Reading Nested JSON Files in PySpark: A Guide
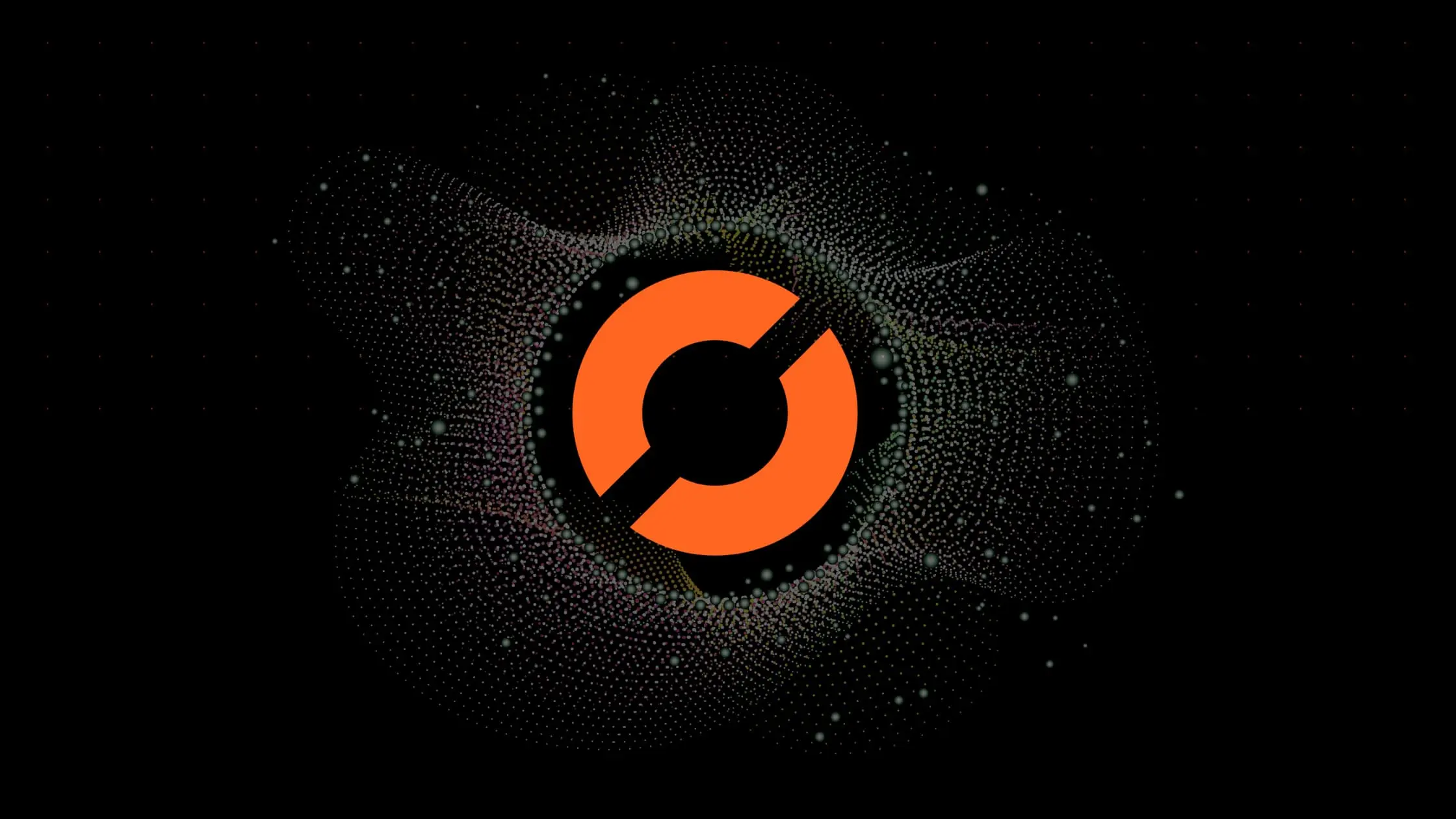
In the world of big data, JSON (JavaScript Object Notation) has become a popular format for data interchange due to its simplicity and readability. However, when dealing with nested JSON files, data scientists often face challenges. This blog post aims to guide you through reading nested JSON files using PySpark, a Python library for Apache Spark.
Table of Contents
Introduction to PySpark
PySpark is a Python API for Apache Spark, a powerful open-source data processing engine. It is designed to handle large datasets through distributed computing. PySpark provides an easy-to-use interface for big data processing, making it a go-to tool for data scientists.
Prerequisites Before diving into PySpark, it’s essential to set up your environment properly. Key prerequisites include:
- Java: Apache Spark runs on the Java Virtual Machine (JVM), so having Java installed is critical. Java 8 or later versions are recommended.
- Hadoop: Although not always necessary, Spark can leverage Hadoop’s ecosystem, particularly for HDFS storage and YARN resource management.
- Spark Configuration: Ensure that your Spark installation is configured correctly to interact with the necessary resources and cluster management systems.
Understanding Nested JSON Files
A nested JSON file contains other JSON structures like arrays or objects within it. This nesting can occur multiple levels deep, making the data extraction process complex. Here’s an example of a nested JSON:
{
"name": "John",
"age": 30,
"cars": [
{"car1": "Ford", "model": "Mustang"},
{"car2": "BMW", "model": "X5"}
]
}
Reading Nested JSON Files in PySpark
Let’s dive into the process of reading nested JSON files using PySpark.
Step 1: Importing Necessary Libraries
First, we need to import the necessary PySpark libraries.
from pyspark.sql import SparkSession
from pyspark.sql.functions import explode
Step 2: Creating a Spark Session
Next, we create a Spark session, which is the entry point to any Spark functionality.
spark = SparkSession.builder.appName('nestedJSON').getOrCreate()
Step 3: Loading the JSON File
Now, we load the JSON file using the read.json
function.
df = spark.read.json('path_to_your_json_file')
Step 4: Exploring the Data
To understand the structure of our data, we use the printSchema()
function.
df.printSchema()
Output:
root
|-- age: long (nullable = true)
|-- cars: array (nullable = true)
| |-- element: struct (containsNull = true)
| | |-- car1: string (nullable = true)
| | |-- car2: string (nullable = true)
| | |-- model: string (nullable = true)
|-- name: string (nullable = true)
Step 5: Flattening the JSON File
To flatten the nested JSON file, we use the explode
function. This function creates a new row for each element in the given array or map column.
df_flat = df.select('name', 'age', explode('cars').alias('car_info'))
df_flat = df_flat.select('name', 'age', 'car_info.car1', 'car_info.model')
df_flat.show()
Output:
+----+---+----+-------+
|name|age|car1| model|
+----+---+----+-------+
|John| 30|Ford|Mustang|
|John| 30|NULL| X5|
+----+---+----+-------+
Conclusion
Reading nested JSON files in PySpark can be a bit tricky, but with the right approach, it becomes straightforward. By understanding the structure of your data and using PySpark’s powerful functions, you can easily extract and analyze data from nested JSON files.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.