Random Row Selection in Pandas Dataframe
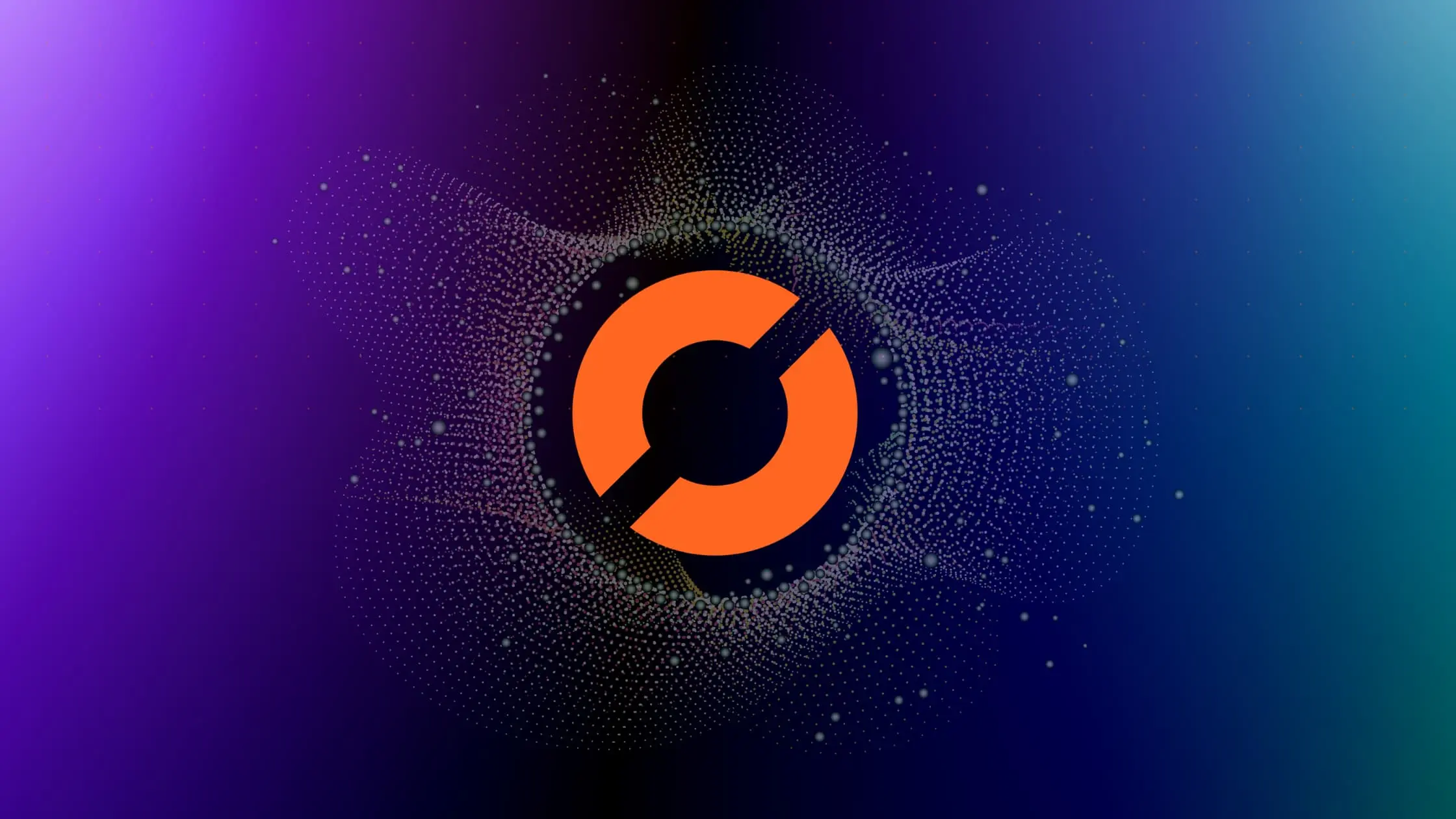
As a data scientist, you may frequently encounter scenarios where you need to randomly select rows from a Pandas dataframe. This can be useful for tasks such as data exploration, sampling, and testing. In this blog post, we will explore different ways to perform random row selection in a Pandas dataframe.
Table of Contents
Random Row Selection with Pandas 2.1 Method 1: Using the
sample
Method 2.1.1 Example 2.2 Method 2: Using therandom
Method 2.2.1 Example 2.3 Method 3: Using thenumpy
Module 2.3.1 ExampleBest Practices 3.1 Seed for Reproducibility 3.2 Stratified Sampling 3.3 Weighted Sampling 3.4 Efficiently Selecting a Subset of Columns 3.5 Handling Large Datasets 3.6 Error Handling for Small Datasets 3.7 Testing Performance
What is Pandas?
Before diving into the topic of random row selection, let us briefly introduce Pandas. Pandas is a powerful Python library for data manipulation and analysis. It provides data structures and functions for handling tabular data, such as dataframes, which are similar to tables in a relational database.
Pandas is widely used in data science and machine learning workflows due to its ease of use, flexibility, and performance. It allows you to load, transform, and analyze data from various sources, such as CSV files, SQL databases, and web APIs.
