Solving the 'DataFrame Object Has No Attribute 'name' Error in Pandas
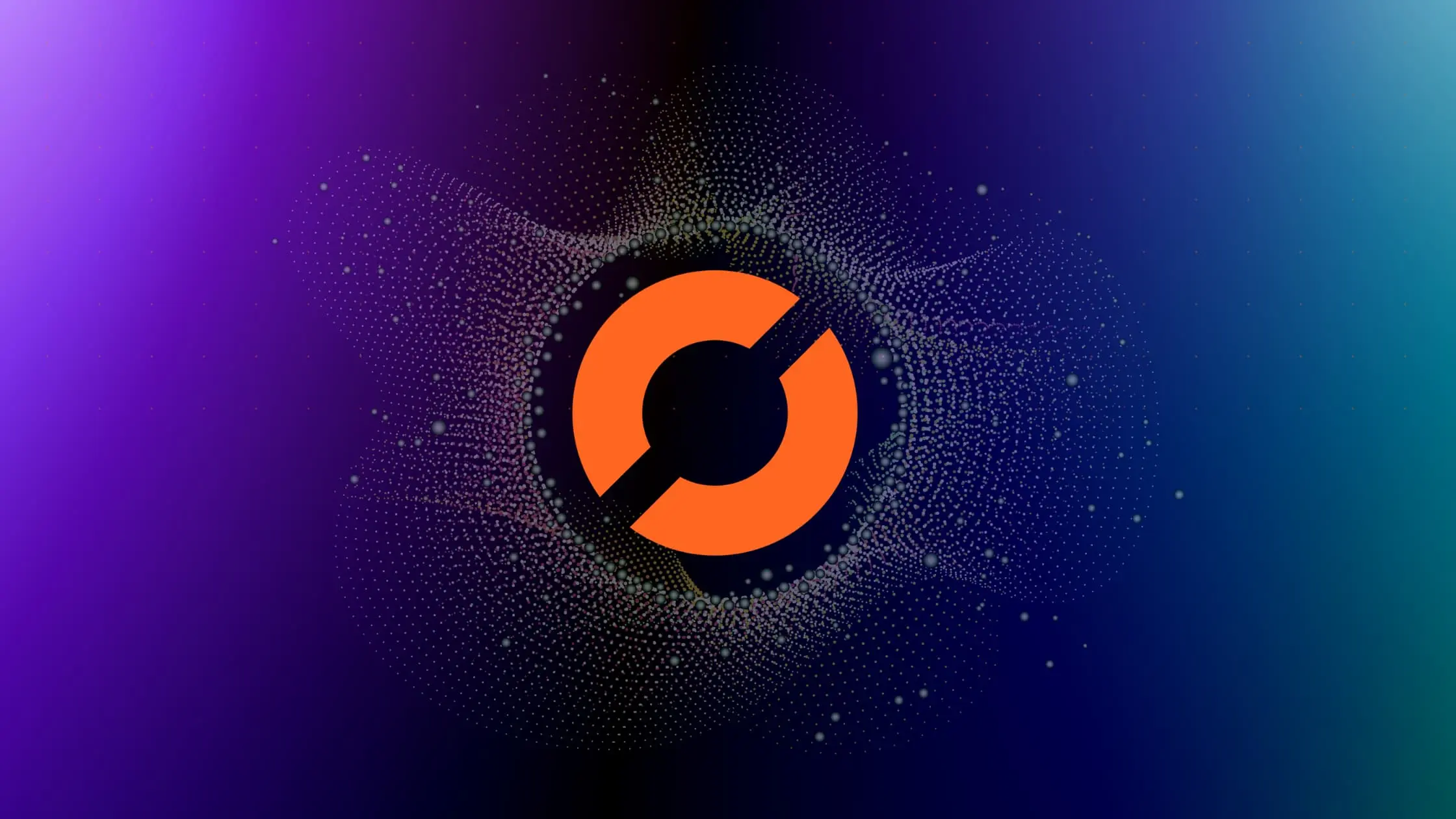
Solving the ‘DataFrame Object Has No Attribute ‘name’’ Error in Pandas
Pandas is a powerful data manipulation library in Python, widely used by data scientists and analysts. However, it’s not uncommon to encounter errors while working with it. One such error is the ‘DataFrame object has no attribute ‘name’’ error. This blog post will guide you through understanding and resolving this error.
Understanding the Error
Before we delve into the solution, let’s understand the error. The 'DataFrame' object has no attribute 'name'
error typically occurs when you try to access a DataFrame’s 'name'
attribute, which doesn’t exist.
import pandas as pd
# Create a dataframe with 2 columns named 'A' and 'B'
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df.name)
Running this code will result in an AttributeError: 'DataFrame' object has no attribute 'name'
. This is because a DataFrame as a whole does not have a 'name'
attribute.
The name
Attribute in Pandas
In Pandas, the name
attribute is associated with Series objects, not DataFrame objects. A Series object represents a single column or row in a DataFrame, and its name
attribute corresponds to the column or row label.
series = df['A']
print(series.name)
Output
A
Resolving the Error
Now that we understand the error and the 'name'
attribute, let’s look at how to resolve this error.
Accessing Column Names
If you want to access the names of all columns in a DataFrame, you can use the columns
attribute.
print(df.columns)
Output:
Index(['A', 'B'], dtype='object')
This will output Index(['A', 'B'], dtype='object')
, which is a list of all column names.
Accessing a Series’s Name
If you want to access the name of a specific Series (column or row), you can do so by first selecting the Series and then accessing its ‘name’ attribute.
series = df['A']
print(series.name)
Output
A
Renaming a Series
If you want to rename a Series, you can use the rename
method.
series = df['A'].rename('new_name')
print(series.name)
Output
new_name
Conclusion
The DataFrame object has no attribute 'name'
error in Pandas is a common error that can be easily resolved once you understand that the ‘name’ attribute is associated with Series objects, not DataFrame objects. By using the columns
attribute and the rename
method, you can effectively work with column and row names in your DataFrame.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.