Python Pandas TypeError unsupported operand types for datetimetime and Timedelta
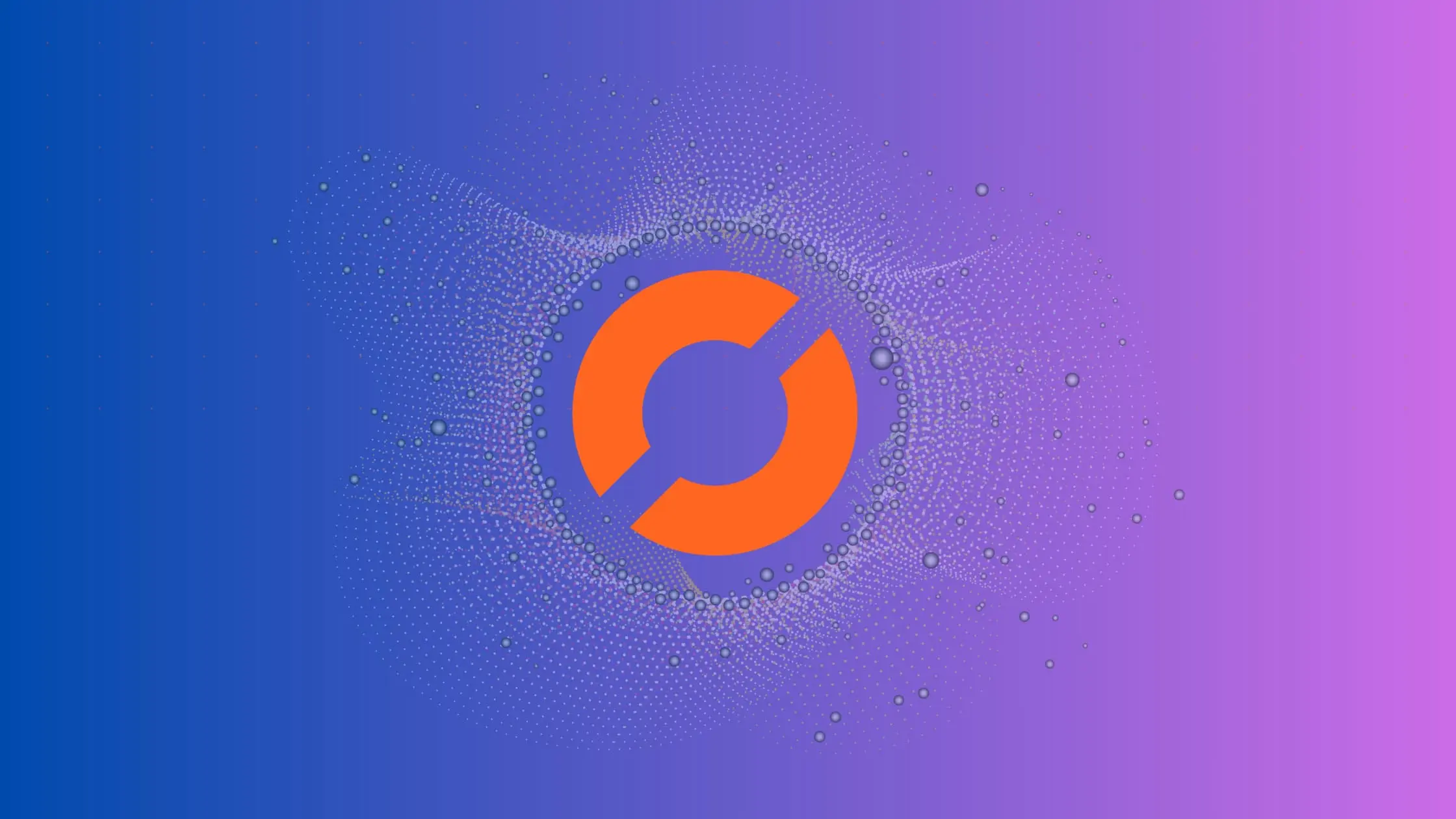
If you are a data scientist or software engineer working with Python, chances are high that you have encountered this error message: “TypeError: unsupported operand type(s) for +: ‘datetime.time’ and ‘Timedelta’”. This error can be frustrating to deal with, especially when you are working with time-series data using the popular Python library, Pandas. In this article, we will examine the causes of this error and various solutions that you can use to fix it.
Table of Contents
Understanding the Error
Before we delve into the possible solutions, let us first understand what this error message means. The error message is telling us that we are trying to add two objects of incompatible types: a datetime.time
object and a Timedelta
object. The datetime.time
object represents a time of day, while the Timedelta
object represents a duration. Attempting to add these two objects does not make sense, and hence, the error message is raised.
Causes of the Error
Now that we understand what the error message means, let us examine some of the possible causes of this error. One common cause of this error is when you are trying to add a datetime.time
object to a Timedelta
object. For instance, consider the following code snippet:
import datetime
import pandas as pd
time_obj = datetime.time(hour=10, minute=30)
delta_obj = pd.Timedelta(minutes=30)
result = time_obj + delta_obj
When you run this code, you will get the following error:
TypeError: unsupported operand type(s) for +: 'datetime.time' and 'Timedelta'
This error occurs because you cannot add a datetime.time
object to a Timedelta
object.
