Why You Need to Compile Your Keras Model Before Using model.evaluate()
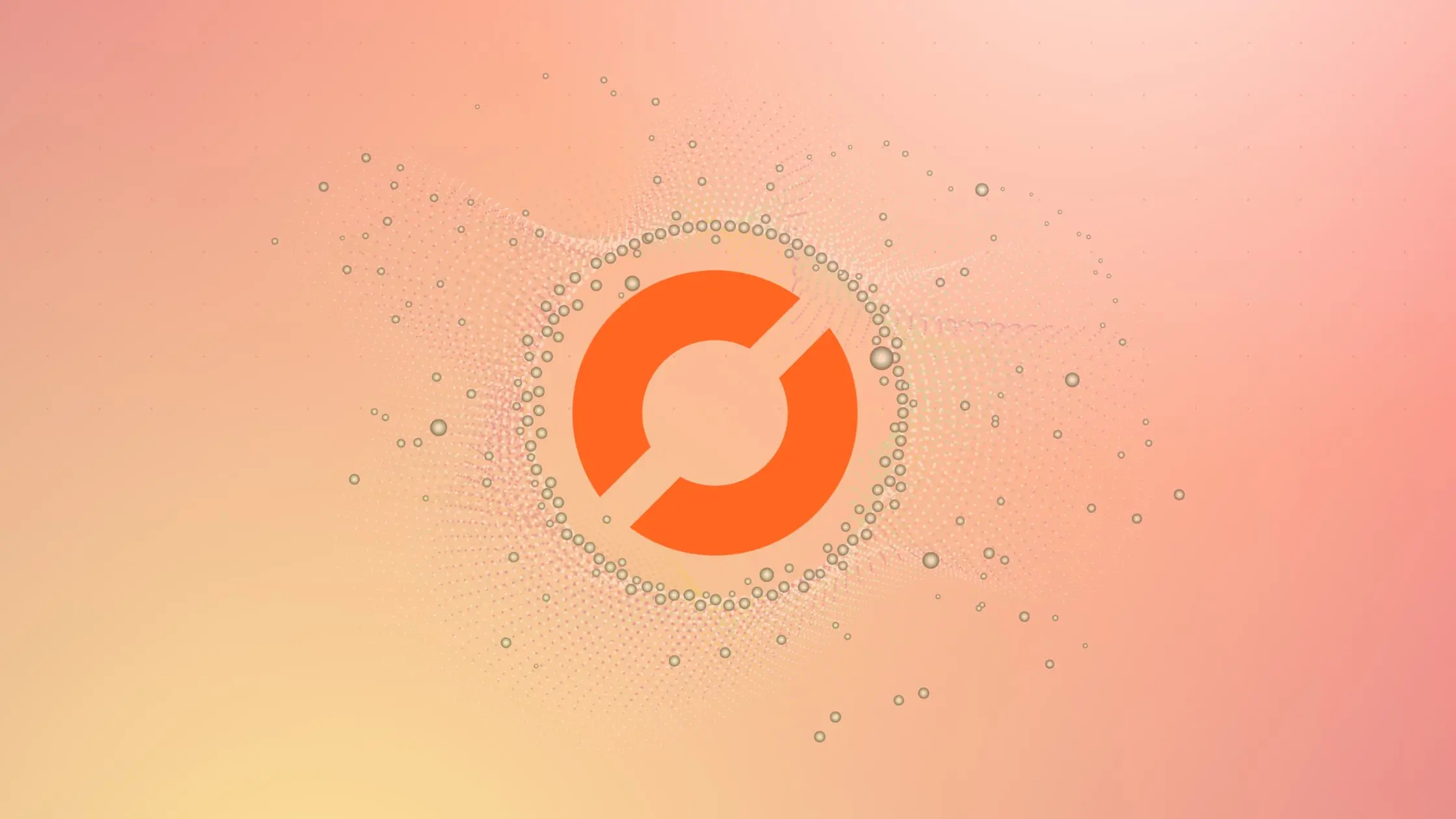
When working with Keras, a popular deep learning library in Python, it’s essential to understand the workflow to effectively build and evaluate your models. One crucial step that often confuses beginners is the need to compile the model before using the model.evaluate()
function. This blog post will delve into why this step is necessary and how to do it correctly.
