What Is Appending to an Empty DataFrame in Pandas
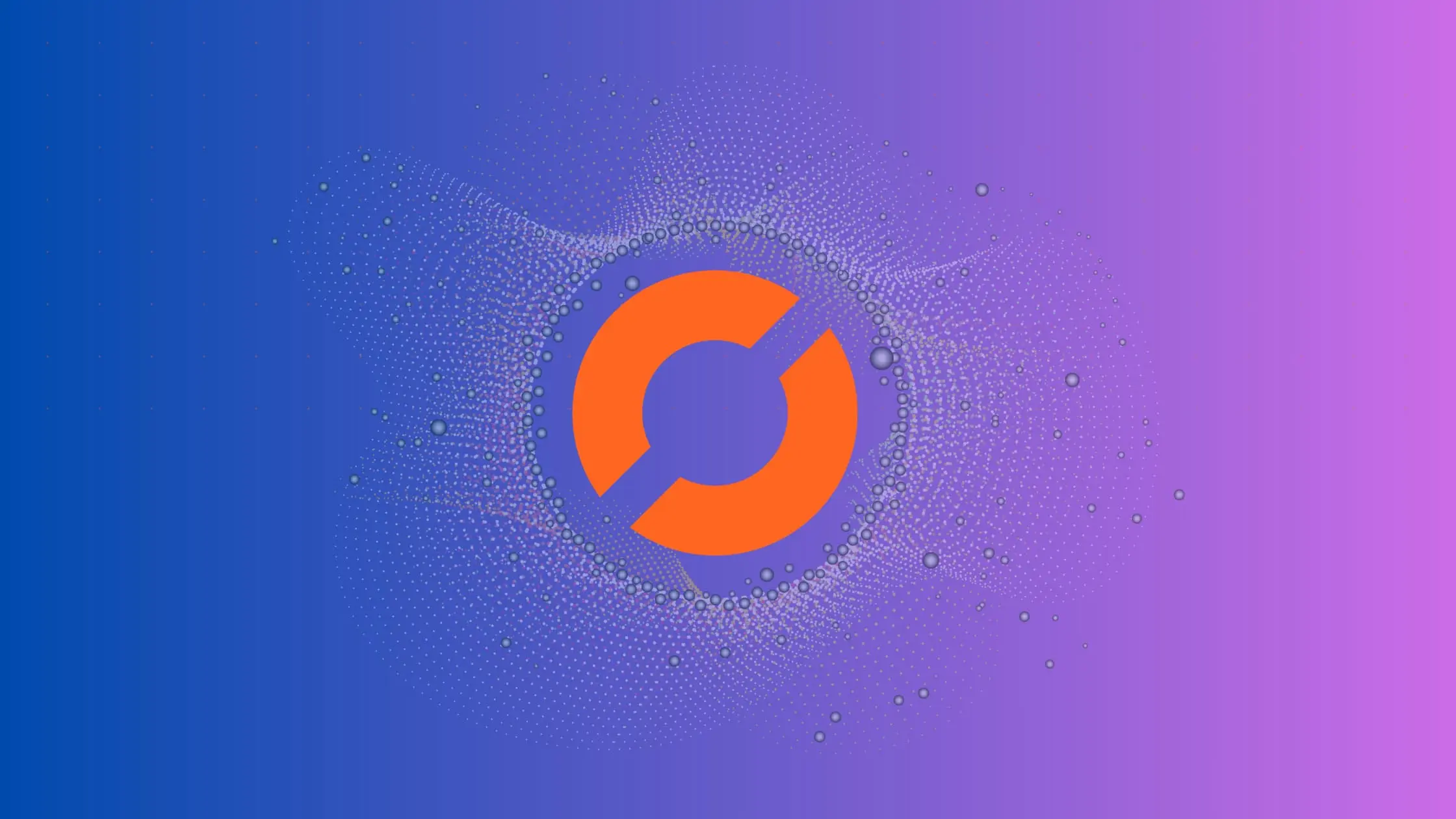
As a data scientist or software engineer, you will often encounter situations where you need to manipulate data using a programming language. One of the most commonly used tools for data manipulation is Pandas, a Python library that provides powerful data structures and functions for working with tabular data.
In this blog post, we will explore the topic of appending to an empty DataFrame in Pandas. We will explain what an empty DataFrame is, why you might need to append data to it, and how to do it using Pandas.
What Is an Empty DataFrame?
A DataFrame is a two-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or SQL table, but with more powerful features. An empty DataFrame is simply a DataFrame with no rows or columns.
Empty DataFrames are often used as a starting point for data manipulation tasks. They can be created using the pd.DataFrame()
function in Pandas, as shown below:
# import library
import pandas as pd
# create an empty DataFrame
df = pd.DataFrame()
print(df)
Output:
Empty DataFrame
Columns: []
Index: []
This creates an empty DataFrame with no rows or columns. Once you have an empty DataFrame, you can append data to it using the append()
function.
Why Append Data to an Empty DataFrame?
Appending data to an empty DataFrame is useful in situations where you want to create a DataFrame from scratch. For example, you might have data stored in different files or databases, and you want to combine them into a single DataFrame. Or, you might want to create a DataFrame to store data that you will generate using Python code.
Appending data to an empty DataFrame can also be useful if you want to start with a DataFrame that has the same columns as another DataFrame, but with no rows. You can then append data to this DataFrame as needed.
How to Append Data to an Empty DataFrame in Pandas
Appending data to an empty DataFrame in Pandas is straightforward. You can use the append()
function to append rows to an existing DataFrame, or to create a new DataFrame by appending rows to an empty DataFrame.
Appending Rows to an Existing DataFrame
Using append()
To append rows to an existing DataFrame, you can use the append()
function as follows:
import pandas as pd
# create an empty DataFrame
df = pd.DataFrame()
# create a new DataFrame with some data
new_data = pd.DataFrame({'col1': [1, 2, 3], 'col2': [4, 5, 6]})
# append the new DataFrame to the existing DataFrame
new_df = df.append(new_data, ignore_index=True)
print('Before Appending:')
print(df)
print('After Appending:')
print(new_df)
Output:
Before Appending:
Empty DataFrame
Columns: []
Index: []
After Appending:
col1 col2
0 1 4
1 2 5
2 3 6
In this example, we first create an empty DataFrame using pd.DataFrame()
. We then create a new DataFrame called new_data
with some data. Finally, we use the append()
function to append the new DataFrame to the existing DataFrame.
Note that we use the ignore_index=True
argument to reset the index of the appended DataFrame. If we didn’t use this argument, the index of the new DataFrame would be preserved when it is appended to the existing DataFrame.
Using pandas.concat()
You can also use pandas.concat
to append new rows to an empty DataFrame.
import pandas as pd
# create an empty DataFrame
df = pd.DataFrame()
# create a new DataFrame with some data
new_data = pd.DataFrame({'col1': [1, 2, 3], 'col2': [4, 5, 6]})
# using pd.concat to append the new DataFrame to the existing DataFrame
new_df = pd.concat([df, new_data], ignore_index=True)
print('Before Appending:')
print(df)
print('After Appending:')
print(new_df)
Output:
Before Appending:
Empty DataFrame
Columns: []
Index: []
After Appending:
col1 col2
0 1 4
1 2 5
2 3 6
Conclusion
In this blog post, we have explained what an empty DataFrame is, why you might need to append data to it, and how to do it using Pandas. Appending data to an empty DataFrame is a useful technique for creating a DataFrame from scratch or for adding rows to an existing DataFrame. With the append()
function in Pandas, it is easy to append data to an empty DataFrame, and we hope this blog post has helped you understand how to do it.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.