Shipping Virtual Environments with PySpark: A Guide
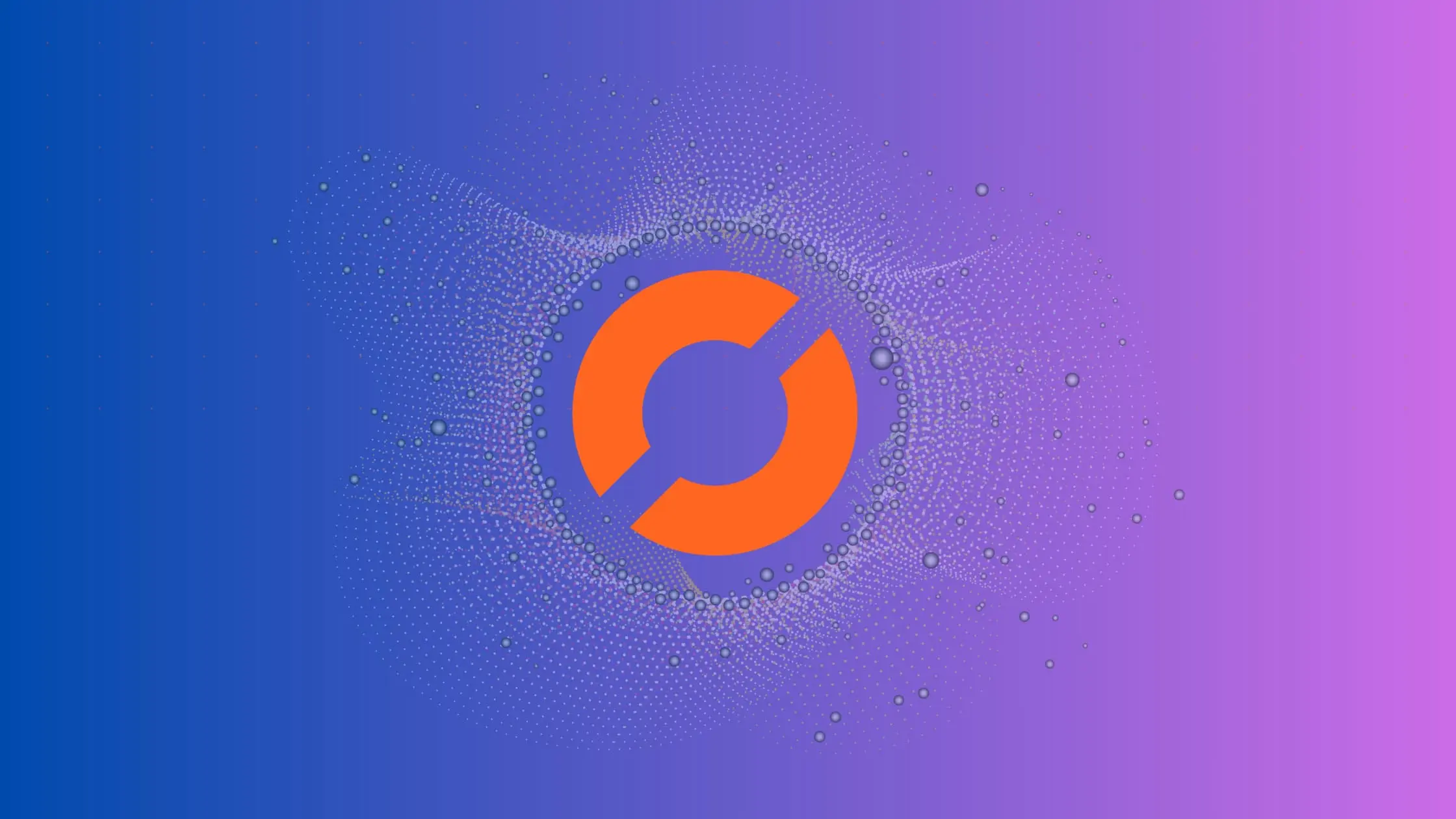
Shipping Virtual Environments with PySpark: A Guide
PySpark, the Python library for Apache Spark, is a powerful tool for data scientists. It allows for distributed data processing, which is essential for handling large datasets. However, one challenge that often arises is shipping virtual environments with PySpark. This blog post will guide you through the process, ensuring your PySpark applications run seamlessly across different environments.
