Python/Numpy/Scipy - Converting String to Mathematical Function
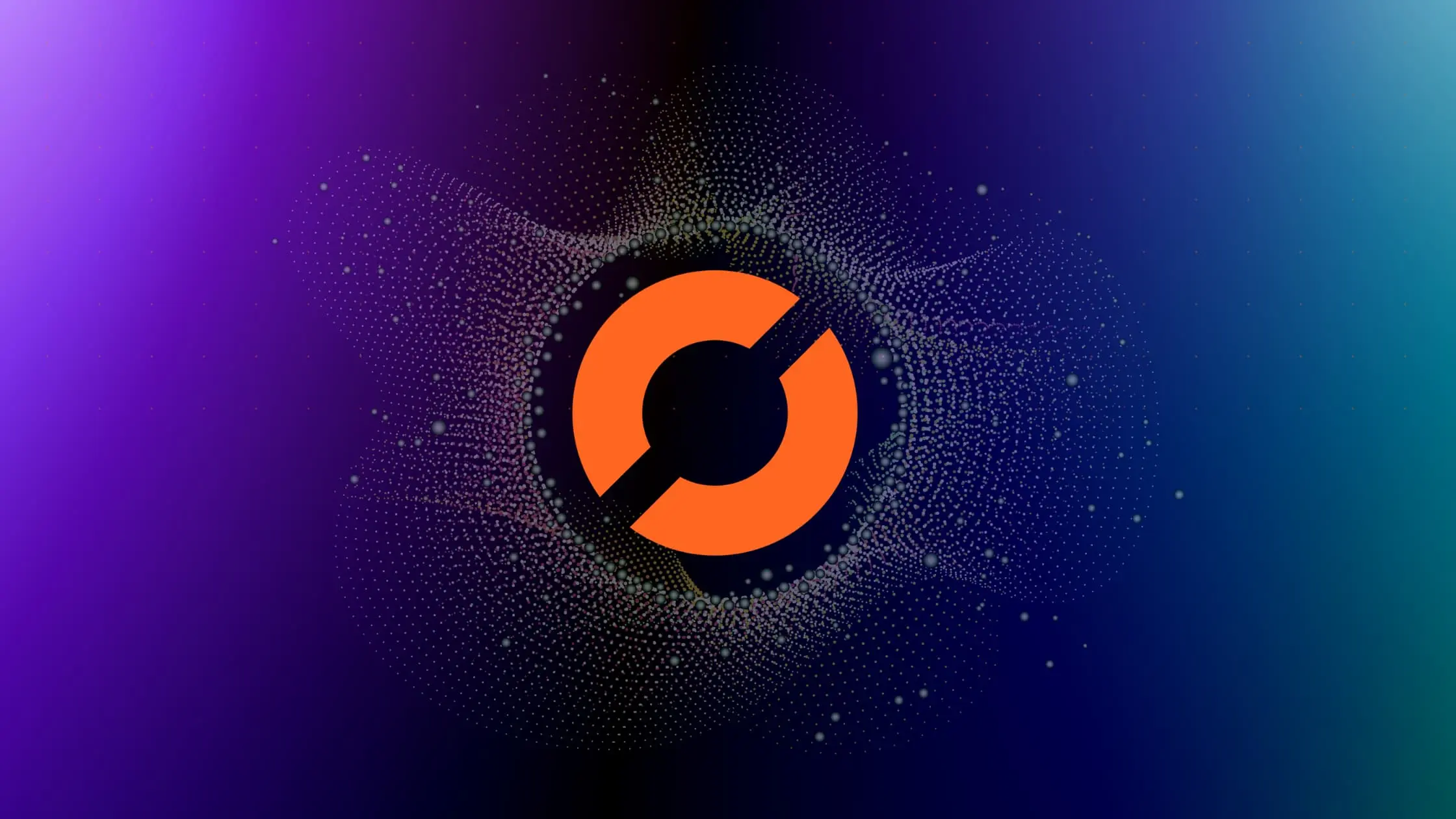
As data scientists, we often encounter scenarios where we need to convert a string representation of a mathematical function into an actual executable function. This process is crucial for tasks such as equation solving, optimization, and model fitting. In this blog post, we will explore how to convert a string into a mathematical function using the powerful Python libraries: NumPy and SciPy.
Table of Contents
- Why Convert a String to a Mathematical Function?
- The Eval Approach
- NumPy’s frompyfunc() Function
- SciPy’s sympify() Function
- Error Handling
- Conclusion
Why Convert a String to a Mathematical Function?
Before diving into the implementation details, let’s understand the motivation behind converting a string into a mathematical function. Imagine you have a mathematical expression in string format, such as "2 * x + 5"
. Converting this string into a function allows you to evaluate it for different values of x
without manually parsing and evaluating the expression each time.
By converting strings to functions, you can dynamically define and manipulate mathematical expressions during runtime. This flexibility is particularly useful when dealing with complex equations or when you need to experiment with different functions without changing your code.
The Eval Approach
One straightforward way to convert a string into a mathematical function is by using the eval()
function in Python. The eval()
function parses and evaluates a Python expression from a string, returning the result.
