How to Convert Pandas Series to DateTime in a DataFrame
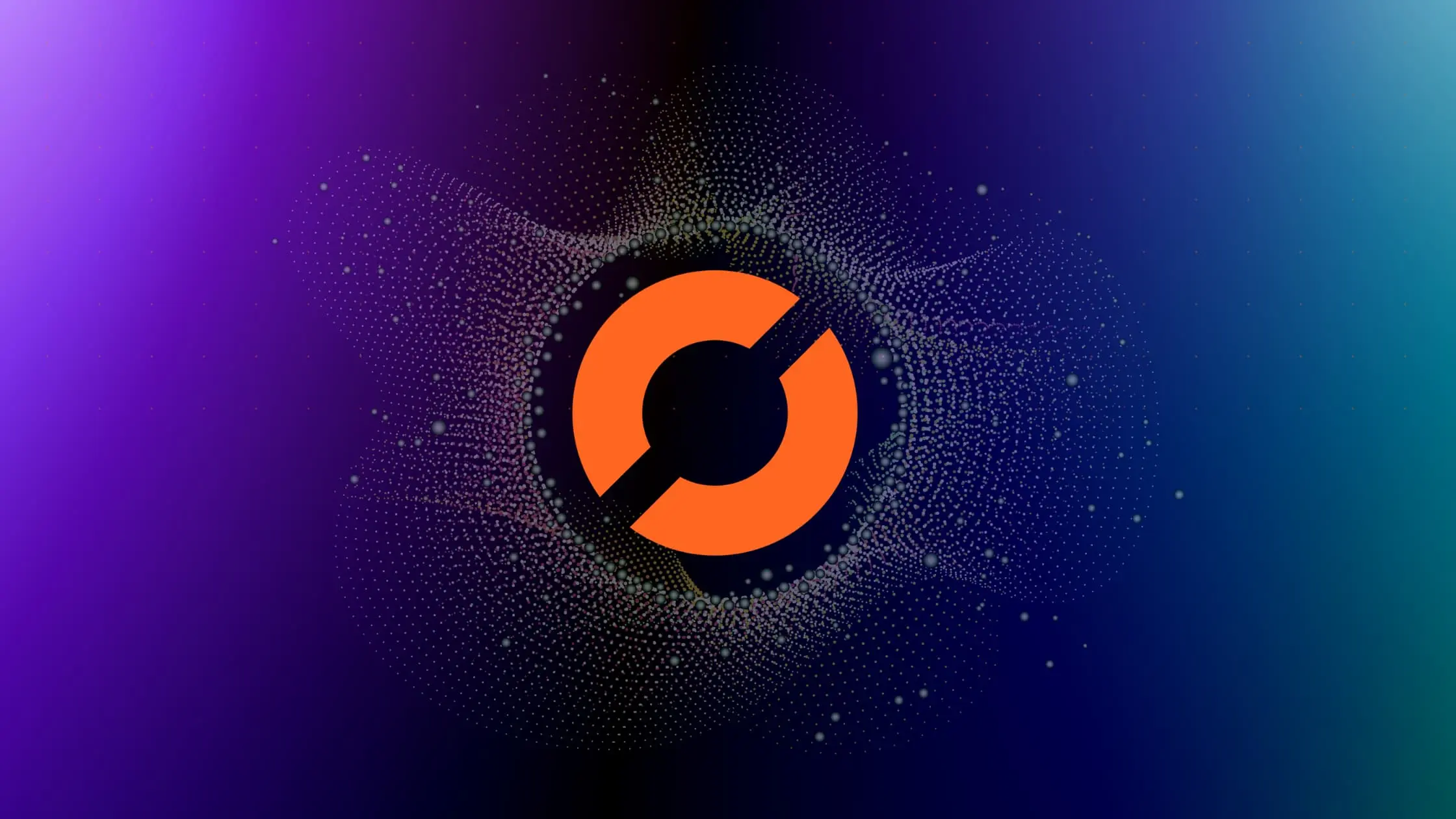
As a data scientist or software engineer, you may often come across the need to convert a Pandas Series to DateTime in a DataFrame. This is a common task when working with time-series data, which is prevalent in many applications, including finance, healthcare, and IoT.
In this article, we will walk you through the steps to convert a Pandas Series to DateTime in a DataFrame. We will start by explaining what Pandas Series and DateTime are and why you might need to convert them. Then, we will show you the different methods to convert a Pandas Series to DateTime in a DataFrame.
Table of Contents
- What are Pandas Series and DateTime?