Why Ship Virtual Environments with PySpark?
Before diving into the how, let’s understand the why. When working with PySpark, you may need to use specific Python libraries that aren’t available in the default Python environment on the Spark cluster. By shipping a virtual environment, you can ensure that your PySpark application has access to the necessary libraries, regardless of the cluster’s default environment.
Prerequisites
Before you embark on the journey of shipping virtual environments with PySpark, there are a few prerequisites you should meet to ensure a smooth and successful experience. Make sure you have the following in place:
Python and PySpark Installed: To work with PySpark, you must have Python and PySpark installed on your local machine or the cluster where you intend to run your PySpark applications. You can follow the official installation guides for Python and PySpark to set them up properly.
Apache Spark Installed: You should have Apache Spark installed on the machine from which you intend to submit your Spark applications using the spark-submit command. Ensure that Spark is correctly configured and accessible.
Basic Understanding of PySpark: Familiarize yourself with the basics of PySpark, including its architecture, RDDs, DataFrames, and how to create and run PySpark applications. This knowledge is crucial for effectively using PySpark in conjunction with virtual environments.
Basic Cluster Configuration: For a Spark cluster to function correctly, several cluster configuration parameters need to be set. Here are some of the basic configurations that should be considered:
Core-site.xml:
<configuration>
<property>
<name>fs.defaultFS</name>
<value>hdfs://localhost:9000</value>
</property>
</configuration>
HDFS-site.xml or HTTPS-site.xml:
<configuration>
<property>
<name>dfs.replication</name>
<value>1</value>
</property>
<property>
<name>dfs.namenode.name.dir</name>
<value>\your\hadoop\data\namenode\location</value>
</property>
<property>
<name>dfs.datanode.data.dir</name>
<value>\your\hadoop\data\datanode\location</value>
</property>
</configuration>
Mapred-site.xml:
<configuration>
<property>
<name>mapreduce.framework.name</name>
<value>yarn</value>
</property>
</configuration>
Yarn-site.xml:
<configuration>
<property>
<name>yarn.nodemanager.aux-services</name>
<value>mapreduce_shuffle</value>
</property>
<property>
<name>yarn.nodemanager.auxservices.mapreduce.shuffle.class</name>
<value>org.apache.hadoop.mapred.ShuffleHandler</value>
</property>
</configuration>
- Starting HDFS and YARN Services:" Running
start-dfs.sh
andstart-yarn.sh
commands is necessary to start the Hadoop Distributed File System (HDFS) and YARN ResourceManager before you submit Spark jobs in a Hadoop cluster. These commands ensure that the Hadoop services are up and running, which are essential for Spark to run successfully. Make sure you follow these steps:
Start HDFS:
start-dfs.sh
This command will start the HDFS daemons, including the NameNode and DataNode.
Start YARN:
start-yarn.sh
This command will start the YARN ResourceManager and NodeManagers.
After running these commands, it’s important to verify that all the services are running correctly. You can check the status of your Hadoop services by accessing the Hadoop ResourceManager and NameNode web interfaces. You can access these interfaces in a web browser:
ResourceManager: http://localhost:8088 NameNode: http://localhost:9870 Replace localhost with the actual hostname or IP address of your cluster if necessary.
Access to a Spark Cluster: You should have access to a Spark cluster where you intend to deploy your PySpark applications. This might be a local Spark cluster for testing or a remote cluster for production use. Ensure you have the necessary permissions and access rights.
Pip Installed: Ensure that the pip package manager is installed in your Python environment. This is required to install libraries within your virtual environment.
Java and Hadoop on the Spark Cluster: Java and Hadoop are essential components for running Spark on the Spark cluster itself. You, as the PySpark user, do not need to have Java and Hadoop installed on your local machine. The administrators of the Spark cluster are responsible for ensuring that Java and Hadoop are properly set up on the cluster where your PySpark job will execute.
Understanding of Virtual Environments: It’s essential to have a basic understanding of virtual environments in Python. If you are new to this concept, you can refer to the official Python documentation or external resources for a quick primer.
With these prerequisites in place, you’ll be well-prepared to create and ship virtual environments with PySpark, allowing your applications to run seamlessly with the required libraries across various environments. If you have any doubts or questions about these prerequisites, take the time to address them before proceeding with the steps outlined in this guide.
