PandasPython Fill empty cells with previous row value
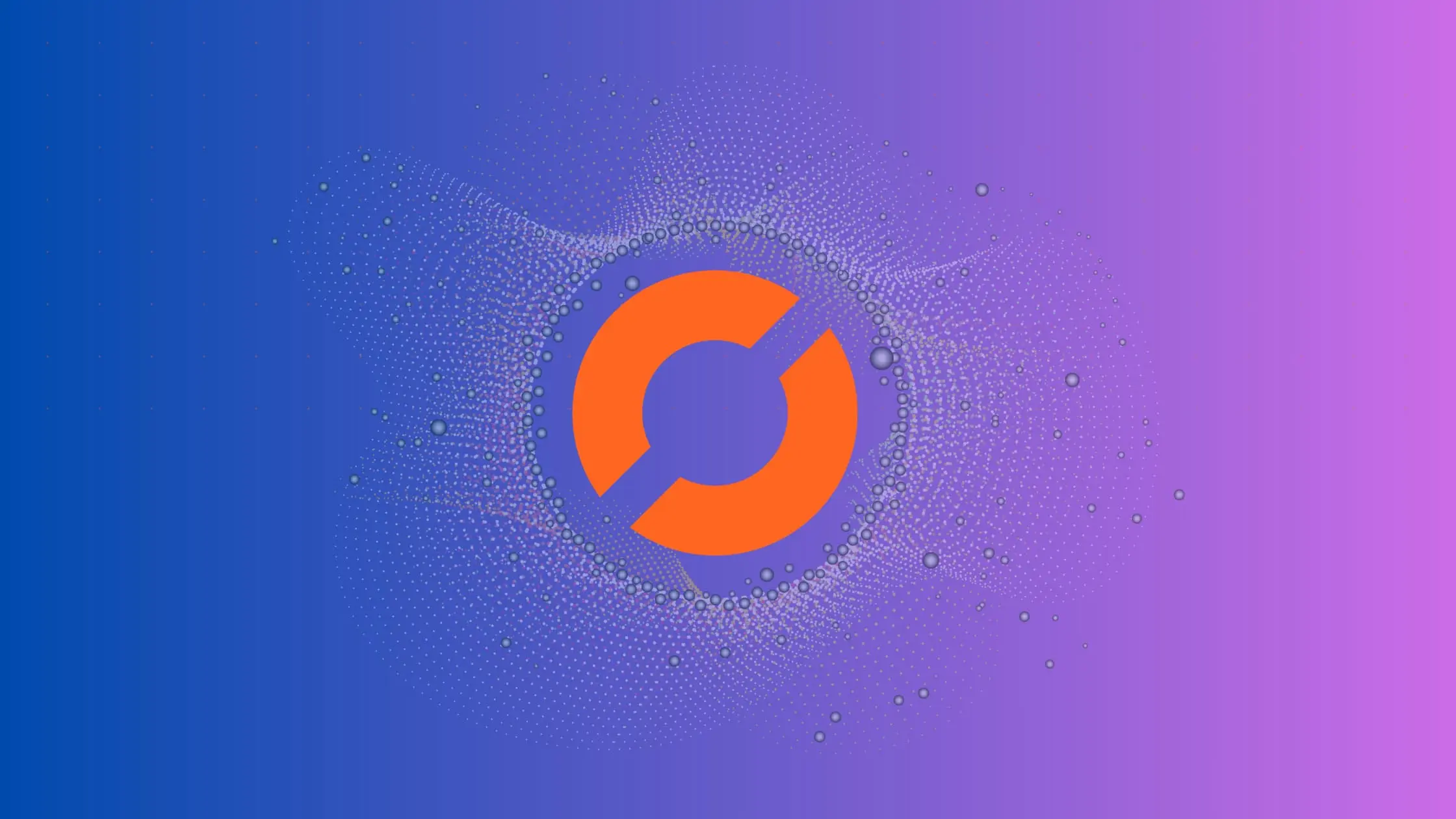
As a data scientist or software engineer, you might encounter datasets that have missing values. These missing values can be problematic when it comes to data analysis or machine learning. One common solution to this problem is to fill the missing values with the previous row value. In this blog post, we will explore how to fill empty cells with the previous row value using the Pandas library in Python.
Table of Contents
- What is Pandas?
- Why Fill Empty Cells with the Previous Row Value?
- How to Fill Empty Cells with the Previous Row Value in Pandas
- Pros and Cons of Using
ffill
- Common Errors and Solutions
- Conclusion
What is Pandas?
