How to Check if PyTorch is Using the GPU
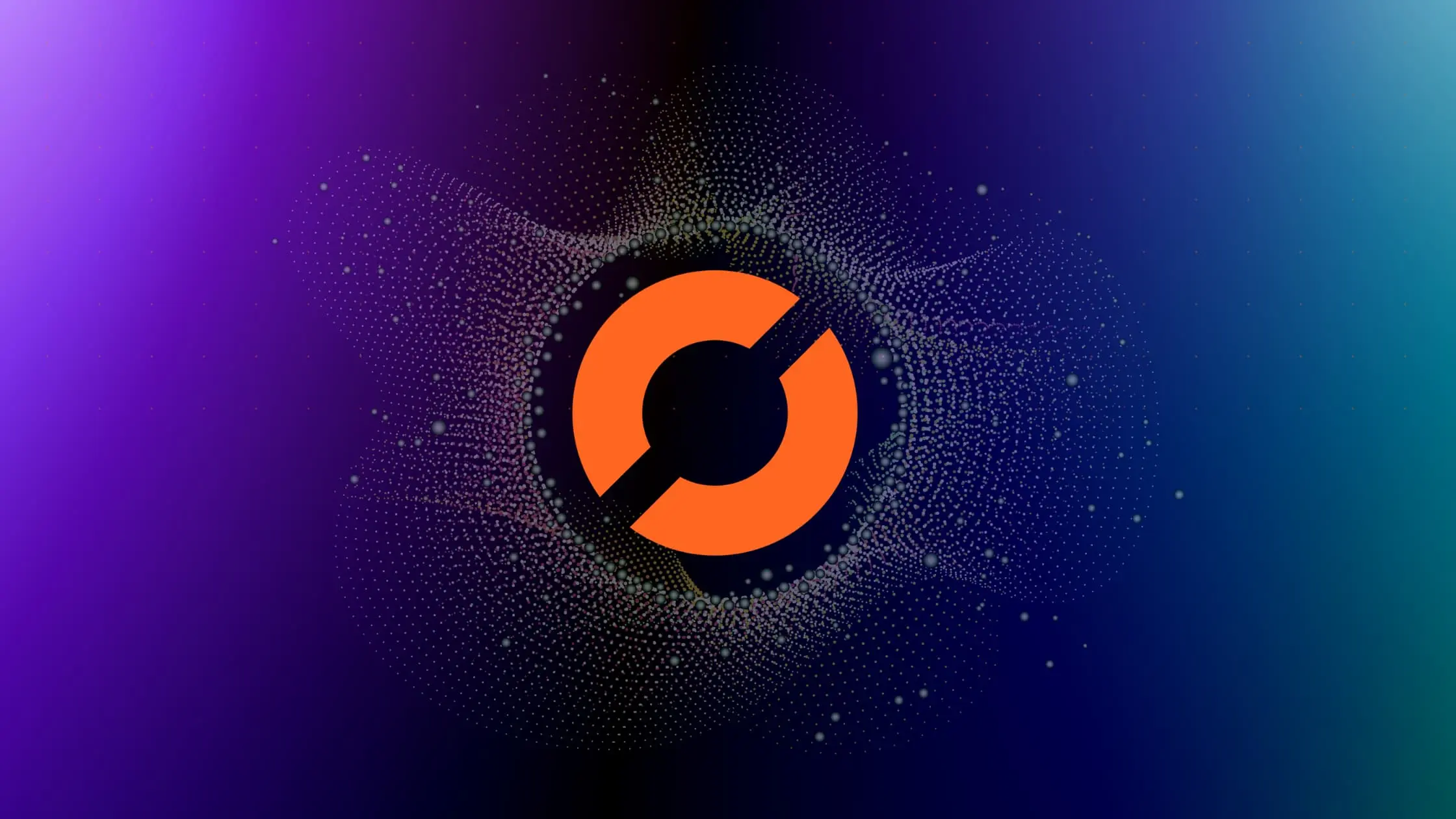
If you’re a data scientist or software engineer using PyTorch for deep learning projects, you’ve probably wondered whether your code is utilizing the GPU or not. GPUs can significantly speed up training and inference times for deep learning models, so it’s important to ensure that your code is utilizing them to their fullest extent. In this article, we’ll explore how to check if PyTorch is using the GPU.
