What Is the Best Way to Remove Characters from a String in Pandas
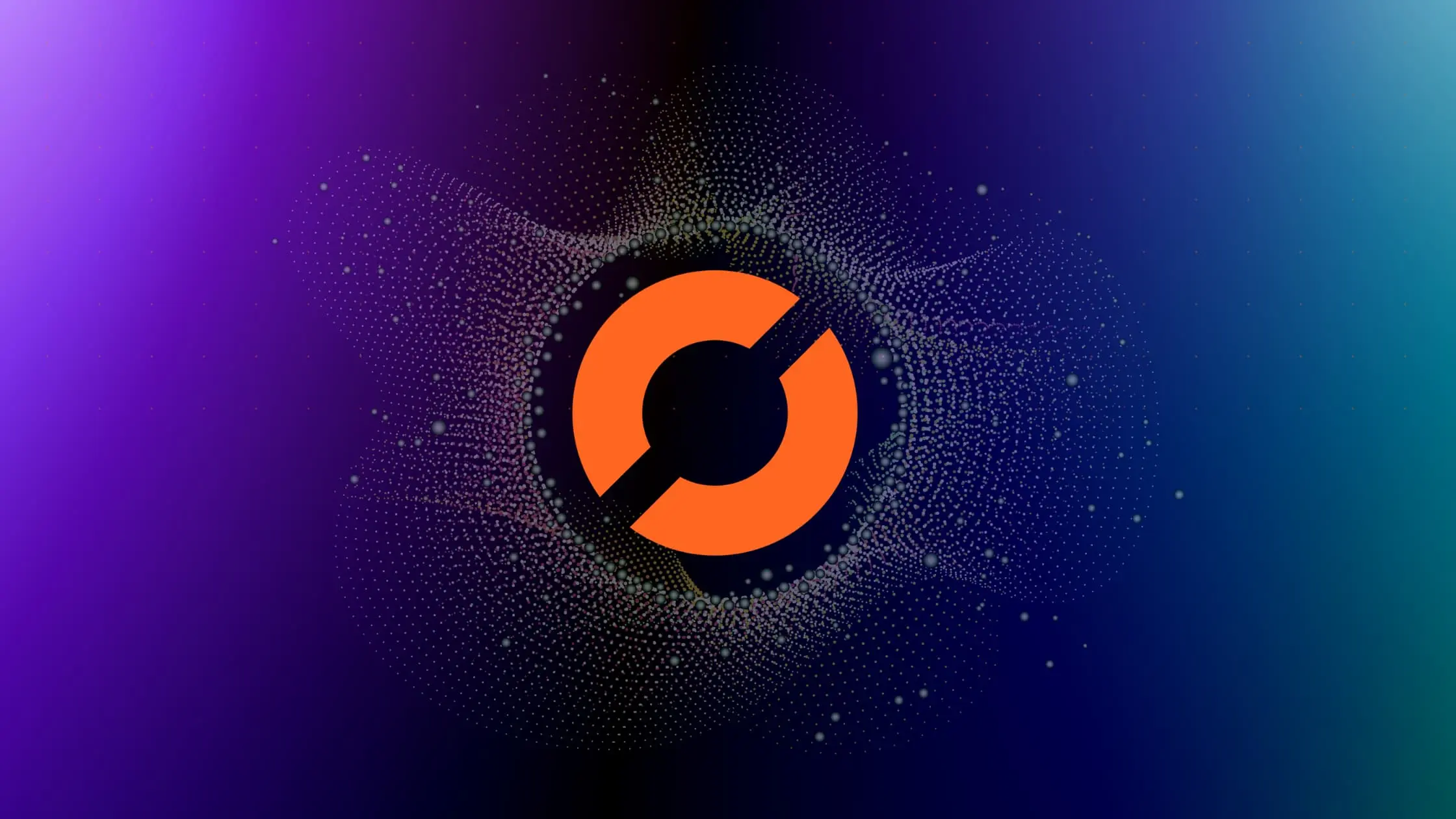
Table of Contents
As a data scientist or software engineer, you know that working with data requires a lot of cleaning and preprocessing. One common task is to remove unwanted characters from strings. In this blog post, we will explore the best ways to remove characters from a string in pandas, a popular data manipulation library in Python.
