Understanding the Difference Between Flatten() and GlobalAveragePooling2D() in Keras
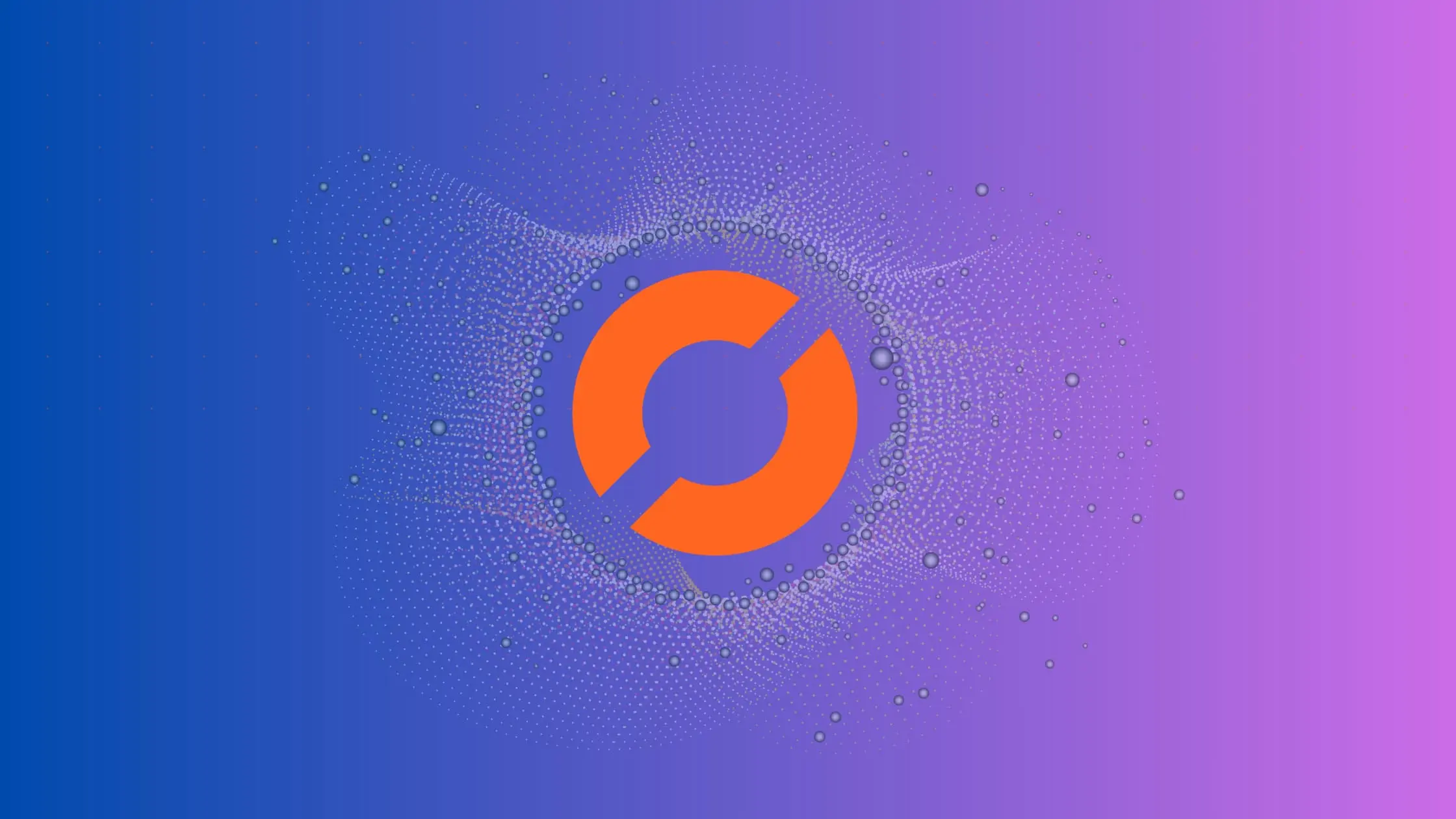
When working with convolutional neural networks (CNNs) in Keras, you’ll often need to reshape your data or reduce its dimensionality. Two common methods for this are Flatten()
and GlobalAveragePooling2D()
. While they may seem similar, they serve different purposes and can significantly impact your model’s performance. This post will delve into the differences between these two functions, their use cases, and how to implement them in Keras.
