What Is the Most Efficient Way of Counting Occurrences in Pandas
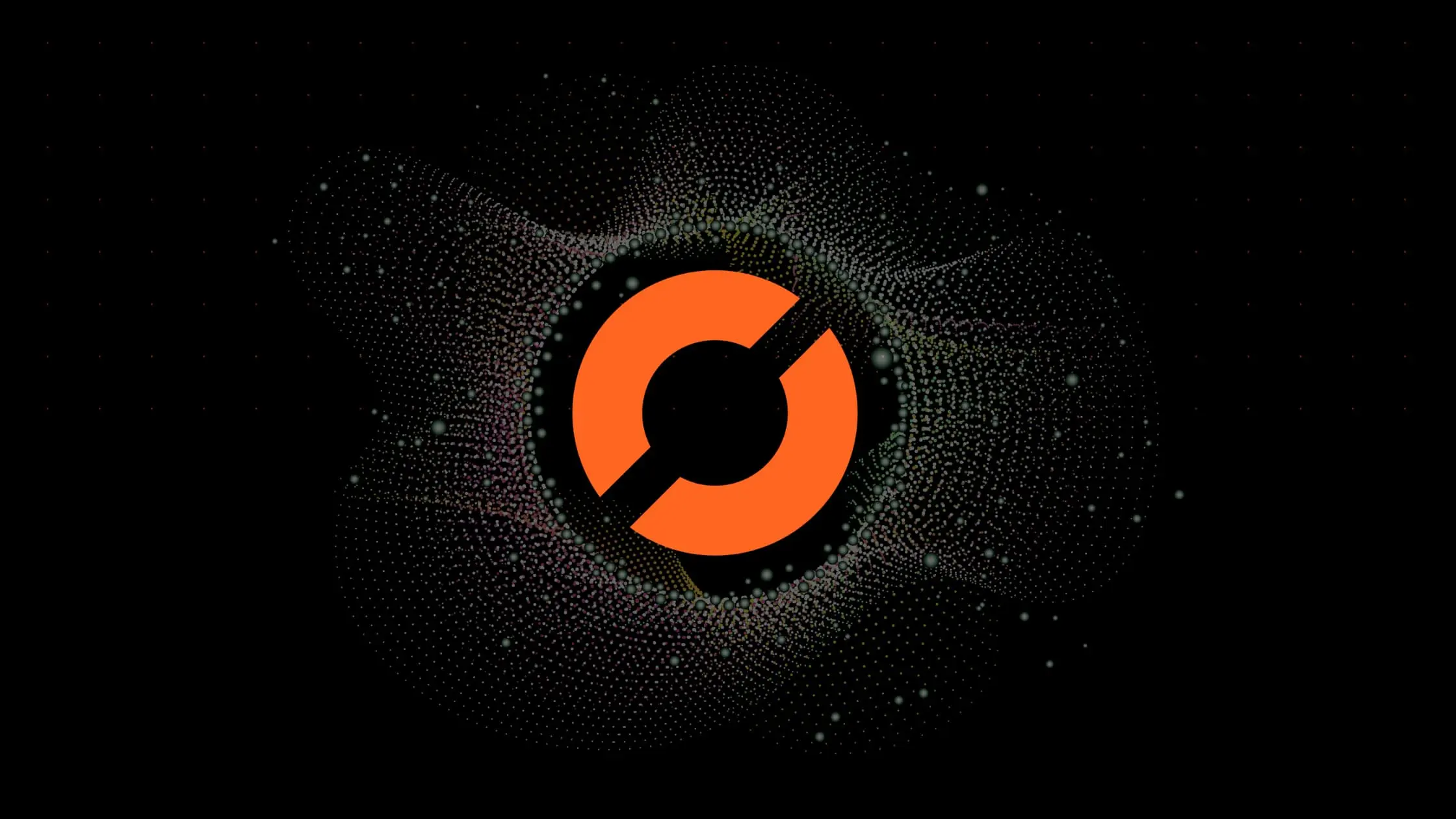
In this article, we will explore the most efficient way of counting occurrences in Pandas. We will cover the basic techniques for counting values, as well as advanced methods that can significantly improve performance when dealing with large datasets.
Counting Values in Pandas
The simplest way to count the occurrences of values in a Pandas DataFrame or Series is to use the value_counts()
method. This method returns a Series containing the counts of unique values in the input data.
Here is an example:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({
'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'foo'],
'B': ['one', 'one', 'two', 'two', 'one', 'two'],
'C': [1, 2, 3, 4, 5, 6]
})
# Count the occurrences of values in column A
counts = df['A'].value_counts()
print(counts)
Output:
foo 4
bar 2
Name: A, dtype: int64
In this example, we created a DataFrame with three columns (A
, B
, and C
) and six rows. We then used the value_counts()
method to count the occurrences of values in column A
. The resulting Series shows that the value foo
occurs four times and the value bar
occurs twice.
The value_counts()
method is simple to use and works well for small to medium-sized datasets. However, it can be slow and memory-intensive for large datasets. In addition, it may not always return the desired output format.
Using GroupBy for Counting
Another way to count occurrences in Pandas is to use the groupby()
method. This method groups the data by one or more columns and applies an aggregation function to each group. To count occurrences, we can use the size()
method, which returns the number of elements in each group.
Here is an example:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({
'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'foo'],
'B': ['one', 'one', 'two', 'two', 'one', 'two'],
'C': [1, 2, 3, 4, 5, 6]
})
# Group by column A and count the occurrences
counts = df.groupby('A').size()
print(counts)
Output:
A
bar 2
foo 4
dtype: int64
In this example, we used the groupby()
method to group the data by column A
and applied the size()
method to each group. The resulting Series shows the counts of values in column A
.
Using groupby()
can be more efficient than value_counts()
for large datasets, especially when grouping by multiple columns. However, it can also be slower for small datasets and may require more code to achieve the desired output format.
Counting Occurrences with a Dictionary
If you need more control over the output format and performance is a concern, you can use a Python dictionary to count occurrences in Pandas. The value_counts()
and groupby()
methods internally use dictionaries to count occurrences, but using a dictionary directly can be faster and more flexible.
Here is an example:
import pandas as pd
# Create a sample DataFrame
df = pd.DataFrame({
'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'foo'],
'B': ['one', 'one', 'two', 'two', 'one', 'two'],
'C': [1, 2, 3, 4, 5, 6]
})
# Count the occurrences of values in column A using a dictionary
counts = {}
for value in df['A']:
counts[value] = counts.get(value, 0) + 1
print(counts)
Output:
{'foo': 4, 'bar': 2}
In this example, we used a for loop to iterate over the values in column A
and count the occurrences using a dictionary. The resulting dictionary shows the counts of values in column A
.
Using a dictionary can be significantly faster than value_counts()
and groupby()
for large datasets, especially when dealing with only one column. However, it requires more code and may not be as flexible as the other methods when dealing with more complex data structures.
Conclusion
Counting occurrences is a fundamental operation in data analysis, and Pandas provides several methods for counting values in a DataFrame or Series. The value_counts()
method is simple to use and works well for small to medium-sized datasets. The groupby()
method is more flexible and can be more efficient for large datasets, especially when grouping by multiple columns. Using a dictionary can be significantly faster for large datasets, especially when dealing with only one column, but requires more code and may not be as flexible as the other methods.
When choosing a method for counting occurrences in Pandas, consider the size and complexity of your data, as well as the desired output format and performance requirements. By choosing the most efficient method for your specific task, you can improve the speed and accuracy of your data analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.