Whats the Best Way to Sum all Values in a Pandas Dataframe
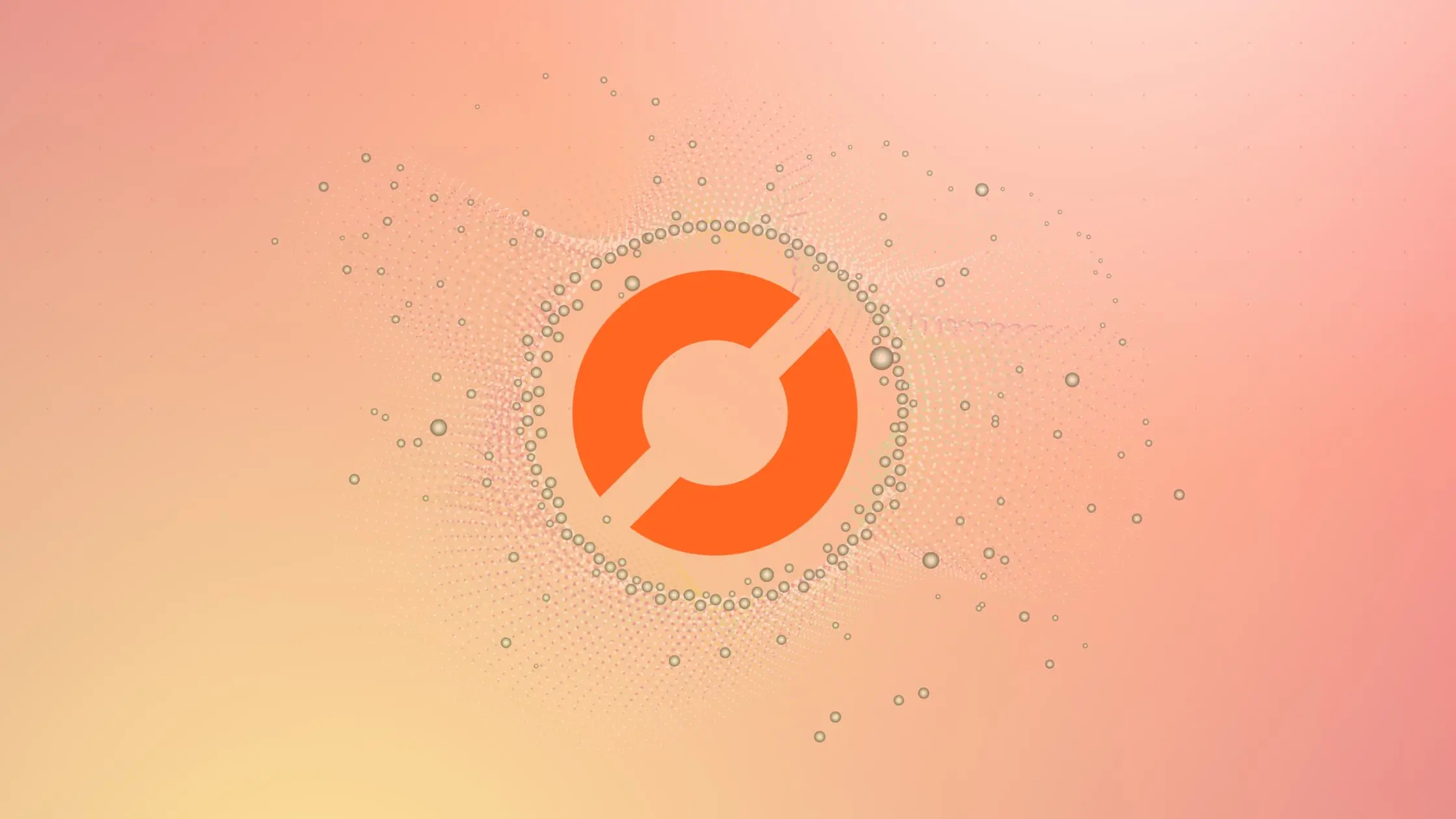
Whats the Best Way to Sum all Values in a Pandas Dataframe
As a data scientist or software engineer, you’ve likely worked with Pandas dataframes before. Pandas is a powerful Python library for data manipulation and analysis, and it’s widely used in the data science community.
