PySpark DataFrame: Filtering Columns with Multiple Values
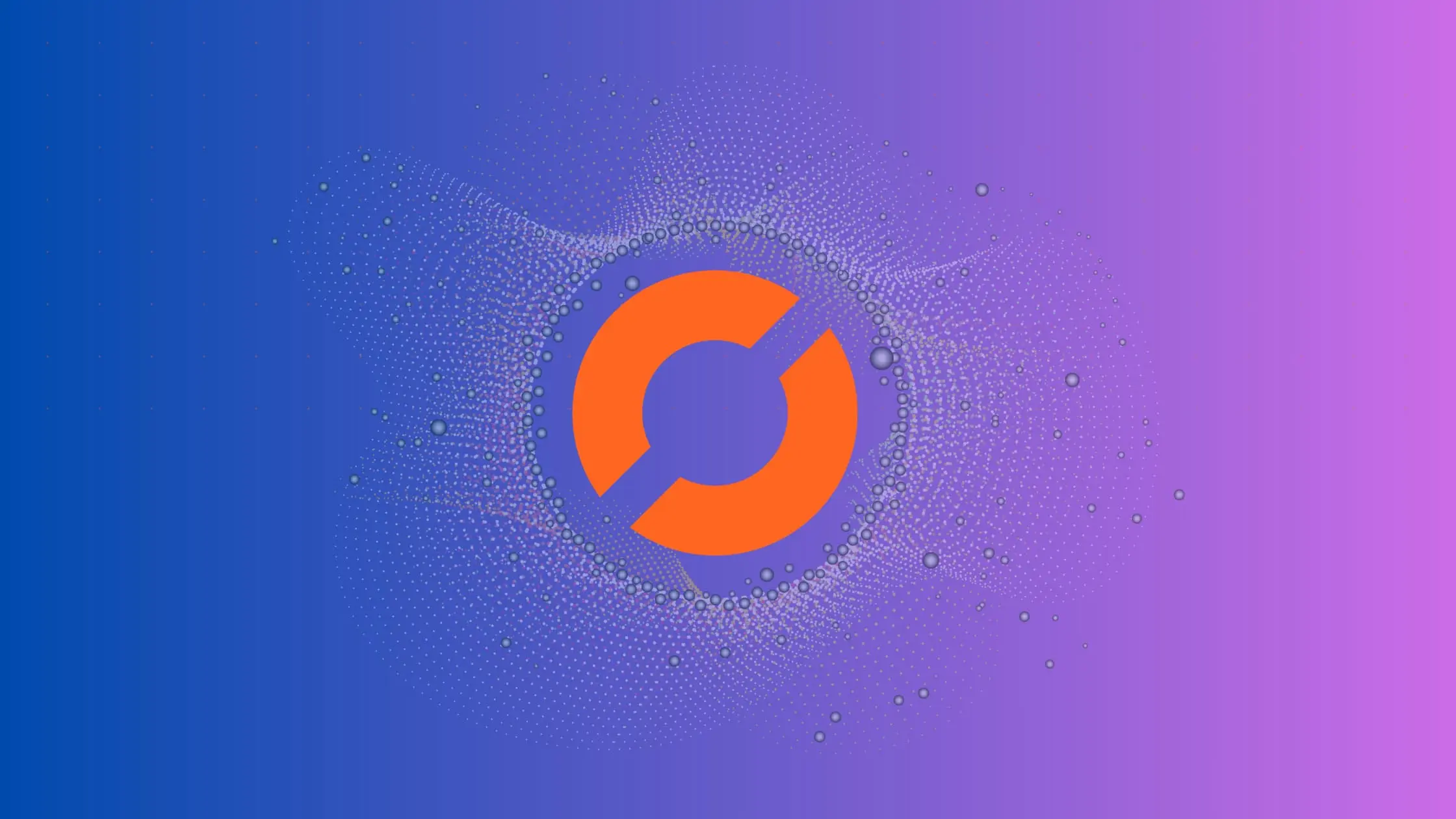
In the realm of big data processing, PySpark has emerged as a powerful tool for data scientists. It allows for distributed data processing, which is essential when dealing with large datasets. One common operation in data processing is filtering data based on certain conditions. In this blog post, we’ll explore how to filter a DataFrame column that contains multiple values in PySpark.