Now, let’s move on to the process of shipping virtual environments with PySpark.
Step 1: Create a Virtual Environment
First, you need to create a virtual environment. You can do this using venv
, a module provided by Python to create isolated Python environments. Here’s how:
python3 -m venv my_env
This command creates a new virtual environment named my_env
.
Step 2: Install Necessary Libraries
Next, activate the virtual environment and install the necessary libraries. For example, if your PySpark application requires numpy
and pandas
, you would do the following:
source my_env/bin/activate
pip install numpy pandas
Step 3: Package the Virtual Environment
Once you’ve installed the necessary libraries, you need to package the virtual environment. This can be done using the zip
command:
cd my_env
zip -r my_env.zip .
This creates a zip file of your virtual environment, which can be shipped with your PySpark application.
Step 4: Ship the Virtual Environment with PySpark
Now, you’re ready to ship the virtual environment with your PySpark application. You can do this using the --archives
option of the spark-submit
command:
spark-submit --master yarn --deploy-mode cluster --archives my_env.zip#env my_script.py
In this command, my_env.zip
is the zip file of your virtual environment, and my_script.py
is your PySpark script. The #env
part is an alias for the virtual environment, which you can use in your PySpark script to refer to the virtual environment.
Execution Time and Resource Dependency
It’s important to understand that the execution time of PySpark applications is closely tied to the resources available in your Spark cluster. The resources include the number of worker nodes, the amount of memory allocated to each node, the CPU cores, and network bandwidth.
Cluster Size: Larger clusters with more worker nodes generally provide better parallelism and can process larger datasets more quickly. However, setting up and maintaining larger clusters can be more complex and costly.
Resource Allocation: The allocation of resources to your PySpark applications is a critical factor. The amount of memory, the number of CPU cores, and the level of parallelism allocated to your tasks can significantly impact execution time.
Data Distribution: The distribution of data across the cluster also affects execution time. If data is skewed or not evenly distributed, it can lead to longer execution times.
Complexity of the Task: The nature of the PySpark job itself plays a role. Complex transformations and actions may take longer to execute compared to simpler operations.
Optimizations: The use of Spark’s built-in optimizations, such as data caching and broadcast joins, can improve performance and reduce execution time.
Therefore, when working with PySpark, it’s essential to consider the cluster’s available resources and optimize your applications accordingly. Keep in mind that a well-configured and appropriately scaled Spark cluster can significantly reduce execution times and improve the efficiency of your data processing tasks.
You can specify the number of executors, executor memory, and executor CPU cores using the --num-executors
, --executor-memory
, and --executor-cores
options, respectively. For example:
spark-submit \
--master yarn \
--deploy-mode cluster \
--num-executors 5 \
--executor-memory 2g \
--executor-cores 2 \
my_script.py
Hadoop YARN provides web interfaces that allow you to monitor the status and resource utilization of your cluster on your local machine.
ResourceManager Web UI:
The ResourceManager Web UI is the central resource management and job scheduling page for your YARN cluster. To access the ResourceManager Web UI, open a web browser and go to: http://localhost:8088 This page provides an overview of the cluster’s status, including the number of applications submitted, their states (such as NEW, RUNNING, FINISHED), and resource allocation metrics.
Step 5: Use the Virtual Environment in Your PySpark Script
Finally, in your PySpark script, you need to activate the virtual environment before importing any libraries. Here’s how:
import os
import sys
# Activate the virtual environment
activate_env = os.path.expanduser("~/env/bin/activate_this.py")
exec(open(activate_env).read(), dict(__file__=activate_env))
# Now you can import any library installed in the virtual environment
import numpy as np
import pandas as pd
And that’s it! You’ve successfully shipped a virtual environment with your PySpark application.
Conclusion
Shipping virtual environments with PySpark is a powerful technique that allows for greater flexibility and control over the Python environment in which your PySpark applications run. By following the steps outlined in this blog post, you can ensure that your PySpark applications have access to the necessary Python libraries, regardless of the default Python environment on the Spark cluster.
Remember, the key to successful data science is not just about having the right tools, but also about knowing how to use them effectively. So, keep exploring, keep learning, and keep pushing the boundaries of what’s possible with PySpark.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.