Let’s consider a simple example where we want to convert the string "x**2 + 3 * x - 1"
into a function:
def string_to_function(expression):
def function(x):
return eval(expression)
return function
my_function = string_to_function("x**2 + 3 * x - 1")
result = my_function(2)
print(result) # Output: 9
In this example, we define a helper function string_to_function()
that takes an expression as input and returns a function. This returned function accepts a single argument x
and evaluates the expression using eval()
.
Although the eval()
approach provides a quick solution, it is important to be cautious when using it. The eval()
function can execute arbitrary code, making it a potential security risk if you are accepting user input. Additionally, the eval()
approach might not be efficient for complex functions or large datasets.
Pros:
- Simplicity: The eval approach is simple and straightforward, making it easy to implement.
- Quick Solution: It provides a quick solution for converting simple mathematical expressions into functions.
Cons:
- Security Risk: Eval can execute arbitrary code, posing a security risk, especially when dealing with user input.
- Inefficiency: It may not be efficient for complex functions or when dealing with large datasets.
NumPy’s frompyfunc() Function
NumPy provides a powerful function called frompyfunc()
that allows us to create a universal function from an arbitrary Python function. This function can then be used to evaluate the function across arrays of input arguments.
To convert a string into a mathematical function using NumPy, we can combine the frompyfunc()
function with the eval()
approach:
import numpy as np
def string_to_function(expression):
def function(x):
return eval(expression)
return np.frompyfunc(function, 1, 1)
my_function = string_to_function("x**2 + 3 * x - 1")
x = np.array([1, 2, 3, 4, 5])
result = my_function(x)
print(result) # Output: [3 9 17 27 39]
In this example, we use np.frompyfunc()
to create a universal function from our custom function. The frompyfunc()
function takes three arguments: the function to be converted, the number of input arguments, and the number of output values. Here, we pass 1
for both input and output arguments since our function takes a single input x
and returns a single output.
By converting the string into a universal function, we can now evaluate it efficiently across arrays of input arguments using NumPy’s broadcasting capabilities.
Pros:
- Efficiency: Utilizes NumPy’s broadcasting capabilities, making it efficient for evaluating functions across arrays of input arguments.
- Integration with NumPy: Integrates well with NumPy, allowing for seamless use in numerical operations.
Cons:
- Limited Symbolic Capabilities: It primarily focuses on numerical evaluations and lacks the symbolic manipulation capabilities of SymPy.
SciPy’s sympify() Function
Another approach to convert a string into a mathematical function involves using the sympify()
function from the SymPy library, which is built on top of SciPy. SymPy provides a comprehensive set of tools for symbolic mathematics, including parsing and manipulating mathematical expressions.
Let’s see how we can use sympify()
to convert a string into a mathematical function:
from sympy import sympify, symbols
expression = "x**2 + 3 * x - 1"
x = symbols('x')
my_function = sympify(expression)
result = my_function.subs(x, 2)
print(result) # Output: 9
In this example, we start by defining the expression as a string. We then create a symbolic variable x
using the symbols()
function from SymPy. Finally, we use sympify()
to convert the expression into a symbolic expression, which can be evaluated using the subs()
method by substituting the value of x
.
The advantage of using SymPy is that it provides a more comprehensive and powerful toolkit for symbolic computations. It allows you to perform symbolic manipulations, solve equations, and differentiate functions, among other capabilities.
Pros:
- Symbolic Capabilities: SymPy provides symbolic computation capabilities, allowing for more advanced mathematical manipulations.
- Comprehensive Toolkit: Offers a comprehensive toolkit for symbolic mathematics, including equation solving and differentiation.
Cons:
- Overhead: May introduce additional overhead, especially if only numerical evaluations are needed.
- Learning Curve: Working with symbolic expressions might have a steeper learning curve for users unfamiliar with symbolic mathematics.
Error Handling:
Invalid Expression: Implement checks to validate the input expression for syntax errors or invalid mathematical expressions. Utilize try-except blocks to catch and handle potential errors.
Data Type Mismatch: Ensure that the data types of input values are compatible with the mathematical operations. Perform explicit type conversions if needed.
Security Concerns: Avoid using eval for untrusted input. Implement additional input validation and sanitize user inputs to prevent code injection attacks.
Array Dimension Mismatc: Verify that the number of input and output arguments specified in frompyfunc() matches the function’s expectations. Handle dimension mismatches gracefully.
Missing SymPy Installation: Check for the presence of SymPy library before attempting to use it. Provide instructions for users to install SymPy if it is not already installed.
Conclusion
Converting a string into a mathematical function is a common requirement for data scientists when working with mathematical expressions. In this blog post, we explored different approaches to achieve this using Python libraries like NumPy and SciPy.
We started with the eval()
approach, which is simple but has certain security and performance considerations. We then looked at NumPy’s frompyfunc()
function, which allows us to efficiently evaluate functions across arrays of input arguments. Finally, we explored the SymPy library and its sympify()
function, which provides a comprehensive toolkit for symbolic computations.
By leveraging these techniques, data scientists can dynamically convert strings into executable functions, enabling them to solve equations, optimize models, and perform other mathematical tasks with ease.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.