Table of Contents
- What is
Flatten()
in Keras? - What is
GlobalAveragePooling2D()
in Keras? - The Key Differences
- When to Use
Flatten()
vsGlobalAveragePooling2D()
? - Common Errors and How to Handle Them
- Conclusion
What is Flatten()
in Keras?
Flatten()
is a function in Keras that transforms a multi-dimensional tensor into a one-dimensional tensor (vector). It does this by preserving the batch size and combining all other dimensions.
Here’s a simple example of how Flatten()
works:
from keras.models import Sequential
from keras.layers import Flatten
model = Sequential()
model.add(Flatten(input_shape=(3,3)))
In this example, the Flatten()
layer transforms a 3x3 input into a 1D tensor with nine elements.
What is GlobalAveragePooling2D()
in Keras?
GlobalAveragePooling2D()
is another function in Keras that reduces the spatial dimensions of a tensor. Unlike Flatten()
, which simply reshapes the data, GlobalAveragePooling2D()
performs an operation on the data. It calculates the average value of each feature map in the input tensor and outputs a tensor that is smaller in size.
Here’s how you can use GlobalAveragePooling2D()
:
from keras.models import Sequential
from keras.layers import GlobalAveragePooling2D
model = Sequential()
model.add(GlobalAveragePooling2D(input_shape=(3,3,3)))
In this example, the GlobalAveragePooling2D()
layer calculates the average of each 3x3 feature map, resulting in a 1D tensor with three elements.
The Key Differences
The main difference between Flatten()
and GlobalAveragePooling2D()
lies in their operation and the resulting output size.
Operation:
Flatten()
reshapes the tensor by combining all dimensions except the batch size into one. On the other hand,GlobalAveragePooling2D()
performs an average pooling operation, reducing the spatial dimensions.Output Size:
Flatten()
results in a larger output size as it combines all elements into a single dimension.GlobalAveragePooling2D()
, however, significantly reduces the output size by averaging each feature map.
When to Use Flatten()
vs GlobalAveragePooling2D()
?
The choice between Flatten()
and GlobalAveragePooling2D()
depends on your specific use case and the architecture of your neural network.
Flatten(): Use this when you want to maintain all the information from your feature maps and connect your convolutional layers to fully connected layers. However, be aware that this can lead to a large number of parameters, potentially causing overfitting.
GlobalAveragePooling2D(): Use this when you want to reduce the dimensionality of your feature maps drastically. It’s commonly used in modern CNN architectures like ResNet and Inception, where it helps to prevent overfitting and reduces computational cost.
Common Errors and How to Handle Them
Error 1: Shape Mismatch
One common error occurs when the shape of the input is not compatible with the Flatten() or GlobalAveragePooling2D() layer. Ensure that the input shape matches the layer requirements.
Error 2: Negative Dimension Size
When dealing with convolutional layers preceding Flatten(), negative dimension size errors may occur. Address this by checking the output shape of the convolutional layers.
Conclusion
Understanding the difference between Flatten()
and GlobalAveragePooling2D()
is crucial when working with CNNs in Keras. While Flatten()
reshapes your tensor into a 1D vector, GlobalAveragePooling2D()
performs an average pooling operation, reducing the size of your tensor. The choice between the two depends on your specific use case and the architecture of your neural network.
Remember, the key to building effective neural networks is understanding how different layers and functions impact your model’s performance. So, experiment with both Flatten()
and GlobalAveragePooling2D()
to see which works best for your model.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.