PyTorch Tensor Indexing: A Guide
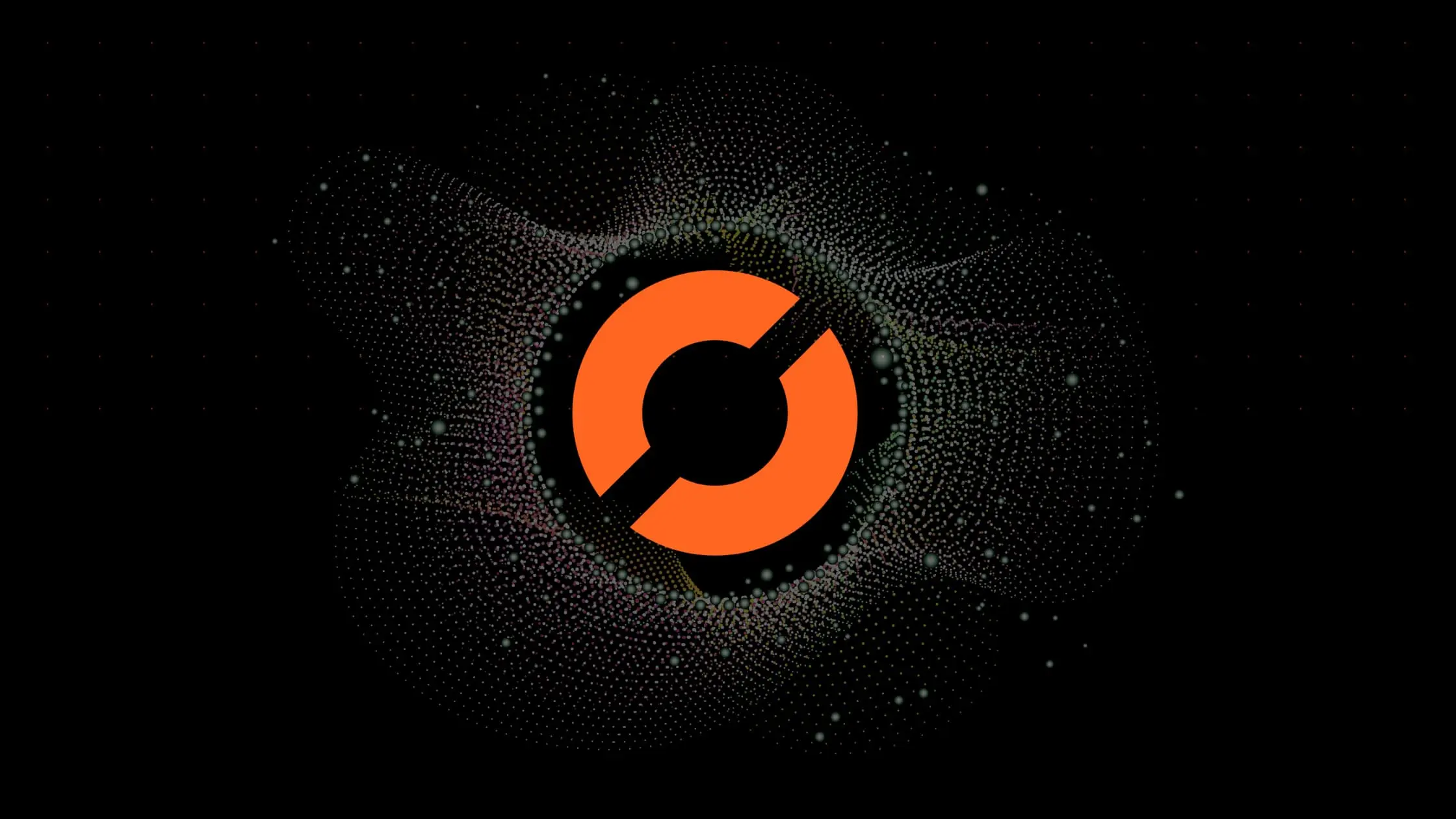
As a data scientist or software engineer, you may often work with large datasets and complex mathematical operations that require efficient and scalable computing. PyTorch is a popular open-source machine learning library that offers fast and flexible tensor computation with GPU acceleration. In this article, we will dive deep into PyTorch tensor indexing, a powerful technique that allows you to select and manipulate specific elements or subsets of a tensor with ease.
