What Is the Difference Between Merge and Concat in Pandas
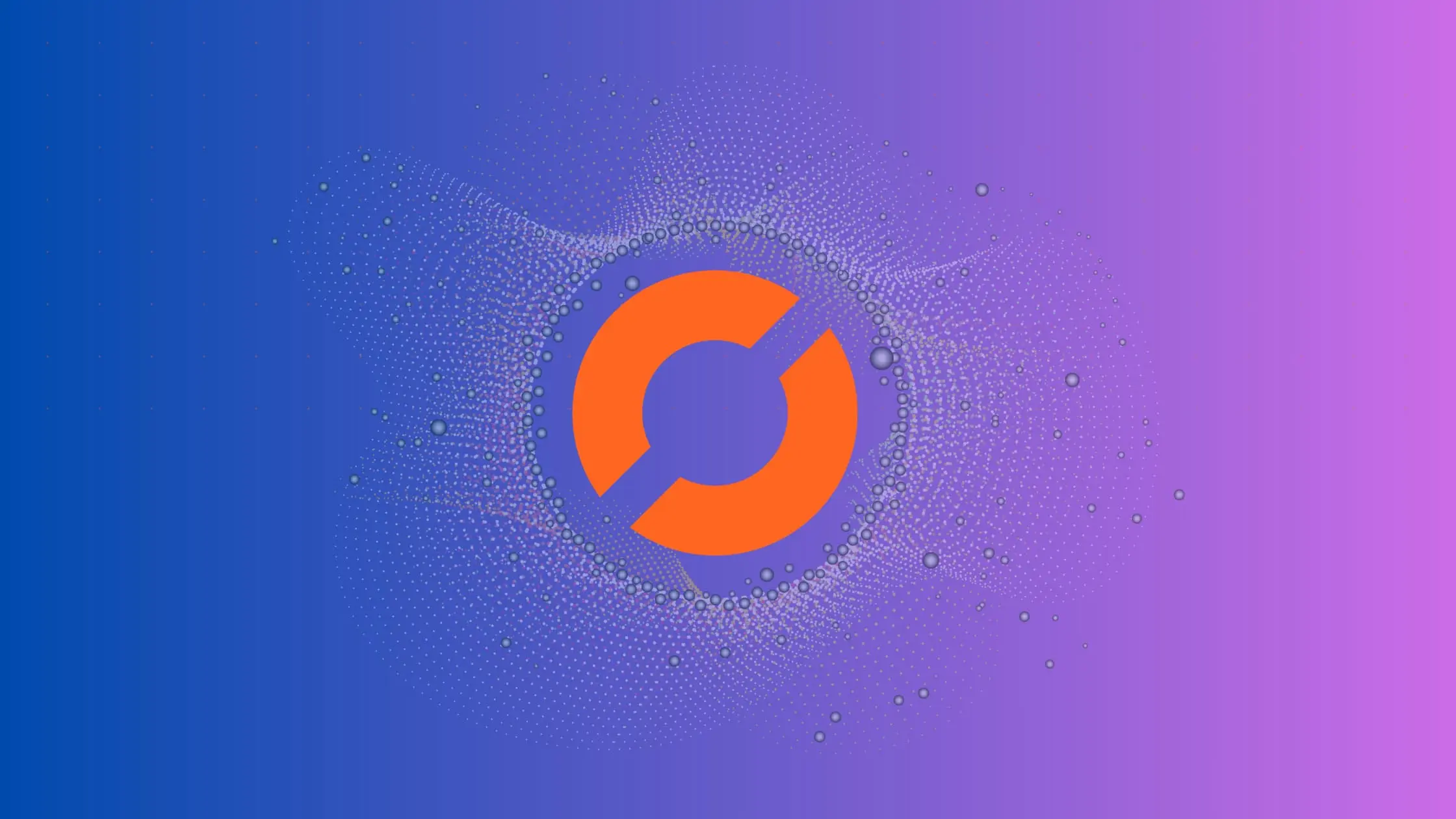
As a data scientist or software engineer, you’ve probably worked with pandas, the popular data manipulation library in Python. Pandas provides a wide range of functions for manipulating and analyzing data, including merge() and concat(). Merge() and concat() are two of the most commonly used functions in pandas, but they have different use cases and syntax. Understanding the difference between merge() and concat() is essential for efficient data manipulation in pandas.
