How to Efficiently Read Large CSV Files in Python Pandas
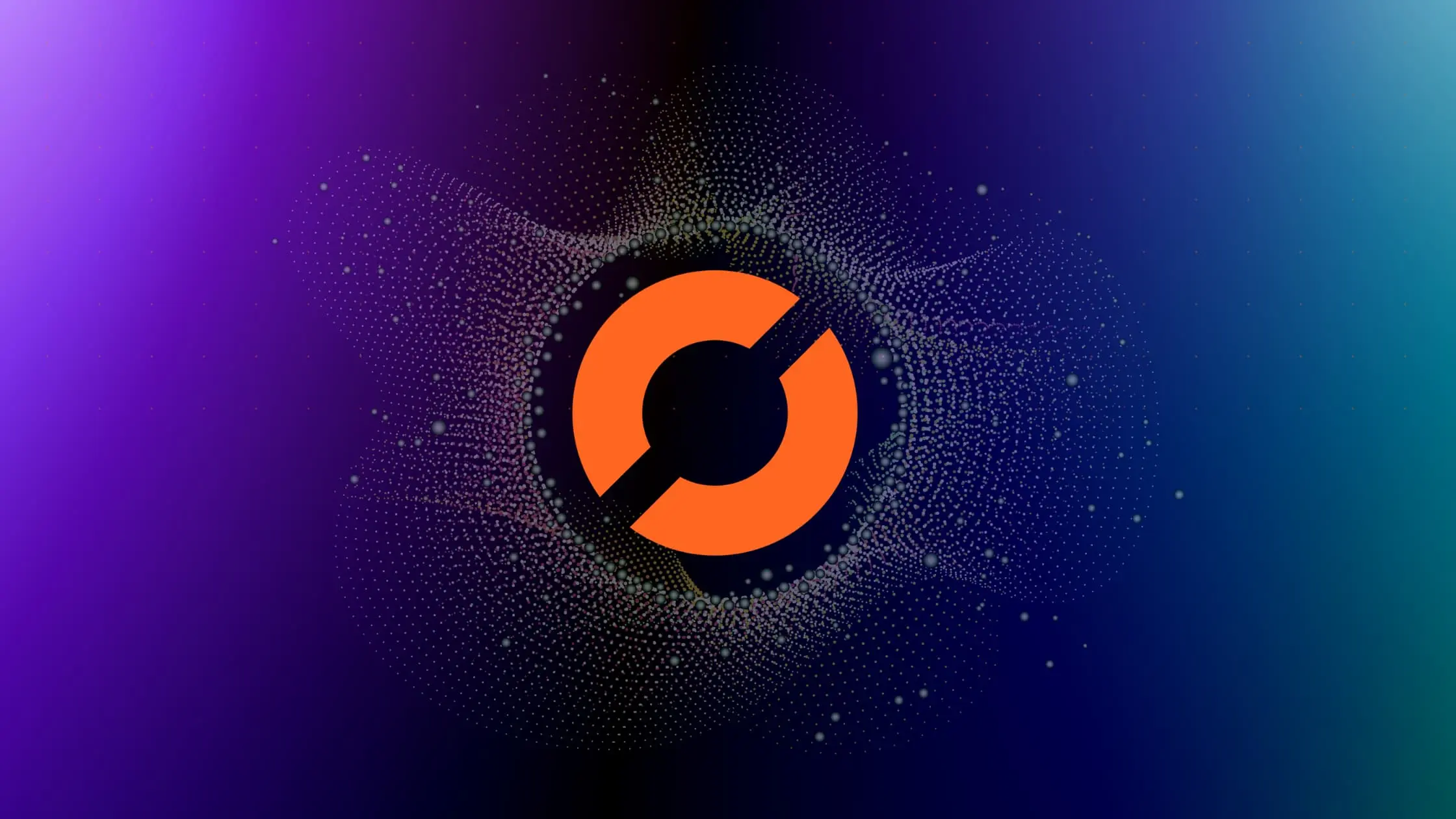
As a data scientist or software engineer, you are likely familiar with the Python Pandas library. Pandas is an essential tool for data analysis and manipulation, providing a fast and flexible way to work with structured data. However, when dealing with large datasets, you may encounter memory issues when trying to load data into Pandas data frames. In this article, we will discuss how to efficiently read large CSV files in Python Pandas without causing memory crashes.
