Why is the training time so long for my neural network?
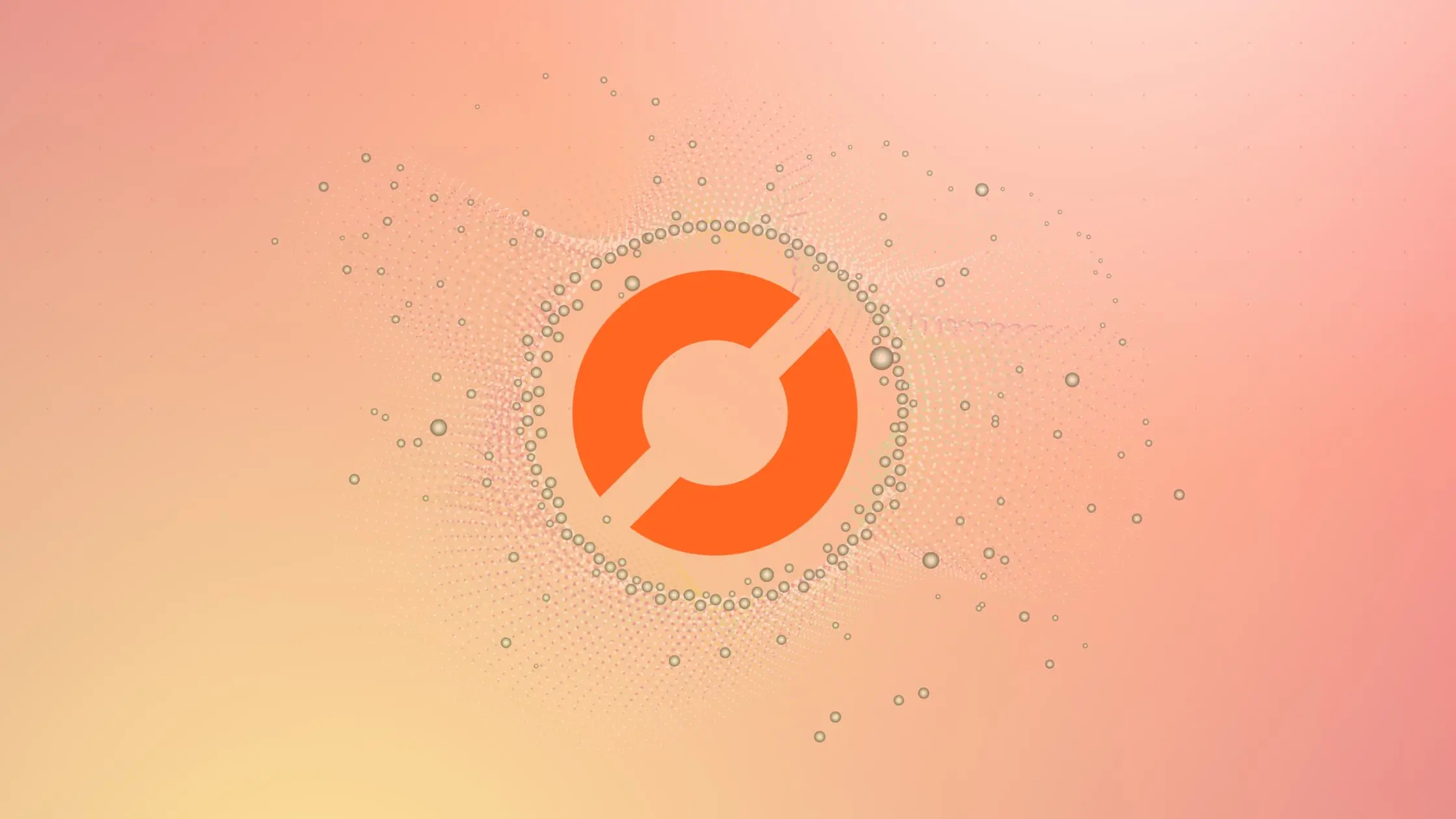
As a data scientist or software engineer, you may have encountered the problem of long training times when working with neural networks. This can be a frustrating issue, particularly when you’re working with large datasets or complex models. In this article, we’ll explore some of the reasons why neural network training times can be so long, and discuss some strategies for improving performance.
Table of Contents
- What is a neural network?
- Reasons for long training times
- Strategies for improving performance
- Conclusion
What is a neural network?
Before we dive into the reasons for long training times, let’s briefly review what a neural network is. A neural network is a type of machine learning model that is loosely inspired by the structure and function of the human brain. It consists of layers of interconnected nodes, called neurons, which process and transmit information.
A neural network typically has an input layer, one or more hidden layers, and an output layer. The input layer receives the data that the model will learn from, and the output layer produces the model’s predictions. The hidden layers perform calculations on the input data, using weights and biases that are learned during training.
Reasons for long training times
Now that we have a basic understanding of neural networks, let’s explore some of the reasons why training times can be so long.
Large datasets
One of the most common reasons for long training times is the size of the dataset. Neural networks require large amounts of data to learn from, and the more data you have, the longer it will take to train the model. This is particularly true if you’re working with complex models that have many layers or parameters.
One way to address this issue is to use a technique called mini-batch training. Rather than training on the entire dataset at once, you can break it up into smaller batches and train on each batch separately. This can help to speed up training times, as the model doesn’t have to process the entire dataset in one go.