Another common cause of this error is when you are trying to perform arithmetic operations on columns of a Pandas DataFrame that contain datetime.time
objects. For instance, consider the following code snippet:
import datetime
import pandas as pd
df = pd.DataFrame({
'start_time': [datetime.time(hour=10, minute=30), datetime.time(hour=11, minute=30)],
'end_time': [datetime.time(hour=11, minute=30), datetime.time(hour=12, minute=30)]
})
df['duration'] = df['end_time'] - df['start_time']
When you run this code, you will get the following error:
TypeError: unsupported operand type(s) for -: 'datetime.time' and 'datetime.time'
This error occurs because you cannot subtract two datetime.time
objects. Instead, you should convert the datetime.time
objects to datetime.datetime
objects before performing the arithmetic operations.
Solutions to the Error
Now that we have identified some of the common causes of this error, let us examine some of the possible solutions to fix it.
Solution 1: Convert datetime.time Objects to datetime.datetime Objects
If you are trying to perform arithmetic operations on columns of a Pandas DataFrame that contain datetime.time
objects, you should first convert these objects to datetime.datetime
objects. You can do this by combining the datetime.date
object with the datetime.time
object to create a datetime.datetime
object. For instance, consider the following code snippet:
import datetime
import pandas as pd
df = pd.DataFrame({
'start_time': [datetime.time(hour=10, minute=30), datetime.time(hour=11, minute=30)],
'end_time': [datetime.time(hour=11, minute=30), datetime.time(hour=12, minute=30)]
})
df['start_datetime'] = pd.to_datetime('2023-06-18') + pd.to_timedelta(df['start_time'].astype(str))
df['end_datetime'] = pd.to_datetime('2023-06-18') + pd.to_timedelta(df['end_time'].astype(str))
df['duration'] = df['end_datetime'] - df['start_datetime']
In this code, we first create two new columns in the DataFrame, start_datetime
and end_datetime
, by combining the datetime.date
object with the datetime.time
object using the pd.to_timedelta()
function. We then perform the arithmetic operation on these columns to compute the duration.
Pros
Accuracy: This method ensures accurate arithmetic operations since
datetime.datetime
objects can handle both date and time, providing more detailed time information.Flexibility: By converting to
datetime.datetime
, you can perform a wider range of operations, like adding days or adjusting for time zones.
Cons
Complexity: It adds an extra step of conversion which might complicate the code, especially for simple time manipulations.
Potential Overhead: Converting large datasets from
datetime.time
todatetime.datetime
can increase memory usage and processing time.
Solution 2: Use datetime.datetime Objects Instead of datetime.time Objects
If you are working with time-series data and need to perform arithmetic operations, it might be better to store the time information as datetime.datetime
objects instead of datetime.time
objects. This will allow you to perform arithmetic operations without encountering the “unsupported operand type(s) for +: ‘datetime.time’ and ‘Timedelta’” error. For instance, consider the following code snippet:
import datetime
import pandas as pd
df = pd.DataFrame({
'datetime': [datetime.datetime(2023, 6, 18, 10, 30), datetime.datetime(2023, 6, 18, 11, 30)]
})
df['datetime'] = df['datetime'] + pd.to_timedelta('30 minutes')
In this code, we create a DataFrame with a column datetime
containing datetime.datetime
objects. We then add a Timedelta
object to this column to shift the time by 30 minutes.
Pros
Simplicity: Directly using
datetime.datetime
objects simplifies operations as it avoids the need for conversions.Versatility: It allows for straightforward manipulation of both dates and times, which is often required in time-series data analysis.
Cons
Redundancy: If the date part is not relevant, using
datetime.datetime
might store redundant information.Less Intuitive: For scenarios strictly needing time-of-day information, using
datetime.datetime
may be less intuitive and clear thandatetime.time
.
Error Handling
Validate Data Types: Before performing operations, check the data types of your objects. This can prevent unexpected errors and make debugging easier.
Use Exception Handling: Implement
try-except
blocks to gracefully handle errors that might occur during datetime operations. This is especially useful when dealing with data from external sources where the format might vary.Logging: Maintain logs of operations, especially in a production environment. If an error like “TypeError: unsupported operand type(s)” occurs, logs can help trace back to the problematic operation.
Unit Testing: Write unit tests for your time manipulation functions. This ensures that your code behaves as expected and can handle various edge cases.
Time Zone Awareness: Be cautious about time zones when converting between
datetime.time
anddatetime.datetime
. Always specify time zones to avoid errors related to time zone differences.Handle Null Values: Ensure that your code can handle
None
orNaT
(Not a Time) values gracefully, especially when working with real-world datasets that might have missing values.Performance Considerations: For large datasets, benchmark the performance of your datetime operations. Optimize the code if necessary to handle large volumes of data efficiently.
Conclusion
In this article, we examined the “TypeError: unsupported operand type(s) for +: ‘datetime.time’ and ‘Timedelta’” error that you might encounter when working with Pandas and time-series data. We identified some of the common causes of this error and provided two possible solutions to fix it. By following these solutions, you can avoid this error and work with time-series data more efficiently in Python.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.