Using Lambda Function Pandas to Set Column Values
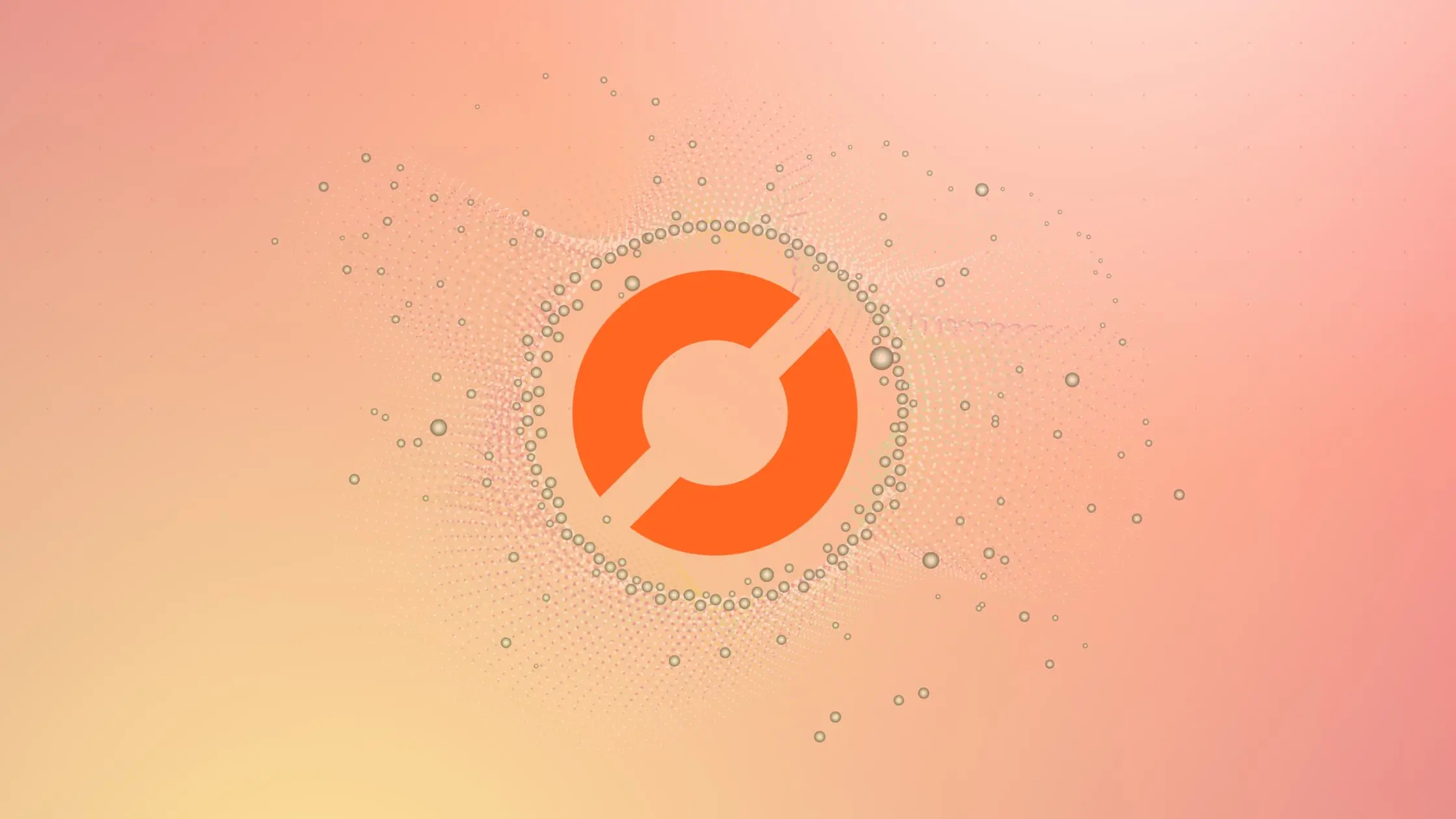
As a data scientist or software engineer, you may have come across the need to manipulate data in a Pandas DataFrame. One common task is to set column values based on certain conditions. In this blog post, we will explore how to use a lambda function in Pandas to set column values.
Table of Contents
- What is a Pandas DataFrame?
- Setting Column Values with a Lambda Function
- More Advanced Examples
- Common Errors and Solutions
- Best Practices
- Conclusion
What is a Pandas DataFrame?
A Pandas DataFrame is a two-dimensional table-like data structure with rows and columns. It is a popular data structure in Python for data manipulation and analysis. Pandas provides many functions to manipulate and analyze data in a DataFrame.
Setting Column Values with a Lambda Function
A lambda function is a small anonymous function in Python. It can take any number of arguments, but can only have one expression. A lambda function can be used as an argument for other functions or used to create a new function on the fly.