Table of Contents
What is PyTorch Tensor Indexing?
Tensor indexing is the process of selecting specific elements or subsets of a tensor based on their positions or values. PyTorch tensor indexing provides a rich set of indexing operations that enable you to select and modify tensor elements using different indexing schemes, such as integer indexing, boolean indexing, and advanced indexing.
In PyTorch, a tensor is a multi-dimensional array that can store numerical data of different types and sizes. A tensor can be indexed using one or more indices that specify the position of the elements along each dimension of the tensor. Indexing a tensor returns a new tensor that contains the selected elements or a view of the original tensor with modified elements.
How to Index PyTorch Tensors?
Integer Indexing
Integer indexing is the most basic form of tensor indexing that allows you to select specific elements of a tensor using their integer position along each dimension. You can use integer indexing to select a single element or a sub-tensor by providing a list of integers that correspond to the indices of the desired elements.
import torch
# create a 2D tensor of size (3, 4)
x = torch.tensor([[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]])
# select the element at position (1, 2)
print(x[1, 2]) # output: tensor(7)
# select the sub-tensor of size (2, 3) starting at position (0, 1)
print(x[0:2, 1:4]) # output: tensor([[2, 3, 4], [6, 7, 8]])
Boolean Indexing
Boolean indexing allows you to select specific elements of a tensor based on a boolean condition. You can use boolean indexing to select elements that satisfy a certain condition or to mask out elements that do not satisfy the condition.
# create a 1D tensor of size 5
x = torch.tensor([1, 2, 3, 4, 5])
# select the elements that are greater than 3
print(x[x > 3]) # output: tensor([4, 5])
# mask out the elements that are not even
x[x % 2 != 0] = 0
print(x) # output: tensor([0, 2, 0, 4, 0])
Advanced Indexing
Advanced indexing allows you to select specific elements of a tensor using a combination of integer indexing, boolean indexing, and other advanced indexing schemes. You can use advanced indexing to select elements from multiple dimensions of a tensor or to create a new tensor with a different shape.
import torch
# create a 3D tensor of size (2, 3, 4)
x = torch.tensor([[[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]],
[[13, 14, 15, 16],
[17, 18, 19, 20],
[21, 22, 23, 24]]])
# select the elements at positions (0, 1, 2) and (1, 2, 3)
print(x[:, (0, 1, 2), (1, 2, 3)]) # output: tensor([[ 2, 7, 12],
# [14, 19, 24]])
# create a new tensor by selecting the elements that are greater than 10
y = x[x > 10]
print(y) # output: tensor([11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24])
Modifying Tensor Elements
PyTorch tensor indexing not only allows you to select tensor elements but also enables you to modify them in place or create a new tensor with modified elements. You can use indexing to assign new values to tensor elements or to apply mathematical operations to selected elements.
# create a 2D tensor of size (3, 3)
x = torch.tensor([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
# assign a new value to the element at position (1, 2)
x[1, 2] = 10
print(x) # output: tensor([[ 1, 2, 3], [ 4, 5, 10], [ 7, 8, 9]])
# multiply the elements that are greater than 5 by 2
x[x > 5] *= 2
print(x) # output: tensor([[ 1, 2, 3], [ 4, 5, 20], [14, 16, 18]])
Conclusion
PyTorch tensor indexing is a powerful and flexible technique that enables you to select and modify specific elements or subsets of a tensor using different indexing schemes. Whether you are working with large datasets or complex mathematical operations, PyTorch tensor indexing provides a simple and efficient way to manipulate tensor data. In this article, we covered the basics of PyTorch tensor indexing, including integer indexing, boolean indexing, advanced indexing, and modifying tensor elements. By mastering these indexing techniques, you can unlock the full potential of PyTorch for your data science and machine learning projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.