What Is merge()
?
merge()
is a function used to combine two or more data frames on the basis of a common column or index. It is similar to SQL Join operation and can perform left, right, inner or outer join. merge()
can join data frames based on one or more columns. The syntax for merge()
function is as follows:
merged_df = pd.merge(left_df, right_df, on='common_column')
Here, left_df and right_df are the data frames that you want to merge, and ‘common_column’ is the column that is common in both data frames. If the column names are different, you can specify them using the left_on and right_on parameters.
merged_df = pd.merge(left_df, right_df, left_on='left_column', right_on='right_column')
You can also specify the type of join by using the how parameter. The default value of how is 'inner'
, which means only the rows with matching values in both data frames will be included in the merged data frame.
merged_df = pd.merge(left_df, right_df, on='common_column', how='outer')
Let’s see an example:
# Example of merge()
import pandas as pd
# Creating sample data frames
left_df = pd.DataFrame({'key': ['A', 'B', 'C'], 'value': [1, 2, 3]})
right_df = pd.DataFrame({'key': ['A', 'B', 'D'], 'value': [4, 5, 6]})
# Merging based on the 'key' column
merged_df = pd.merge(left_df, right_df, on='key', how='outer')
print(merged_df)
Output:
key value_x value_y
0 A 1.0 4.0
1 B 2.0 5.0
2 C 3.0 NaN
3 D NaN 6.0
What Is concat()
?
concat()
is a function used to concatenate two or more data frames either vertically or horizontally. Concatenation is a process of combining two or more data frames with the same columns. concat()
can concatenate data frames vertically using the axis parameter set to 0 or horizontally using the axis parameter set to 1. The syntax for concat()
function is as follows:
concatenated_df = pd.concat([df1, df2], axis=0)
Here, df1 and df2 are the data frames that you want to concatenate, and axis=0
specifies that the data frames should be concatenated vertically. If you want to concatenate horizontally, you can set axis=1
.
concatenated_df = pd.concat([df1, df2], axis=1)
When you concatenate data frames using concat()
, you can choose to keep the original index or reset the index by using the ignore_index parameter.
concatenated_df = pd.concat([df1, df2], axis=0, ignore_index=True)
Example:
# Example of concat()
import pandas as pd
# Creating sample data frames
df1 = pd.DataFrame({'A': [1, 2], 'B': [3, 4]})
df2 = pd.DataFrame({'A': [5, 6], 'B': [7, 8]})
# Concatenating vertically
concatenated_df = pd.concat([df1, df2], axis=0, ignore_index=True)
print(concatenated_df)
Output:
A B
0 1 3
1 2 4
2 5 7
3 6 8
How Are merge()
and concat()
Different?
While merge()
and concat()
can both be used to combine data frames, they have different use cases and syntax. Here are some of the key differences between merge()
and concat()
:
1. Joining Data Frames
merge()
is used for joining two or more data frames based on a common column or index. On the other hand, concat()
is used for concatenating two or more data frames either vertically or horizontally.
2. Syntax
The syntax for merge()
and concat()
is different. merge()
requires you to specify the common column or index, while concat()
requires you to specify the axis of concatenation.
3. Handling Missing Values
merge()
can handle missing values in different ways by using the how parameter. On the other hand, concat()
simply concatenates data frames without considering missing values.
4. Resulting Data Frame
merge()
returns a new data frame that combines the columns from the two or more data frames that were merged. concat()
, on the other hand, returns a new data frame with the same columns as the original data frames.
Common Errors and How to Handle Them
Error: Duplicate Indices
If you encounter duplicate indices after concatenation, use the ignore_index parameter to reset the index:
result = pd.concat([df1, df2], ignore_index=True)
Error: Non-matching Values
If you encounter non-matching values during merging, check for typos or differences in data types. You can use the how parameter to control the type of merge (e.g., 'inner'
, 'outer'
):
merged_result = pd.merge(df1, df3, on='A', how='inner')
Conclusion
merge()
and concat()
are two of the most commonly used functions in pandas for combining data frames. Understanding the difference between these two functions is essential for efficient data manipulation in pandas. While merge()
is used for joining data frames based on a common column or index, concat()
is used for concatenating data frames either vertically or horizontally. By understanding the syntax and use cases of these two functions, you can choose the right function for your specific data manipulation needs in pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.