Python Pandas: Converting Object to String Type in DataFrames
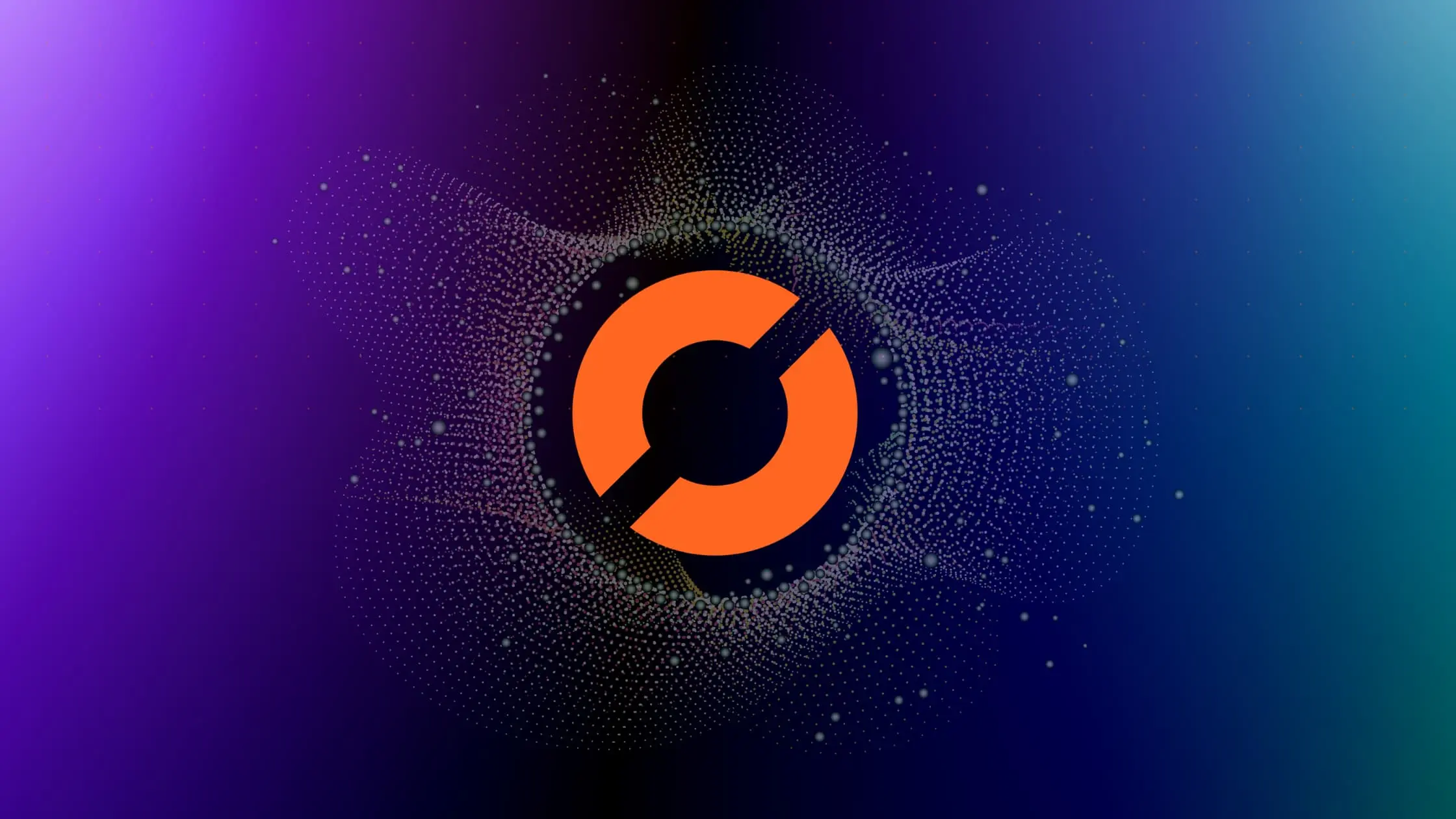
Python Pandas: Converting Object to String Type in DataFrames
In the world of data science, Python’s Pandas library is a powerful tool for data manipulation and analysis. One common task that data scientists often encounter is the need to convert data types within a DataFrame. This blog post will focus on converting object data types to string data types in Pandas DataFrames.
Introduction
Pandas is a software library for Python that provides flexible data structures designed to make working with structured data fast, easy, and expressive. It is a fundamental high-level building block for doing practical, real-world data analysis in Python.
One of the most common data structures in Pandas is the DataFrame, a two-dimensional labeled data structure with columns of potentially different types. DataFrames are similar to SQL tables or Excel sheets, and they are very flexible and powerful.
However, when working with DataFrames, you may encounter situations where you need to convert data from one type to another. This is especially true when dealing with object data types, which are typically used for storing text or mixed numeric and non-numeric values.
In this post, we will walk you through the process of converting object data types to string data types in Pandas DataFrames.
Why Convert Object to String?
Before we dive into the how, let’s discuss the why. Why would you want to convert an object data type to a string data type?
There are several reasons:
Data Consistency: Ensuring that all data in a specific column is of the same type is crucial for data consistency. This is especially important when performing operations on the data, as inconsistent data types can lead to unexpected results or errors.
Data Analysis: Certain types of data analysis require specific data types. For example, if you want to perform text analysis on a column of data, you need to ensure that the data is in string format.
Data Storage and Export: When storing or exporting your data, you may need to convert it to a specific type to meet the requirements of the storage or export format.
How to Convert Object to String in Pandas
Now, let’s dive into the process of converting object data types to string data types in Pandas. Here’s a step-by-step guide:
# Import Pandas
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': ['a', 'b', 'c']})
# Type of column B before converting
print("Type of column B before converting: ", df['B'].dtype)
# Convert column 'B' from object to string
df['B'] = df['B'].astype("string")
# Type of column B after converting
print("Type of column B after converting: ", df['B'].dtype)
Type of column B before converting: object
Type of column B after converting: string
In the code above, we first import the Pandas library. We then create a DataFrame with two columns: A
and B
. Column A
contains integers, and column B
contains objects.
To convert column B
from object to string, we use the astype()
function, which is a function that converts the data type of a Pandas Series. We pass the string string
to the astype()
function to specify that we want to convert the data to string type.
Conclusion
Converting object data types to string data types in Pandas is a common task in data science. It’s crucial for data consistency, data analysis, and data storage and export. With the astype()
function, you can easily perform this conversion and ensure that your data is in the format you need for your analysis.
Data type conversion is just one of the many powerful features of the Pandas library. By mastering these features, you can make your data analysis process more efficient and effective. Stay tuned for more posts on how to leverage the power of Python and Pandas in your data science projects!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.