To set column values in a Pandas DataFrame, we can use the .apply()
function along with a lambda function. The .apply()
function applies a function to each element of a DataFrame. We can use a lambda function inside the .apply()
function to set column values based on certain conditions.
Let’s take a look at an example. Suppose we have a DataFrame df
with columns A
, B
, and C
. We want to set the values in column C
based on the values in columns A
and B
. If the value in column A
is greater than the value in column B
, we want to set the value in column C
to True
. Otherwise, we want to set it to False
.
We can use the following lambda function to set the values in column C
:
df['C'] = df.apply(lambda row: True if row['A'] > row['B'] else False, axis=1)
print(df)
In this lambda function, we are applying the if
statement to each row of the DataFrame. If the condition row['A'] > row['B']
is true, we set the value in column C
to True
. Otherwise, we set it to False
. The axis=1
argument tells the .apply()
function to apply the lambda function to each row of the DataFrame.
Output:
A B C
0 5 3 True
1 8 9 False
2 12 6 True
3 4 15 False
More Advanced Examples
Lambda functions can be used to set column values based on even more complex conditions. Let’s take a look at a few more examples.
Example 1: Setting Values Based on Multiple Conditions
Suppose we have a DataFrame df
with columns A
, B
, and C
. We want to set the values in column C
based on the values in columns A
and B
. If the value in column A
is greater than the value in column B
and the value in column A
is less than 10, we want to set the value in column C
to True
. Otherwise, we want to set it to False
.
We can use the following lambda function to set the values in column C
:
df['C'] = df.apply(lambda row: True if row['A'] > row['B'] and row['A'] < 10 else False, axis=1)
print(df)
In this lambda function, we are applying two conditions to each row of the DataFrame. If both conditions are true, we set the value in column C
to True
. Otherwise, we set it to False
.
Output:
A B C
0 5 3 True
1 8 9 False
2 12 6 False
3 4 15 False
Example 2: Setting Values Based on a Dictionary
Suppose we have a DataFrame df
with columns A
, B
, and C
. We want to set the values in column C
based on a dictionary that maps values in column A
to values in column C
.
We can use the following lambda function to set the values in column C
:
mapping = {4: 'Four', 5: 'Five', 8: 'Eight', 12: 'Twelve'}
df['C'] = df.apply(lambda row: mapping[row['A']], axis=1)
print(df)
In this lambda function, we are using a dictionary to map values in column A
to values in column C
. The axis=1
argument tells the .apply()
function to apply the lambda function to each row of the DataFrame.
Output:
A B C
0 5 3 Five
1 8 9 Eight
2 12 6 Twelve
3 4 15 Four
Creating the DataFrame df
:
import pandas as pd
data = {'A': [5, 8, 12, 4],
'B': [3, 9, 6, 15]}
df = pd.DataFrame(data)
Common Errors and Solutions
1. Error 1: DataFrame Columns Do Not Exist
# Error
df['C'] = df.apply(lambda row: True if row['X'] > row['Y'] else False, axis=1)
# Solution
# Ensure that the column names 'X' and 'Y' exist in your DataFrame.
# Double-check column names for typos or case sensitivity issues.
2. Error 2: Incorrect Lambda Function Syntax
# Error
df['C'] = df.apply(lambda row True if row['A'] > row['B'] else False, axis=1)
# Solution
# Ensure correct lambda function syntax by adding a colon after 'lambda row'.
3. Error 3: Incorrect Usage of axis
Parameter
# Error
df['C'] = df.apply(lambda row: True if row['A'] > row['B'] else False, axis=0)
# Solution
# Use axis=1 for applying the lambda function to each row.
# Using axis=0 would apply it to each column, which is not the desired behavior in this case.
Best Practices
1. Use Vectorized Operations When Possible:
- Instead of applying a lambda function using
apply
, try to use vectorized operations, which are generally faster and more efficient.
Example:
df['C'] = (df['A'] > df['B']).astype(bool)
2. Handle Missing Values Appropriately:
- Check for and handle missing values before applying lambda functions to avoid unexpected behavior.
Example:
df.dropna(subset=['A', 'B'], inplace=True)
3. Use .loc
for Conditional Updates:
- For setting values based on conditions, consider using
.loc
instead ofapply
for improved readability.
Example:
df.loc[df['A'] > df['B'], 'C'] = True
df.loc[df['A'] <= df['B'], 'C'] = False
Conclusion
In this blog post, we explored how to use a lambda function in Pandas to set column values based on certain conditions. We saw how to use the .apply()
function along with a lambda function to set column values. We also saw some more advanced examples of using lambda functions to set column values based on multiple conditions or a dictionary.
Lambda functions are a powerful tool in Python for manipulating data. They can be used to create new functions on the fly and apply them to data structures like Pandas DataFrames. By using lambda functions in Pandas, you can quickly and easily manipulate your data to meet your needs.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.