Complex models
Another reason for long training times is the complexity of the model itself. As mentioned earlier, neural networks can have many layers and many parameters, and the more complex the model, the longer it will take to train. This is because the model has to perform more calculations and make more adjustments to the weights and biases during training.
One way to address this issue is to use a technique called regularization. Regularization involves adding a penalty term to the loss function that the model is optimizing. This penalty term encourages the model to find simpler solutions, which can help to reduce overfitting and improve performance.
Hardware limitations
Another factor that can contribute to long training times is hardware limitations. Neural networks require a lot of computational power to train, particularly if you’re working with large datasets or complex models. If you’re working on a machine with limited resources, such as a laptop or desktop computer, you may find that training times are prohibitively long.
One solution to this problem is to use a cloud-based computing platform, such as Amazon Web Services or Google Cloud Platform. These platforms offer powerful computing resources that can be used to train neural networks, often at a much faster rate than a local machine.
Strategies for improving performance
Now that we’ve explored some of the reasons for long training times, let’s discuss some strategies for improving performance.
Use pre-trained models
One way to reduce training times is to use pre-trained models. Pre-trained models have already been trained on large datasets, and can be fine-tuned for specific tasks. This can help to reduce the amount of training time required, as the model has already learned some of the underlying patterns in the data.
import torch
import torch.nn as nn
import torch.optim as optim
from torch.utils.data import DataLoader
from torchvision import datasets, transforms, models
# Set device (GPU if available, else CPU)
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
# Download and prepare the CIFAR-10 dataset
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5))
])
train_dataset = datasets.CIFAR10(root='./data', train=True, download=True, transform=transform)
train_loader = DataLoader(train_dataset, batch_size=32, shuffle=True)
# Load pre-trained ResNet18 model
model = models.resnet18(pretrained=True)
model.to(device)
# Freeze all layers except the final fully connected layer
for param in model.parameters():
param.requires_grad = False
# Modify the final fully connected layer for CIFAR-10
model.fc = nn.Linear(model.fc.in_features, 10)
# Optimizer and loss function
optimizer = optim.Adam(model.parameters(), lr=0.001)
criterion = nn.CrossEntropyLoss()
# Training loop
for epoch in range(5): # Adjust the number of epochs as needed
running_loss = 0.0
for i, (inputs, labels) in enumerate(train_loader, 0):
inputs, labels = inputs.to(device), labels.to(device)
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
optimizer.zero_grad()
running_loss += loss.item()
print(f"Epoch {epoch + 1}, Loss: {running_loss / len(train_loader)}")
print("Training finished!")
# Save the pruned model
torch.save(model.state_dict(), 'pruned_resnet18_cifar10.pth')
Optimize hyperparameters
Another strategy for improving performance is to optimize hyperparameters. Hyperparameters are parameters that are set before training, such as the learning rate or the number of layers in the model. Choosing the right hyperparameters can have a significant impact on training times and performance.
One way to optimize hyperparameters is to use a technique called grid search. Grid search involves testing different combinations of hyperparameters to find the best configuration for your model.
Use transfer learning
Transfer learning is a technique that involves using a pre-trained model as a starting point for a new model. This can help to reduce training times, as the new model can leverage the pre-existing knowledge of the pre-trained model. Transfer learning is particularly useful when working with limited amounts of data, as it can help to prevent overfitting.
Gradient Accumulation:
Accumulate gradients over multiple mini-batches before performing a weight update. This helps simulate larger batch sizes without the need for increased memory.
Pros | Cons |
---|---|
Reduced GPU memory requirements | Slower convergence |
Enables training with larger batches | Increased potential for overfitting |
# Gradient accumulation in PyTorch
optimizer.zero_grad()
for i, (inputs, labels) in enumerate(train_loader):
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
if (i + 1) % accumulation_steps == 0:
optimizer.step()
optimizer.zero_grad()
Mixed Precision Training:
Use lower precision (e.g., float16) for training to reduce memory requirements. Handle overflow and underflow issues by careful scaling.
import torch
import torch.nn as nn
import torch.optim as optim
from torch.cuda.amp import autocast, GradScaler
# Define a simple neural network
class SimpleModel(nn.Module):
def __init__(self):
super(SimpleModel, self).__init__()
self.fc = nn.Linear(1000, 100)
def forward(self, x):
x = self.fc(x)
return x
# Instantiate the model and move it to the GPU
model = SimpleModel().cuda()
# Create a dummy dataset
dummy_input = torch.randn((64, 1000)).cuda()
# Define the loss and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
# Create a GradScaler for automatic mixed precision
scaler = GradScaler()
# Training loop
for epoch in range(10):
for inputs, targets in dataloader: # Assuming you have a dataloader
inputs, targets = inputs.cuda(), targets.cuda()
# Clear previous gradients
optimizer.zero_grad()
# Forward pass
with autocast(): # Use autocast to enable mixed precision
outputs = model(inputs)
loss = criterion(outputs, targets)
# Backward pass
scaler.scale(loss).backward()
scaler.step(optimizer)
scaler.update()
# It's important to use scaler.step() and scaler.update() to scale and update gradients appropriately
Conclusion
In conclusion, there are several reasons why training times for neural networks can be so long. These include the size of the dataset, the complexity of the model, and hardware limitations. However, there are also several strategies that can be used to improve performance, such as using pre-trained models, optimizing hyperparameters, and using transfer learning. By implementing these strategies, you can reduce training times and improve the performance of your neural network.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.