Scatter Plot Labels in One Line - Matplotlib
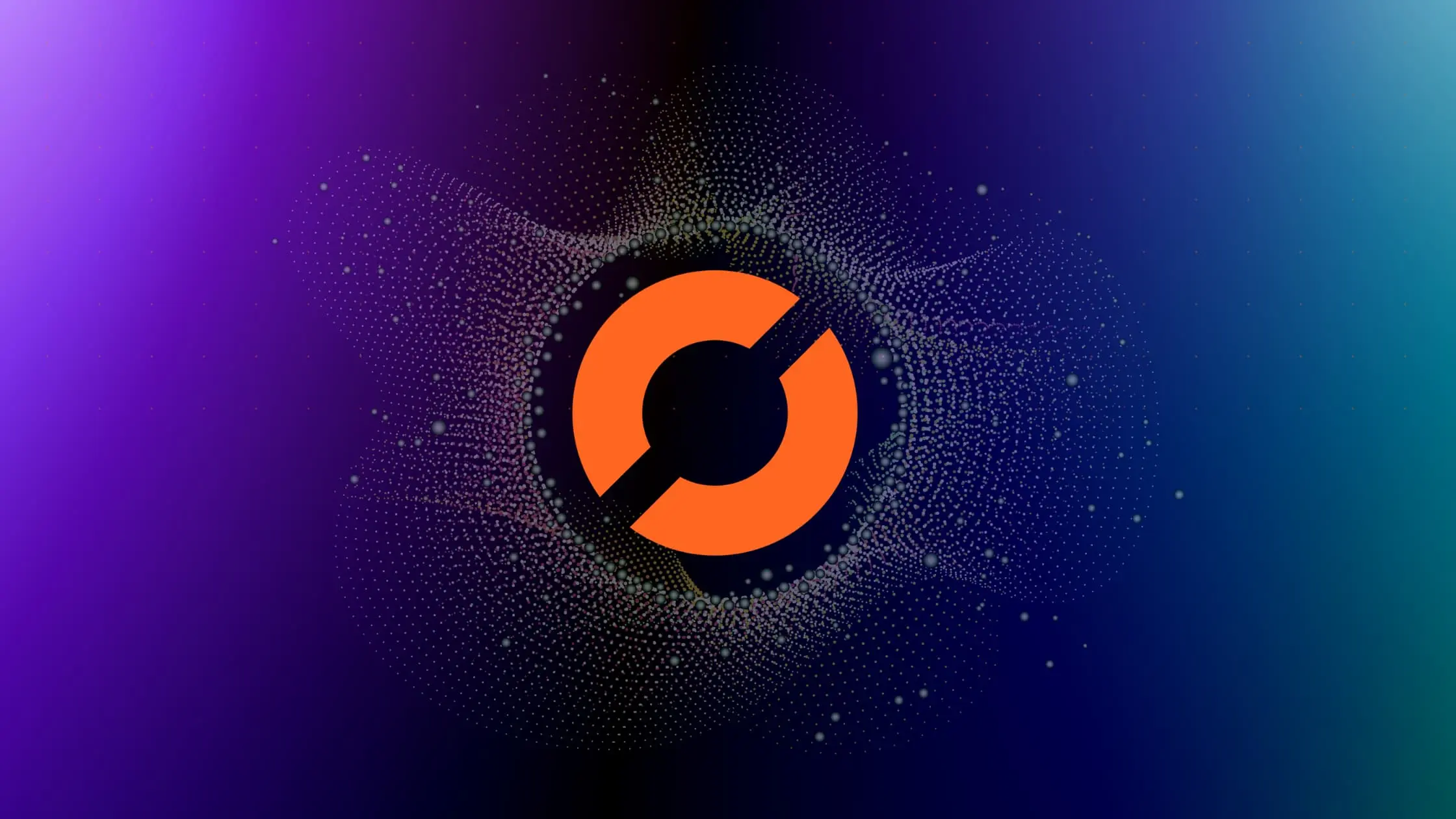
Why Scatter Plots?
Scatter plots are a staple in the data scientist’s toolkit. They allow us to visualize the relationship between two variables, making it easier to identify trends, correlations, and outliers. However, when dealing with large datasets, labeling each point in the scatter plot can become a challenge. That’s where Matplotlib’s one-line labeling comes in handy.
Setting Up Your Environment
Before we dive in, make sure you have the necessary tools installed. You’ll need Python (preferably 3.6 or later) and Matplotlib. If you haven’t installed Matplotlib yet, you can do so using pip:
pip install matplotlib
Creating a Scatter Plot
Let’s start by creating a simple scatter plot. We’ll use the pyplot
module from Matplotlib, which provides a MATLAB-like interface for plotting.
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create scatter plot
plt.scatter(x, y)
plt.show()
This will create a scatter plot of our data. But what if we want to label each point?
Adding Labels in One Line
In Matplotlib, you can add labels to your scatter plot points using the text
function. However, doing this for each point can be tedious and make your code less readable. Instead, we can use a one-liner to label all points.
# Label points
for (i, j) in zip(x, y):
plt.text(i, j, f'({i}, {j})')
This will add labels to each point in the format (x, y)
. But we can make this even more concise with a list comprehension.
# Label points in one line
[plt.text(i, j, f'({i}, {j})') for (i, j) in zip(x, y)]
And there you have it! All your scatter plot points are labeled in just one line of code.
Customizing Your Labels
Matplotlib offers a variety of options to customize your labels. You can adjust the font size, color, and position to make your plot more readable.
# Label points with customizations
[plt.text(i, j, f'({i}, {j})', fontsize=8, ha='right') for (i, j) in zip(x, y)]
In this example, we’ve set the font size to 8 and aligned the labels to the right of the points.
Conclusion
Scatter plots are an essential tool for data visualization, and Matplotlib makes it easy to create and customize them. With the one-line labeling technique, you can make your code more concise and readable. So next time you’re working with scatter plots, give this method a try!
Remember, effective data visualization is not just about presenting data; it’s about telling a story. With Matplotlib’s powerful features, you can tell your data’s story more effectively.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.