- Why Convert Pandas Series to DateTime in a DataFrame?
- How to Convert Pandas Series to DateTime in a DataFrame
- Conclusion
What are Pandas Series and DateTime?
Before we dive into the details of converting Pandas Series to DateTime in a DataFrame, let’s first define what Pandas Series and DateTime are.
Pandas Series: A Pandas Series is a one-dimensional labeled array that can hold any data type, including integers, floats, strings, and objects. It is similar to a column in a spreadsheet or a database table and can be used to represent a single variable or feature.
DateTime: DateTime is a Python module that provides classes for working with dates and times. It allows you to perform various operations on dates and times, such as parsing, formatting, arithmetic, and comparison.
Why Convert Pandas Series to DateTime in a DataFrame?
There are several reasons why you might need to convert a Pandas Series to DateTime in a DataFrame. Some of the common use cases are:
- Working with time-series data: Time-series data is a sequence of data points collected at regular intervals over time. To analyze and visualize time-series data, you need to convert it to DateTime format.
- Merging data from different sources: When merging data from different sources, you might encounter DateTime values in different formats. To merge the data correctly, you need to convert the DateTime values to a consistent format.
- Filtering and sorting data: When filtering or sorting data based on DateTime values, you need to convert them to a DateTime format to perform the operations correctly.
How to Convert Pandas Series to DateTime in a DataFrame
Now that we have a clear understanding of what Pandas Series and DateTime are and why we might need to convert them, let’s look at the different methods to convert a Pandas Series to DateTime in a DataFrame.
Method 1: Using the pd.to_datetime() Method
The easiest and most straightforward way to convert a Pandas Series to DateTime in a DataFrame is to use the pd.to_datetime()
method. This method converts a Pandas Series to a DateTime object and returns a new Series with the DateTime values.
Here’s an example:
import pandas as pd
# create a DataFrame with a Pandas Series
df = pd.DataFrame({'date': ['2021-05-01', '2021-05-02', '2021-05-03'], 'value': [10, 20, 30]})
# convert the 'date' column to DateTime format
df['date'] = pd.to_datetime(df['date'])
print(df)
Output:
date value
0 2021-05-01 10
1 2021-05-02 20
2 2021-05-03 30
As you can see, we first created a DataFrame with a Pandas Series called date
that contains string values representing dates. We then used the pd.to_datetime()
method to convert the date
column to DateTime format.
Method 2: Using the pd.to_datetime() Method with Custom Format
Sometimes, the DateTime values in your Pandas Series might not be in a format that the pd.to_datetime()
method can recognize. In such cases, you can use the format
parameter to specify a custom format for the DateTime values.
Here’s an example:
import pandas as pd
# create a DataFrame with a Pandas Series
df = pd.DataFrame({'date': ['01-05-2021', '02-05-2021', '03-05-2021'], 'value': [10, 20, 30]})
# convert the 'date' column to DateTime format with custom format
df['date'] = pd.to_datetime(df['date'], format='%d-%m-%Y')
print(df)
Output:
date value
0 2021-05-01 10
1 2021-05-02 20
2 2021-05-03 30
As you can see, we used the format
parameter to specify a custom format for the DateTime values in the ‘date’ column.
Method 3: Using the pd.to_datetime() Method with Multiple Columns
In some cases, you might need to convert multiple columns in a DataFrame to DateTime format. In such cases, you can use the pd.to_datetime()
method with multiple columns.
Here’s an example:
import pandas as pd
# create a DataFrame with multiple Pandas Series
df = pd.DataFrame({
'year': [2021, 2021, 2021],
'month': [5, 5, 5],
'day': [1, 2, 3],
'value': [10, 20, 30]
})
# convert the 'year', 'month', and 'day' columns to DateTime format
df['date'] = pd.to_datetime(df[['year', 'month', 'day']])
print(df)
Output:
year month day value date
0 2021 5 1 10 2021-05-01
1 2021 5 2 20 2021-05-02
2 2021 5 3 30 2021-05-03
As you can see, we first created a DataFrame with multiple Pandas Series representing the year, month, day, and value. We then used the pd.to_datetime()
method with the year
, month
, and day
columns to create a new column called ‘date’ containing DateTime values.
Conclusion
Converting a Pandas Series to DateTime in a DataFrame is a common task when working with time-series data or merging and filtering data based on DateTime values. In this article, we showed you the different methods to convert a Pandas Series to DateTime in a DataFrame, including using the pd.to_datetime()
method and specifying custom formats and multiple columns. We hope this article has been helpful in your data science and software engineering projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.