Table of Contents
- Understanding the Problem
- Solutions
- Pros and Cons of Each Method
- Common Errors and How to Handle Them
- Conclusion
Understanding the Problem
When working with large datasets, it’s common to use CSV files for storing and exchanging data. CSV files are easy to use and can be easily opened in any text editor. However, when you try to load a large CSV file into a Pandas data frame using the read_csv
function, you may encounter memory crashes or out-of-memory errors. This is because Pandas loads the entire CSV file into memory, which can quickly consume all available RAM.
Solutions
1. Use Chunking
One way to avoid memory crashes when loading large CSV files is to use chunking. Chunking involves reading the CSV file in small chunks and processing each chunk separately. This approach can help reduce memory usage by loading only a small portion of the CSV file into memory at a time.
To use chunking, you can set the chunksize
parameter in the read_csv
function. This parameter determines the number of rows to read at a time. For example, to read a CSV file in chunks of 1000 rows, you can use the following code:
import pandas as pd
chunksize = 1000
for chunk in pd.read_csv('large_file.csv', chunksize=chunksize):
# process each chunk here
In this example, the read_csv
function will return an iterator that yields data frames of 1000 rows each. You can then process each chunk separately within the for loop.
2. Use Dask
Another solution to the memory issue when reading large CSV files is to use Dask. Dask is a distributed computing library that provides parallel processing capabilities for data analysis. Dask can handle data sets that are larger than the available memory by partitioning the data and processing it in parallel across multiple processors or machines.
Dask provides a read_csv
function that is similar to Pandas read_csv
. The main difference is that Dask returns a Dask data frame, which is a collection of smaller Pandas data frames. To use Dask, you can install it using pip:
pip install dask[complete]
Then, you can use the read_csv
function to load the CSV file as follows:
import dask.dataframe as dd
df = dd.read_csv('large_file.csv')
In this example, the read_csv
function returns a Dask data frame that represents the CSV file. You can then perform various operations on the Dask data frame, such as filtering, aggregating, and joining.
One advantage of using Dask is that it can handle much larger datasets than Pandas. Dask can process data sets that are larger than the available memory by using disk storage and partitioning the data across multiple processors or machines.
3. Use Compression
Another way to reduce memory usage when loading large CSV files is to use compression. Compression can significantly reduce the size of the CSV file, which can help reduce the amount of memory required to load it into a Pandas data frame.
To use compression, you can compress the CSV file using a compression algorithm, such as gzip or bzip2. Then, you can use the read_csv
function with the compression
parameter to read the compressed file. For example, to read a CSV file that has been compressed using gzip, you can use the following code:
import pandas as pd
df = pd.read_csv('large_file.csv.gz', compression='gzip')
In this example, the read_csv
function will read the compressed CSV file and decompress it on the fly. This approach can help reduce the amount of memory required to load the CSV file into a Pandas data frame.
Pros and Cons of Each Method
Method | Pros | Cons |
---|---|---|
Chunks | Memory-efficient, easy to implement | Slower compared to reading entire file |
Dask | Parallel processing, handles large data | Additional dependency, learning curve |
Compression | Saves storage space | May increase reading time |
Common Errors and How to Handle Them
MemoryError
If you encounter a MemoryError
while reading large files, consider using chunks or Dask to process data in smaller portions.
ParserError
A ParserError
may occur due to malformed data. Check for inconsistent delimiters or use the error_bad_lines
parameter to skip problematic lines.
Conclusion
In conclusion, reading large CSV files in Python Pandas can be challenging due to memory issues. However, there are several solutions available, such as chunking, using Dask, and compression. By using these solutions, you can efficiently read large CSV files in Python Pandas without causing memory crashes.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.