Resolving 'ValueError: If using all scalar values, you must pass an index' When Merging Multiple DataFrames
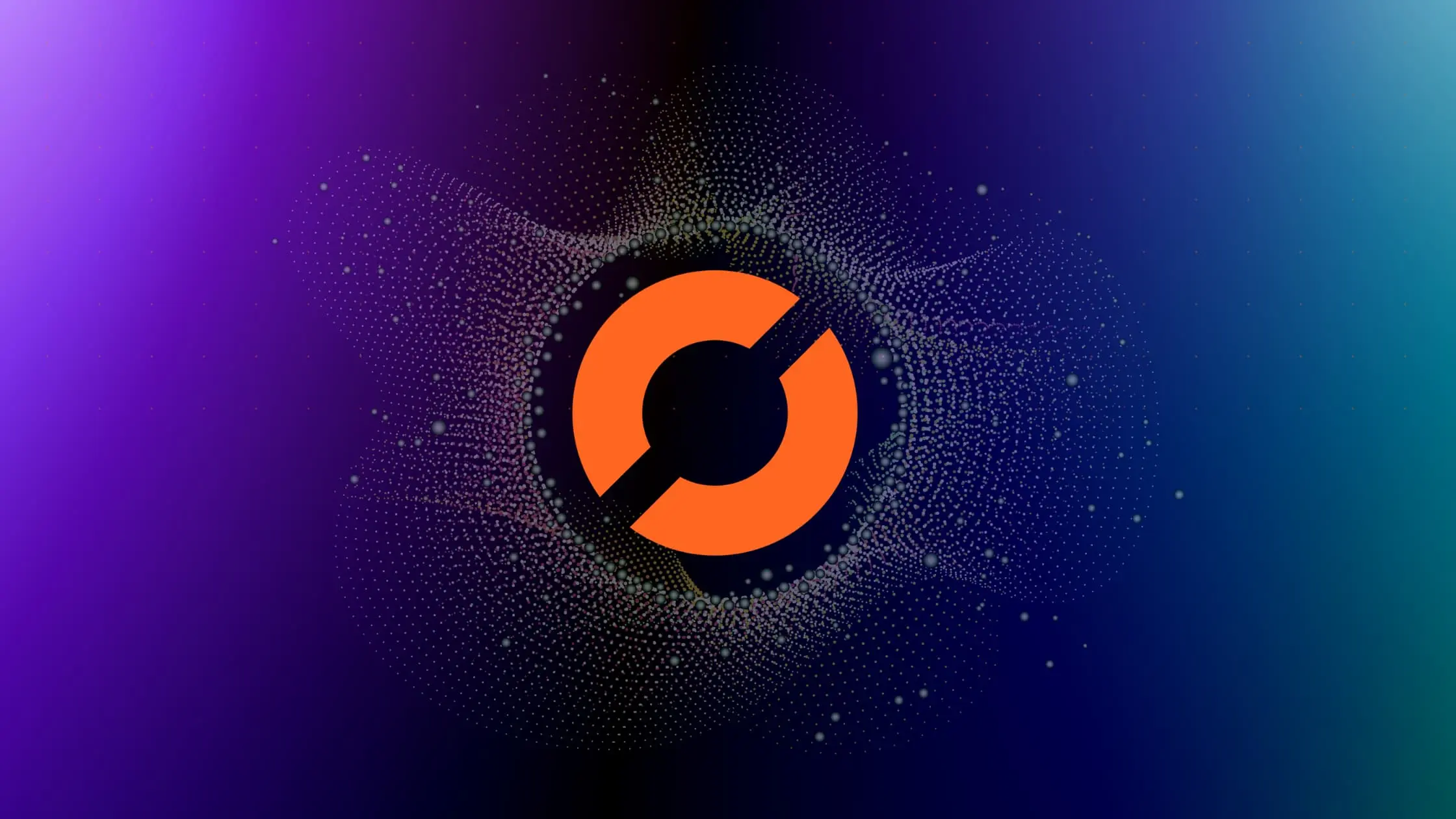
When working with large datasets, data scientists often need to merge multiple dataframes. However, this process can sometimes lead to errors, one of which is the "ValueError: If using all scalar values, you must pass an index"
. This blog post will guide you through the steps to resolve this error, ensuring a smooth and efficient data merging process.
Table of Contents
Understanding the Error
Before we dive into the solution, let’s understand the error. The "ValueError: If using all scalar values, you must pass an index"
typically occurs when you’re trying to create a DataFrame from scalar values without providing an index. In pandas, scalar values are single values like integers, floats, or strings. When you’re creating a DataFrame from these values, pandas needs an index to structure the data.
The Solution
import pandas as pd
# Scalar values
data = {'column1': 1, 'column2': 'value'}
# Create DataFrame without index
df = pd.DataFrame(data)
Output:
ValueError: If using all scalar values, you must pass an index
There are several solutions to this error.
Passing an index to the DataFrame
import pandas as pd
# Scalar values
data = {'column1': 1, 'column2': 'value'}
# Create DataFrame with index
df = pd.DataFrame(data, index=[0])
print(df)
Output:
column1 column2
0 1 value
In the above code, we’re creating a DataFrame from a dictionary of scalar values. By passing index=[0]
, we’re providing pandas with the necessary index.
Using lists instead of scalar values.
import pandas as pd
# Scalar values
data = {'column1': [1], 'column2': ['value']}
# Create DataFrame with index
df = pd.DataFrame(data)
print(df)
Output:
column1 column2
0 1 value
Using pd.DataFrame.from_records()
import pandas as pd
# Scalar values
data = {'column1': [1], 'column2': ['value']}
# Create DataFrame with index
df = pd.DataFrame.from_records(data)
print(df)
Output:
column1 column2
0 1 value
Using pd.DataFrame.from_dict()
import pandas as pd
# Scalar values
data = {'column1': [1], 'column2': ['value']}
# Create DataFrame with index
df = pd.DataFrame.from_dict(data)
print(df)
Output:
column1 column2
0 1 value
Merging Multiple DataFrames
Now that we’ve resolved the error, let’s look at how to merge multiple dataframes. Merging is a crucial operation in pandas that combines two or more dataframes into a single one based on a common column (or ‘key’).
Here’s a simple example:
# Create two dataframes
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']},
index=[0, 1, 2])
df2 = pd.DataFrame({'C': ['C0', 'C1', 'C2'],
'D': ['D0', 'D1', 'D2']},
index=[0, 1, 2])
# Merge dataframes
df = pd.merge(df1, df2, left_index=True, right_index=True)
print(df)
Output:
A B C D
0 A0 B0 C0 D0
1 A1 B1 C1 D1
2 A2 B2 C2 D2
In this example, we’re merging df1
and df2
based on their index. The left_index=True
and right_index=True
parameters tell pandas to use the index of the dataframes as the key for merging.
Conclusion
Merging multiple dataframes is a common operation in data science, but it can sometimes lead to errors if not done correctly. The "ValueError: If using all scalar values, you must pass an index"
is one such error that occurs when creating a DataFrame from scalar values without an index. The solution is to pass an index when creating the DataFrame.
Remember, understanding the errors and knowing how to resolve them is just as important as knowing how to write the code. It not only helps you write better and more efficient code, but it also makes you a better data scientist.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.