Table of Contents
- Introduction to PySpark DataFrame
- Filtering Columns with PySpark DataFrame
- Best Practices
- Pros and Cons Comparison
- Common Errors and How to Handle Them
- Conclusion
Introduction to PySpark DataFrame
PySpark DataFrame is a distributed collection of data organized into named columns. It is conceptually equivalent to a table in a relational database or a data frame in Python, but with optimizations for speed and functionality under the hood. PySpark DataFrames are designed for processing large amounts of structured or semi-structured data.
Filtering Columns with PySpark DataFrame
Using filter
Transformation
The filter
transformation in PySpark allows you to specify conditions to filter rows based on column values. It is a straightforward and commonly used method.
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("example").getOrCreate()
# Assuming df is your DataFrame
filtered_df = df.filter((df['column'] == 'value1') | (df['column'] == 'value2'))
Utilizing SQL Expressions
PySpark also supports SQL-like expressions for DataFrame operations. This can be especially useful for users familiar with SQL.
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("example").getOrCreate()
# Assuming df is your DataFrame
filtered_df = df.filter("column = 'value1' OR column = 'value2'")
Leveraging when
and otherwise
Functions
The when
and otherwise
functions allow you to apply conditional logic to DataFrames. This method is beneficial for complex filtering scenarios.
from pyspark.sql import SparkSession
from pyspark.sql.functions import when
spark = SparkSession.builder.appName("example").getOrCreate()
# Assuming df is your DataFrame
filtered_df = df.withColumn("column", when(df['column'].isin('value1', 'value2'), 'filtered_value').otherwise(df['column']))
Best Practices
Optimal Code Performance
- Predicate Pushdown: Leverage predicate pushdown whenever possible to minimize data movement and improve performance.
# Use predicate pushdown for optimal performance
filtered_df = df.filter((df['column'] == 'value1') & (df['other_column'] > 100))
Code Readability and Maintainability
- Use Descriptive Column Names: Choose meaningful names for columns to enhance code readability and maintainability.
# Use descriptive column names
filtered_df = df.filter((df['category'] == 'electronics') & (df['price'] > 100))
Optimizing Filtering for Large Datasets
When dealing with large datasets, it’s important to optimize your operations for speed. Here are a few tips for optimizing your filtering operations:
Use broadcast variables: If your list of values is small, you can use a broadcast variable to speed up the operation. A broadcast variable is a read-only variable that is cached on each worker node, rather than being sent over the network with each task.
from pyspark.sql.functions import broadcast colors = ['Red', 'Blue'] df_filtered = df.filter(broadcast(df['Color']).isin(colors))
Filter early: Apply your filters as early as possible in your data processing pipeline. This reduces the amount of data that needs to be processed in subsequent steps.
Use column pruning: Only select the columns you need for your analysis. This reduces the amount of data that needs to be processed and sent over the network.
Pros and Cons Comparison
Method | Pros | Cons |
---|---|---|
filter Transformation | Simple and easy to understand | May become verbose for complex conditions |
SQL Expressions | Familiar syntax for users with SQL background | Limited support for complex conditions |
when and otherwise | Powerful for complex transformations | May be overkill for simple filtering |
Common Errors and How to Handle Them
Incorrect Column Names
Error: pyspark.sql.utils.AnalysisException: "cannot resolve 'column' given input columns"
Solution: Ensure that the column name is correct and matches the DataFrame’s schema.
Unsupported Data Types
Error: TypeError: condition should be string or Column
Solution: Verify that the condition is a valid string or Column object and check for unsupported data types.
Ambiguous Column References
Error: org.apache.spark.sql.AnalysisException: Reference 'column' is ambiguous
Solution: Specify the DataFrame alias for ambiguous column references.
Conclusion
Filtering columns with multiple values is a common operation in data processing. PySpark provides powerful tools for this task, allowing us to easily filter a DataFrame based on a list of values. By using broadcast variables and applying filters early, we can optimize our operations for speed when dealing with large datasets.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.