Table of Contents
- Understanding Keras Model Compilation
- The Role of model.evaluate()
- Why Compile Before Evaluate?
- Compiling and Evaluating in Practice
- Common Errors: Solutions and Examples
- Conclusion
Understanding Keras Model Compilation
Before we can evaluate a Keras model, we need to compile it. But why is this step necessary?
In Keras, compiling the model means configuring the learning process. It’s where you define the optimizer, loss function, and metrics that you want to use. The optimizer is the algorithm that adjusts the weights of the network to minimize the loss function. The loss function, also known as the objective function, is what the model will try to minimize. Metrics are used to judge the performance of your model.
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
In the code snippet above, we’re using the Adam optimizer, the sparse categorical cross-entropy loss function, and accuracy as our metric. These choices depend on the specific problem you’re trying to solve.
The Role of model.evaluate()
The model.evaluate()
function in Keras is used to compute the loss values for input data and, optionally, any metrics that you specified when you compiled the model. It’s typically used after training the model to assess its performance.
loss, accuracy = model.evaluate(test_data, test_labels)
In the code snippet above, model.evaluate()
returns the loss and accuracy of the model on the test data and labels.
Why Compile Before Evaluate?
The reason we need to compile the model before calling model.evaluate()
is that the evaluation process uses the loss function and metrics defined during the compilation step. Without compiling the model, Keras wouldn’t know how to compute the loss or the metrics.
If you try to call model.evaluate()
before compiling the model, you’ll get an error message like this:
AttributeError: 'Sequential' object has no attribute 'loss'
This error occurs because the model’s loss attribute, which is set during compilation, doesn’t exist yet.
Compiling and Evaluating in Practice
Let’s look at a practical example. Suppose we’re working on a binary classification problem. We’ll use a simple sequential model with one dense layer. Here’s how we might define, compile, and evaluate the model:
from keras.models import Sequential
from keras.layers import Dense
# Define the model
model = Sequential()
model.add(Dense(1, activation='sigmoid', input_dim=20))
# Compile the model
model.compile(optimizer='adam',
loss='binary_crossentropy',
metrics=['accuracy'])
# Assume we have training data in train_data and train_labels
model.fit(train_data, train_labels, epochs=10)
# Assume we have test data in test_data and test_labels
loss, accuracy = model.evaluate(test_data, test_labels)
print(f'Test loss: {loss}')
print(f'Test accuracy: {accuracy}')
In this example, we first define the model structure. Then, we compile the model with the Adam optimizer, binary cross-entropy loss function, and accuracy metric. After training the model with model.fit()
, we evaluate it on the test data.
Common Errors: Solutions and Examples
Error 1: Model not compiled before model.evaluate()
# Incorrect
model.evaluate(x_test, y_test)
Solution:
# Correct
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
model.evaluate(x_test, y_test)
Error 2: Modifying the Model Without Recompiling
# Incorrect
model.add(Dense(64))
model.evaluate(x_test, y_test)
Solution:
# Correct
model.add(Dense(64))
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
model.evaluate(x_test, y_test)
Conclusion
Compiling a Keras model before using model.evaluate()
is a crucial step in the workflow of building and assessing deep learning models. It allows you to define the optimizer, loss function, and metrics that the evaluation process will use. Understanding this process will help you avoid errors and build more effective models.
Remember, the choice of optimizer, loss function, and metrics should be guided by the specific problem you’re trying to solve. Keras offers a wide range of options, so you can choose the ones that best fit your needs. Happy modeling!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.