Using Weights in CrossEntropyLoss and BCELoss (PyTorch)
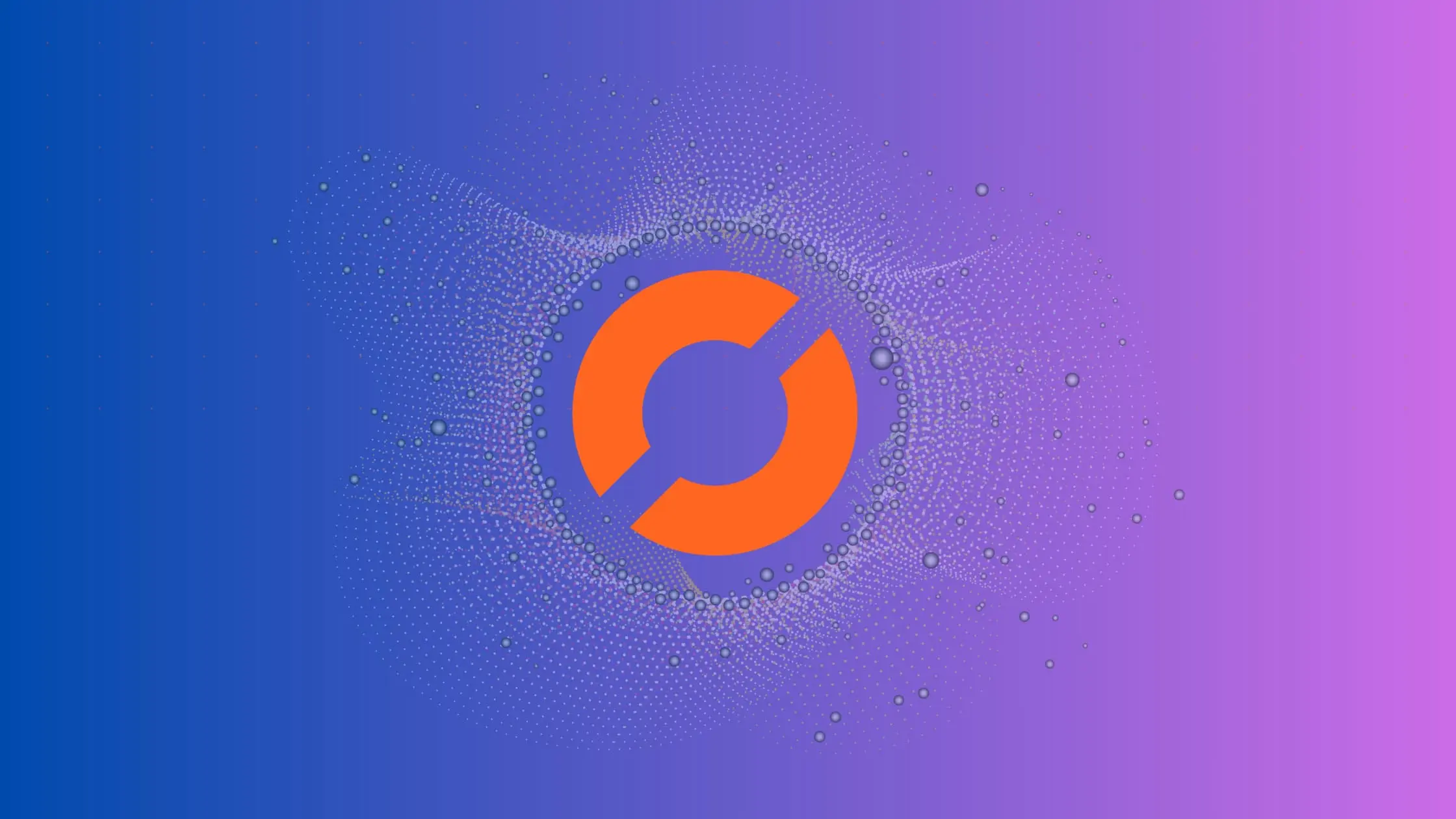
As a data scientist or software engineer, you are probably familiar with the concept of loss functions. In machine learning, loss functions are used to measure how well a model is able to predict the correct outcome. One common type of loss function is the CrossEntropyLoss, which is used for multi-class classification problems. Another commonly used loss function is the Binary Cross Entropy (BCE) Loss, which is used for binary classification problems. In this blog post, we will discuss how to use weights in these two loss functions using PyTorch.
Table of Contents
- What is CrossEntropyLoss?
- What is BCELoss?
- Using Weights in CrossEntropyLoss
- Using Weights in BCELoss
- Pros of Using Weighted Loss Functions
- Cons of Using Weighted Loss Functions
- Common Error Handling in Weighted Loss Functions
- Conclusion
What is CrossEntropyLoss?
CrossEntropyLoss is a loss function that is used for multi-class classification problems. It is a combination of the Softmax activation function and the Negative Log Likelihood (NLL) Loss function. The Softmax function is used to convert the output of the final layer of a neural network into probabilities. The NLL Loss function is then used to calculate the error between the predicted probabilities and the true labels.
The formula for the CrossEntropyLoss is as follows:
$$ l_n = -w_{y_n} \log \left( \frac{\exp(x_{n,y_n})}{\sum_{c=1}^{C} \exp(x_{n,c})} \right) $$
where x
is the input, y
is the target, w
is the weight and C
is the number of classes.
