data': merged_column})
#print the resulting data frame
print(result_df)
Output
Merged_Data
0 John 25 Male
1 Mary 30 Female
2 Peter 35 Male
Method 2: Using the +
Operator
# Method 2: Using the + Operator
df['Merged_Data'] = df['Name'] + ' ' + df['Age'].astype(str) + ' ' + df['Gender']
print(df[['Merged_Data']])
Output
Merged_Data
0 John 25 Male
1 Mary 30 Female
2 Peter 35 Male
Method 3: Using the apply
Function with a Custom Function
# Method 3: Using apply with a custom function
def merge_columns(row):
return f"{row['Name']} {row['Age']} {row['Gender']}"
df['Merged_Data'] = df.apply(merge_columns, axis=1)
print(df[['Merged_Data']])
Output
Merged_Data
0 John 25 Male
1 Mary 30 Female
2 Peter 35 Male
Common Errors and Solutions
Error 1: TypeError - Cannot Concatenate Object of Type 'int'
Code to Generate Error:
# Generate Error
df['Merged_Data'] = df['Name'] + df['Age'] + df['Gender']
Solution:
# Solution
df['Merged_Data'] = df['Name'].astype(str) + df['Age'].astype(str) + df['Gender']
Error 2: KeyError - Column Not Found
Code to Generate Error:
# Generate Error
df['Merged_Data'] = df['FullName']
Solution:
# Solution
df['Merged_Data'] = df['Name'] + ' ' + df['Age'].astype(str) + ' ' + df['Gender']
Best Practices
Data Type Consistency: Ensure that the data types of the columns you are merging are consistent. Convert them to the appropriate data type before merging.
Handling Missing Values: Account for missing values in columns. Use functions like
fillna
or handle them based on your analysis requirements.Avoiding Redundant Columns: After merging, consider dropping the original columns if they are no longer needed to keep the DataFrame clean and concise.
Custom Functions: Utilize custom functions with the
apply
method when you need more complex logic for merging.Performance Considerations: For large datasets, evaluate the performance of different methods. The
+
operator can be faster, but it may not be as flexible as theapply
method for complex operations.
Conclusion
In conclusion, merging multiple column values into a single column is a common task for data scientists and software engineers working with data sets. Python’s Pandas library provides several methods to achieve this, such as using the lambda operator, the + operator, or applying custom functions with the apply method. The choice of method depends on the specific requirements of the task and the complexity of the data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.