Table of Contents
- What is PyTorch?
- Checking if PyTorch is Using the GPU
- Using PyTorch with the GPU
- Common Errors and How to Handle Them
- Conclusion
What is PyTorch?
Before we dive into the details of how to check if PyTorch is using the GPU, let’s briefly discuss what PyTorch is. PyTorch is an open-source machine learning library for Python. It was developed primarily by Facebook’s AI research team and is used extensively for deep learning projects. PyTorch is known for its dynamic computational graph, which allows for easy debugging and efficient memory usage. It also has excellent support for GPUs, which is important for training large deep learning models.
Checking if PyTorch is Using the GPU
So, how do you check if PyTorch is using the GPU? The first step is to ensure that you have PyTorch installed with GPU support. You can do this by running the following command:
pip install torch torchvision torchaudio -f https://download.pytorch.org/whl/cu111/torch_stable.html
Note that the cu111
in the URL specifies that we want to install the version of PyTorch that is compatible with CUDA 11.1, which is the latest version at the time of writing. If you have a different version of CUDA installed, you should adjust the URL accordingly.
Once you have PyTorch installed with GPU support, you can check if it’s using the GPU by running the following code:
import torch
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
print(f"Using device: {device}")
This code first checks if a GPU is available by calling the torch.cuda.is_available()
function. If a GPU is available, it sets the device
variable to "cuda"
, indicating that we want to use the GPU. If a GPU is not available, it sets device
to "cpu"
, indicating that we want to use the CPU.
The code then prints out which device is being used. If you see "cuda"
, then PyTorch is using the GPU. If you see "cpu"
, then PyTorch is using the CPU.
Using PyTorch with the GPU
Now that you know how to check if PyTorch is using the GPU, let’s discuss how to use PyTorch with the GPU.
When using PyTorch with the GPU, you need to ensure that your tensors are on the GPU. You can do this by calling the to()
method on your tensors and passing in the device
variable that we created earlier. For example:
import torch
# Create a tensor on the CPU
x = torch.randn(3, 3)
# Move the tensor to the GPU
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
x = x.to(device)
In this code, we first create a tensor x
on the CPU. We then move the tensor to the GPU by calling the to()
method and passing in the device
variable.
You should also ensure that your model is on the GPU when training or making predictions. You can do this by calling the to()
method on your model and passing in the device
variable. For example:
import torch.nn as nn
# Define a simple neural network
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(10, 10)
self.fc2 = nn.Linear(10, 1)
def forward(self, x):
x = self.fc1(x)
x = self.fc2(x)
return x
# Create an instance of the network
net = Net()
# Move the network to the GPU
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
net = net.to(device)
In this code, we define a simple neural network Net
that consists of two fully connected layers. We then create an instance of the network net
and move it to the GPU by calling the to()
method and passing in the device
variable.
Common Errors and How to Handle Them
- Error 1: CUDA Error Error Message:
RuntimeError: CUDA error: device-side assert triggered
Solution: Ensure your PyTorch version is compatible with your CUDA version. Check the PyTorch documentation for the recommended CUDA version.
- Error 2: Incorrect CUDA Version Error Message:
RuntimeError: Detected that PyTorch and CUDA versions do not match
Solution: Update PyTorch and CUDA to versions that are compatible with each other.
- Error 3: GPU Memory Issues Error Message:
RuntimeError: CUDA out of memory
Solution: Free up GPU memory by reducing batch sizes or unloading unnecessary tensors. Consider using mixed-precision training to reduce memory usage.
Conclusion
In this article, we’ve explored how to check if PyTorch is using the GPU and how to use PyTorch with the GPU. GPUs can significantly speed up deep learning projects, so it’s important to ensure that your code is utilizing them to their fullest extent. By following the steps outlined in this article, you can ensure that PyTorch is using the GPU and take advantage of its performance benefits.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.