What is BCELoss?
BCELoss, or Binary Cross Entropy Loss, is a loss function that is used for binary classification problems. It is similar to the CrossEntropyLoss, but it is used for problems where there are only two classes. The BCELoss is calculated as follows:
loss(x, class) = -w * [class * log(x) + (1 - class) * log(1 - x)]
$$ l_n = -w_n \left[ y_n \cdot \log(x_n) + (1 - y_n) \cdot \log(1 - x_n) \right] $$
where x
is the predicted probability of the positive class and class
is the true label (either 0 or 1). The w
term is used to weight the loss of each class. By default, w
is set to 1 for both classes, which means that the loss of both classes is equally important. However, we can set w
to different values to give more importance to one class over the other.
where x
is the input, y
is the target and w
is the weight.
Using Weights in CrossEntropyLoss
In some cases, we may want to give more importance to certain classes in a multi-class classification problem. For example, if we are working on a medical diagnosis problem and we want to predict whether a patient has cancer or not, we may want to give more importance to the positive class (i.e., the class that represents patients with cancer) because false negatives (i.e., predicting that a patient does not have cancer when they actually do) can be more harmful than false positives (i.e., predicting that a patient has cancer when they actually do not).
To give more importance to a certain class in the CrossEntropyLoss, we can use the weight
parameter in the PyTorch implementation of the loss function. The weight
parameter is a 1D Tensor that contains the weight of each class. The length of the weight
parameter should be equal to the number of classes.
Here is an example of how to use weights in the CrossEntropyLoss:
import torch
import torch.nn as nn
import torch.optim as optim
# define the weights for each class
weights = torch.tensor([1.0, 1.0, 2.0])
# define the CrossEntropyLoss with weights
criterion = nn.CrossEntropyLoss(weight=weights)
# define the inputs and labels
inputs = torch.randn(10, 3)
labels = torch.tensor([0, 1, 2, 1, 0, 2, 0, 1, 0, 1])
# calculate the loss
loss = criterion(inputs, labels)
In this example, we have defined three classes and assigned a weight of 2.0 to the third class. This means that the loss of the third class will be multiplied by 2.0, which makes it twice as important as the other classes.
Using Weights in BCELoss
In binary classification, assigning more weight to one class is often necessary. For instance, in fraud detection, emphasizing the positive class (fraudulent transactions) reduces costly false negatives. PyTorch’s BCEWithLogitsLoss
has a pos_weight
parameter for this purpose. This scalar weights the positive class’s loss.
Here’s how to use pos_weight
in BCEWithLogitsLoss
:
import torch
import torch.nn as nn
# Weight for the positive class
pos_weight = torch.tensor([2.0])
# BCEWithLogitsLoss with pos_weight
criterion = nn.BCEWithLogitsLoss(pos_weight=pos_weight)
# Inputs and labels
logits = torch.tensor([0.2, 0.8, 0.6, 0.4])
labels = torch.tensor([0, 1, 0, 0], dtype=torch.float)
# Calculate loss
loss = criterion(logits, labels)
In this example, we have defined a weight of 2.0 for the positive class. This means that the loss of the positive class will be multiplied by 2.0, which makes it twice as important as the negative class.
Pros of Using Weighted Loss Functions
- Handling Class Imbalance: Weighted loss functions are particularly beneficial in datasets with class imbalances. By assigning higher weights to underrepresented classes, the model can learn to pay more attention to these classes, potentially improving its overall accuracy in real-world scenarios.
- Customization for Specific Needs: The flexibility to assign different weights allows for customization according to the specific requirements of a task. For example, in medical diagnostics, prioritizing sensitivity over specificity can be crucial.
- Improved Model Performance: By focusing on critical classes, weighted loss functions can enhance model performance, especially in cases where certain misclassifications have more severe consequences.
Cons of Using Weighted Loss Functions
- Risk of Overfitting: Overemphasizing certain classes might lead the model to overfit to those classes, potentially at the expense of overall accuracy.
- Difficulty in Choosing the Right Weights: Determining the appropriate weights can be challenging. Incorrect weighting might lead to suboptimal model performance.
- Increased Complexity: Introducing weights adds another layer of complexity to the model training process, which can make it more challenging to debug and tune the model.
Common Error Handling in Weighted Loss Functions
- Ensuring Correct Weight Shape and Type: Verify that the weight tensors have the correct shape and data type. Mismatches can lead to runtime errors.
- Balancing Weight Magnitude: When setting weights, ensure they are not disproportionately high or low, as extreme weights can destabilize the learning process.
- Monitoring Model Performance: Regularly monitor the model’s performance on a validation set to check for signs of overfitting or underfitting.
- Adjusting Weights Based on Performance: If the model shows bias towards certain classes, iteratively adjust the weights to find a more balanced approach.
- Avoiding Zero or Negative Weights: Ensure that weights are positive and non-zero to prevent invalid computations or unintentional ignoring of a class.
Conclusion
In this blog post, we have discussed how to use weights in the CrossEntropyLoss and BCELoss in PyTorch. By using weights, we can give more importance to certain classes in a multi-class or binary classification problem. This can be useful in situations where certain classes are more important than others, such as in medical diagnosis or fraud detection.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.