Pandas is a popular data manipulation library in Python. It provides data structures and tools for working with structured data seamlessly. Pandas is built on top of NumPy and is great for working with tabular data. It is widely used in data science, finance, and other fields that deal with data analysis and manipulation.
Why Fill Empty Cells with the Previous Row Value?
When working with time-series data, it is common for some values to be missing. Filling these missing values with the previous row value is a common technique for handling missing data. This is because time-series data often exhibits a strong correlation between adjacent values. Filling the missing values with the previous row value can help to maintain the integrity of the data and ensure that the time-series data is well-structured.
How to Fill Empty Cells with the Previous Row Value in Pandas
Pandas provides a simple and intuitive way to fill empty cells with the previous row value using the fillna()
method. The fillna()
method can be used to fill missing values in a DataFrame or Series object.
Let’s discover how to fill empty cells with the previous row value in a DataFrame:
Method 1: fillna with method=ffill
import pandas as pd
# Create a sample DataFrame
data = {'A': [1, 2, None, 4, 5],
'B': [10, None, 30, None, 50]}
df = pd.DataFrame(data)
# Fill NaN values with the previous row values
df.fillna(method='ffill', inplace=True)
print(df)
Method 2: fillna with method=pad
import pandas as pd
# Create a sample DataFrame
data = {'A': [1, 2, None, 4, 5],
'B': [10, None, 30, None, 50]}
df = pd.DataFrame(data)
# Fill NaN values with the previous row values
df.fillna(method='pad', inplace=True)
print(df)
Output:
A B
0 1.0 10.0
1 2.0 10.0
2 2.0 30.0
3 4.0 30.0
4 5.0 50.0
In the above examples, we create a sample DataFrame with some missing values. We then use the fillna()
method to fill the missing values with the previous row value. The method='ffill'
and pad
argument tells Pandas to fill the missing values with the previous row value. The inplace=True
argument tells Pandas to modify the DataFrame in place.
Pros and Cons of Using ffill
Pros
- Simplicity: These methods are easy to implement, making them suitable for quick data preprocessing.
- Preservation of Temporal Patterns: Useful for time-series data where the previous values are relevant.
Cons
- Assumption of Temporal Order: Methods assume a meaningful temporal order, which may not always be accurate.
- Propagation of Errors: If the first few rows contain NaN values, the errors may propagate through the dataset.
Common Errors and Solutions
Error 1: “ValueError: cannot convert float NaN to integer”
Solution: Ensure the DataFrame has a consistent data type or convert columns appropriately. If your DataFrame contains mixed data types, especially a mix of integers and NaN values, attempting to fill NaN in an integer column may result in a type conflict. Consider converting the column to a floating-point type before filling NaN values.
import pandas as pd # Assuming 'Column_Name' is the column with mixed types df['Column_Name'] = df['Column_Name'].astype(float) df.fillna(method='ffill', inplace=True)
Error 2: “AttributeError: ‘NoneType’ object has no attribute ‘fillna’"
Solution: This error may occur if you attempt to fill NaN values on a non-existent DataFrame. Double-check that your DataFrame is properly loaded and not
None
. If you are creating a DataFrame from external data, ensure that the data is correctly read and assigned to the DataFrame variable.import pandas as pd # Incorrect: df is None # Correct: Check the data loading process and assign it properly df = pd.read_csv('your_data.csv') df.fillna(method='ffill', inplace=True)
Error 3: “ValueError: forward and backward filling are not supported on DataFrame with a MultiIndex”
Solution: If your DataFrame has a MultiIndex, consider resetting it before filling NaN values and then set it back afterward:
df.reset_index(inplace=True) df.fillna(method='ffill', inplace=True) df.set_index(['index', 'other_index'], inplace=True) # Adjust column names accordingly
Error 4: “ValueError: Index contains duplicate entries, cannot reshape”
Solution: Check for and handle duplicate index entries. You may need to reset the index, handle duplicates, and set the index back:
df.reset_index(inplace=True) df.drop_duplicates(inplace=True) # Handle duplicates as needed df.set_index('index', inplace=True) # Adjust column name accordingly df.fillna(method='ffill', inplace=True)
Conclusion
Filling empty cells with the previous row value is a common technique for handling missing data in time-series data. In this blog post, we have explored how to fill empty cells with the previous row value using the Pandas library in Python. Pandas provides a simple and intuitive way to fill missing values in a DataFrame or Series object. By using the fillna()
method with the method='ffill'
argument, we can fill the missing values with the previous row value. This technique can help to maintain the integrity of the data and ensure that the time-series data is well-structured.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.