One common task when working with dataframes is to sum all the values in the dataframe. This can be useful for getting a quick overview of the data, or for performing calculations on the data.
In this article, we’ll explore the different ways to sum all values in a Pandas dataframe, and discuss the pros and cons of each method. We’ll also provide some best practices for working with dataframes in Pandas.
Method 1: Using the sum() Method
The simplest way to sum all values in a Pandas dataframe is to use the sum()
method. This method computes the sum of each column in the dataframe, and returns a new dataframe with the results.
Here’s an example:
import pandas as pd
# create a dataframe
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# sum all values
total = df.sum().sum()
print(total)
Output:
21
In this example, we create a dataframe with two columns and three rows. We then use the sum()
method twice to compute the sum of all values in the dataframe.
The sum()
method is simple and easy to use, but it has some drawbacks. For one, it can be slow for large dataframes, as it computes the sum of each column separately. Additionally, it may not work correctly for dataframes with missing values (NaNs).
Method 2: Using the numpy Library
Another way to sum all values in a Pandas dataframe is to use the numpy
library. numpy
is a popular library for scientific computing in Python, and it provides fast and efficient numerical operations.
Here’s an example:
import pandas as pd
import numpy as np
# create a dataframe
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# sum all values
total = np.sum(df.values)
print(total)
Output:
21
In this example, we use the values
attribute of the dataframe to get a numpy array of all values. We then use the np.sum()
function to compute the sum of all values in the array.
Using numpy
can be faster than using the sum()
method, especially for large dataframes. However, it may not work correctly for dataframes with missing values (NaNs).
Method 3: Using the applymap() Method
A third way to sum all values in a Pandas dataframe is to use the applymap()
method. This method applies a function to each element of the dataframe, and returns a new dataframe with the results.
Here’s an example:
import pandas as pd
# create a dataframe
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# sum all values
total = df.applymap(lambda x: x).sum().sum()
print(total)
Output:
21
In this example, we use the applymap()
method to apply a lambda function to each element of the dataframe. The lambda function simply returns the element itself, which has the effect of converting the dataframe to a numeric dataframe with no missing values. We then use the sum()
method twice to compute the sum of all values in the dataframe.
Using applymap()
can be slower than using the other methods, as it applies a function to each element of the dataframe. However, it can be more robust for dataframes with missing values (NaNs).
Best Practices for Working with Dataframes in Pandas
When working with dataframes in Pandas, there are some best practices you should follow to ensure your code is efficient and correct:
Avoid using loops: Loops can be slow and inefficient, especially for large dataframes. Instead, use vectorized operations like those provided by
numpy
and Pandas.Use the correct data types: Pandas dataframes can contain different data types for different columns. Using the correct data type can make your code more efficient and prevent errors.
Handle missing values correctly: Pandas dataframes can contain missing values represented by NaNs. Make sure to handle these values correctly in your code.
Use descriptive column names: Use descriptive column names that make it clear what each column represents. This can make your code easier to understand and maintain.
Check your results: Always check your results to make sure they make sense and are consistent with your expectations.
Following these best practices can help you write efficient and correct code when working with dataframes in Pandas.
Conclusion
Summing all values in a Pandas dataframe is a common task in data science and analysis. In this article, we explored three different ways to accomplish this task: using the sum()
method, using the numpy
library, and using the applymap()
method. We also discussed some best practices for working with dataframes in Pandas.
When working with dataframes in Pandas, it’s important to choose the method that’s most appropriate for your data and your use case. By following best practices and choosing the right method for the job, you can write efficient and correct code that gets the job done.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.