Random Row Selection with Pandas
Now let’s get back to the main topic of this blog post, which is random row selection in a Pandas dataframe. There are several ways to perform this task, depending on your specific requirements.
Method 1: Using the sample Method
The simplest way to randomly select rows from a Pandas dataframe is by using the sample
method. This method returns a random sample of rows from the dataframe, based on the specified number or fraction of rows.
Here’s an example:
Name,Age,Score
John,25,80
Jane,30,92
Bob,22,78
Alice,28,85
Charlie,35,90
David,32,88
Eva,27,95
Frank,24,82
Grace,29,89
Hank,26,91
import pandas as pd
# Load a sample dataframe
df = pd.read_csv('data.csv')
# Select 10 random rows
sample_df = df.sample(n=10)
print(sample_df)
Output:
Name Age Score
8 Grace 29 89
7 Frank 24 82
4 Charlie 35 90
0 John 25 80
2 Bob 22 78
6 Eva 27 95
1 Jane 30 92
9 Hank 26 91
3 Alice 28 85
5 David 32 88
In this example, we load a sample dataframe from a CSV file and then use the sample
method to select 10 random rows. The n
parameter specifies the number of rows to select. You can also use the frac
parameter to specify the fraction of rows to select, for example frac=0.1
to select 10% of the rows.
Method 2: Using the random Method
Another way to perform random row selection in Pandas is by using the random
method. This method returns a random integer between 0 and the number of rows in the dataframe, which can be used as an index to select a random row.
Here’s an example:
import pandas as pd
import random
# Load a sample dataframe
df = pd.read_csv('data.csv')
# Select a random row
random_index = random.randint(0, len(df)-1)
random_row = df.iloc[random_index]
print(random_row)
Output:
Name Hank
Age 26
Score 91
Name: 9, dtype: object
In this example, we load a sample dataframe from a CSV file and then use the random
method to generate a random index between 0 and the number of rows in the dataframe. We then use the iloc
method to select the row at the random index.
Method 3: Using the numpy Module
Finally, you can also use the numpy
module to perform random row selection in Pandas. This method involves generating a random array of indices and then using the iloc
method to select the corresponding rows from the dataframe.
Here’s an example:
import pandas as pd
import numpy as np
# Load a sample dataframe
df = pd.read_csv('data.csv')
# Generate an array of random indices
random_indices = np.random.randint(0, len(df), size=10)
# Select the corresponding rows
random_rows = df.iloc[random_indices]
print(random_rows)
Output:
Name Age Score
3 Alice 28 85
8 Grace 29 89
0 John 25 80
6 Eva 27 95
4 Charlie 35 90
7 Frank 24 82
4 Charlie 35 90
2 Bob 22 78
6 Eva 27 95
7 Frank 24 82
In this example, we load a sample dataframe from a CSV file and then use the numpy
module to generate an array of 10 random indices between 0 and the number of rows in the dataframe. We then use the iloc
method to select the corresponding rows from the dataframe.
Best Practices
- Seed for Reproducibility: When using methods like
sample
ornp.random.randint
, consider setting a seed using therandom_state
parameter. This ensures reproducibility, meaning if someone else runs the same code with the same seed, they will get the same random rows.
# Set a seed for reproducibility
random.seed(42)
np.random.seed(42)
- Stratified Sampling: If your dataset has a target variable and you want to maintain the distribution of classes, consider using the
stratify
parameter in thesample
method.
# Stratified sampling based on a categorical column 'target'
sample_df = df.sample(n=10, stratify=df['target'])
- Weighted Sampling: If your dataset has a weight column, you can perform weighted random sampling. The probability of selecting a row is proportional to its weight.
# Weighted random sampling based on a 'weight' column
sample_df = df.sample(n=10, weights=df['weight'])
- Efficiently Selecting a Subset of Columns: If you are working with a large dataframe and need only a subset of columns, use the loc method for efficient selection.
# Selecting 10 random rows and specific columns
random_rows = df.sample(n=10).loc[:, ['column1', 'column2']]
Handling Large Datasets: For large datasets, consider using methods like Dask for distributed computing, which can handle larger-than-memory computations efficiently.
Error Handling for Small Datasets: Add error handling when attempting to select more rows than the total number of rows in the dataframe to avoid unexpected behavior.
# Add error handling for small datasets
if n > len(df):
raise ValueError("Number of rows to select is greater than the total number of rows in the dataframe.")
- Testing Performance: When working with large datasets, consider testing the performance of different methods using the
%timeit
magic command in Jupyter notebooks to identify the most efficient approach.
%timeit df.sample(n=10)
%timeit df.iloc[np.random.randint(0, len(df), size=10)]
Conclusion
Random row selection is a common task in data science and machine learning workflows. In this blog post, we explored different ways to perform random row selection in a Pandas dataframe, including using the sample
method, the random
method, and the numpy
module.
By using these methods, you can easily select random rows from a Pandas dataframe for tasks such as data exploration, sampling, and testing. Experiment with different methods and parameters to find the one that best suits your specific requirements.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.