Understanding the Differences Between Numpy Reshape(-1, 1) and Reshape(1, -1)
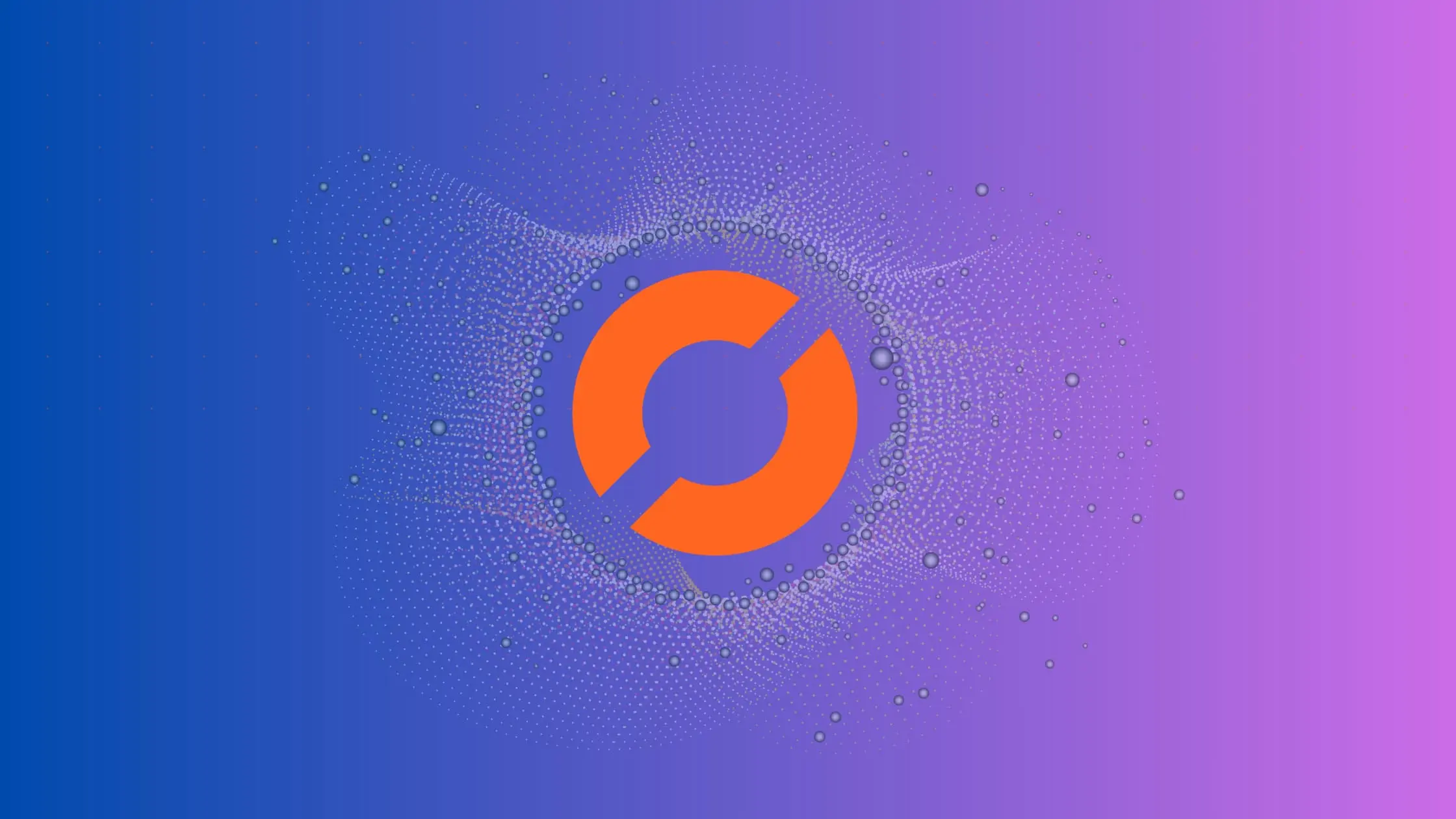
Numpy is a powerful library in Python that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. One of the most commonly used functions in Numpy is reshape()
, which gives a new shape to an array without changing its data. In this blog post, we will delve into the differences between reshape(-1, 1)
and reshape(1, -1)
.
Table of Contents
Understanding Numpy Reshape Function
Before we dive into the differences, let’s first understand what the reshape()
function does. This function allows you to change the dimensions of your array. The syntax is as follows:
numpy.reshape(a, newshape)
Here, a
is the array to be reshaped, and newshape
is an integer or tuple of integers that denote the new shape. The new shape should be compatible with the original shape. If an integer, then the result will be a 1-D array of that length.
The reshape()
function can take parameters like (1, -1)
or (-1, 1)
. The -1
is a placeholder that means “adjust this dimension to make the data fit”.
Reshape(-1, 1)
When you use reshape(-1, 1)
, you are asking numpy to reshape your array with 1 column and as many rows as necessary to accommodate the data. This operation will result in a 2D array with a shape (n, 1)
, where n
is the number of elements in your original array.
Here’s an example:
import numpy as np
# Original array
arr = np.array([1, 2, 3, 4, 5, 6])
print('Original array shape:', arr.shape)
# Reshape array
reshaped_arr = arr.reshape(-1, 1)
print('Reshaped array shape:', reshaped_arr.shape)
Output:
Original array shape: (6,)
Reshaped array shape: (6, 1)
Reshape(1, -1)
On the other hand, reshape(1, -1)
reshapes your array with 1 row and as many columns as necessary to accommodate the data. This operation will result in a 2D array with a shape (1, n)
, where n
is the number of elements in your original array.
Here’s an example:
import numpy as np
# Original array
arr = np.array([1, 2, 3, 4, 5, 6])
print('Original array shape:', arr.shape)
# Reshape array
reshaped_arr = arr.reshape(1, -1)
print('Reshaped array shape:', reshaped_arr.shape)
Output:
Original array shape: (6,)
Reshaped array shape: (1, 6)
Key Differences
The key difference between reshape(-1, 1)
and reshape(1, -1)
is the shape of the resulting array. reshape(-1, 1)
results in an array with a single column and multiple rows (a column vector), while reshape(1, -1)
results in an array with a single row and multiple columns (a row vector).
These differences are important to understand because they can impact how you perform operations on your arrays. For instance, if you’re performing matrix multiplication, the shape of your arrays matters significantly.
Conclusion
Understanding the nuances of the reshape()
function in numpy is crucial for data manipulation and preprocessing. The difference between reshape(-1, 1)
and reshape(1, -1)
may seem subtle, but it can have a significant impact on your data operations. Always ensure that you understand the shape of your arrays when using numpy’s reshape()
function.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.