Understanding the Problem
Before we dive into the solutions, let’s first understand the problem. Suppose you have a column in a pandas DataFrame that contains strings, and you want to remove a specific character or a set of characters from each string. For example, you may want to remove all the commas (',') from a column that contains numbers.
Here’s an example DataFrame:
import pandas as pd
df = pd.DataFrame({'col1': ['apple,banana', 'orange', 'kiwi,grape']})
print(df)
col1
0 apple,banana
1 orange
2 kiwi,grape
Suppose we want to remove all the commas from the ‘col1’ column.
Methods
Using str.replace()
One way to remove characters from a string in pandas is to use the str.replace()
method. This method replaces all occurrences of a substring with another substring.
To remove commas from the ‘col1’ column, we can do:
df['col1'] = df['col1'].str.replace(',', '')
print(df)
col1
0 applebanana
1 orange
2 kiwigrape
The str.replace()
method takes two arguments: the substring to be replaced and the substring to replace it with. In this case, we replace commas with an empty string ('').
Note that the str.replace()
method is case-sensitive. If you want to remove a character regardless of its case, you can use a regular expression with the ’re' module.
Using str.translate()
Another way to remove characters from a string in pandas is to use the str.translate()
method. This method maps each character to another character or deletes it.
To remove commas from the ‘col1’ column using str.translate()
, we can create a translation table that maps commas to None
:
import string
translator = str.maketrans('', '', ',')
df['col1'] = df['col1'].str.translate(translator)
print(df)
col1
0 applebanana
1 orange
2 kiwigrape
The str.maketrans()
method creates a translation table that maps each character in the first argument to the corresponding character in the second argument. In this case, we map commas to None
, which effectively deletes them.
Note that the str.translate()
method is more flexible than str.replace()
because it can map each character to any other character or delete it. However, it can be more difficult to use because you have to create a translation table.
Using apply()
If you need more control over the removal process, you can use the apply()
method to apply a custom function to each element of a column.
Here’s an example function that removes all the commas from a string:
def remove_commas(s):
return s.replace(',', '')
df['col1'] = df['col1'].apply(remove_commas)
print(df)
col1
0 applebanana
1 orange
2 kiwigrape
The apply()
method applies the remove_commas()
function to each element of the ‘col1’ column.
Note that the apply()
method can be slower than the other methods because it applies a function to each element of a column sequentially. However, it is more flexible because you can define any custom function to remove characters.
Handling Common Errors
Mismatched Data Types:
Error Scenario:
If the data type of your column is not a string-like type, applying string manipulation methods directly may result in unexpected behavior or errors.
import pandas as pd
# Sample DataFrame with a numeric column
data = {'numeric_text': [123, 456, 789]}
df = pd.DataFrame(data)
# Attempting to remove digits directly on a non-string column
df['numeric_text'] = df['numeric_text'].str.replace('\d', '')
Output:
AttributeError: Can only use .str accessor with string values!
Handling the Error:
To handle this error, convert the column to a string-like type using the astype(str)
method before applying string manipulation:
df['numeric_text'] = df['numeric_text'].astype(str).str.replace('\d', '')
print(df)
Output:
numeric_text
0
1
2
Case Sensitivity:
Error Scenario:
String manipulation methods like str.replace()
are case-sensitive by default. Failing to account for case sensitivity can lead to incomplete replacements.
import pandas as pd
# Sample DataFrame
data = {'text': ['Hello, World', 'hello, world', 'HELLO, WORLD']}
df = pd.DataFrame(data)
# Attempting to replace 'hello' with 'hi' without case sensitivity
df['text'] = df['text'].str.replace('hello', 'hi')
print(df)
Output:
text
0 Hello, World
1 hi, world
2 HELLO, WORLD
Handling the Error:
To make the replacement case-insensitive, use the case
parameter:
df['text'] = df['text'].str.replace('hello', 'hi', case=False)
Output:
text
0 hi, World
1 hi, world
2 hi, WORLD
Conclusion
In this blog post, we explored the best ways to remove characters from a string in pandas. We learned that we can use the str.replace()
method, the str.translate()
method, or the apply()
method to remove characters from a column of strings.
Which method you choose depends on your specific use case. If you want a simple and fast solution, use str.replace()
. If you need more flexibility, use str.translate()
. If you need even more control, use apply()
with a custom function.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.