Pandas warning when using map A value is trying to be set on a copy of a slice from a DataFrame
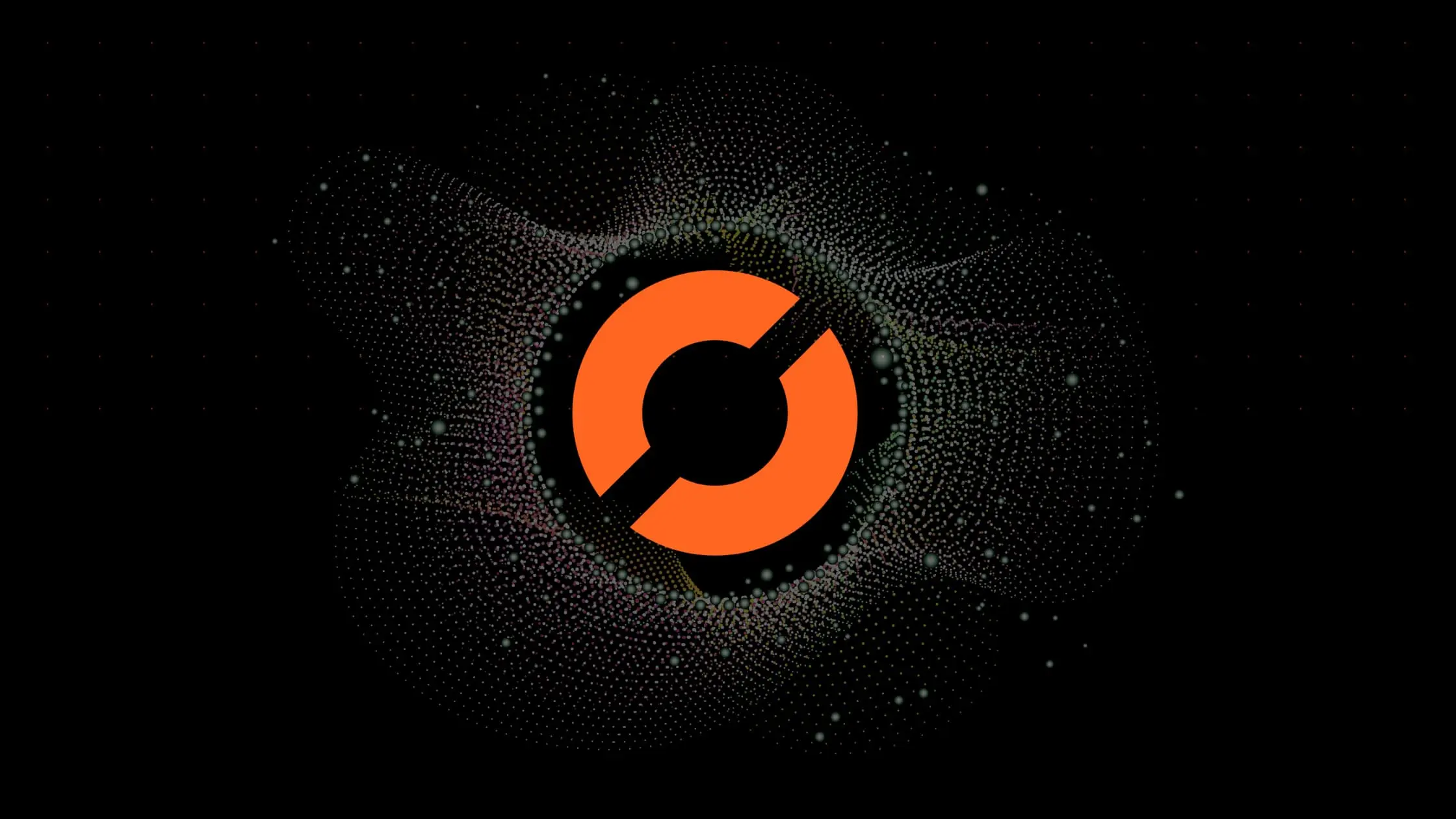
Pandas warning when using map A value is trying to be set on a copy of a slice from a DataFrame
As a data scientist or software engineer, you are likely familiar with the Python library Pandas. Pandas is a powerful and widely used library for data manipulation and analysis. However, when using the map
function in Pandas, you may encounter a warning message that reads:
SettingWithCopyWarning: A value is trying to be set on a copy of a slice from a DataFrame.
This warning can be confusing and frustrating, especially if you’re not sure what it means or how to fix it. In this article, we’ll explain what this warning message means and provide some tips on how to avoid it in your code.
What is the SettingWithCopyWarning?
When you use the map
function in Pandas, you are essentially applying a function to each element in a specified column of a DataFrame. This can be a useful way to transform data and create new columns based on existing ones.
However, when you use map
in certain ways, Pandas may create a copy of the original DataFrame instead of modifying it in place. This can lead to unexpected behavior and errors, particularly if you try to modify the copy in some way.
The SettingWithCopyWarning
is a warning message that Pandas produces when you try to modify a copy of a DataFrame that was created by a previous operation, such as map
. This warning is meant to alert you to the fact that you may be inadvertently modifying a copy of your data instead of the original DataFrame.
Why does this warning occur?
The SettingWithCopyWarning
occurs when Pandas creates a copy of a DataFrame instead of modifying it in place. This can happen in a few different situations, but it often occurs when you chain multiple operations together.
For example, consider the following code:
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = df[df['A'] > 1]
df2['B'] = 0
This code creates a DataFrame df
with two columns, A
and B
, and then creates a new DataFrame df2
that contains only the rows where A
is greater than 1. Finally, it sets the value of the B
column in df2
to 0.
However, when you run this code, you will receive the following warning message:
SettingWithCopyWarning: A value is trying to be set on a copy of a slice from a DataFrame.
This warning is being generated because df2
is actually a copy of a slice of df
, and modifying df2
may not have the intended effect on the original DataFrame df
.
How to avoid the SettingWithCopyWarning
To avoid the SettingWithCopyWarning
, you need to ensure that you are modifying the original DataFrame, rather than a copy of it. There are a few different ways to do this:
1. Use .loc to modify the original DataFrame
One way to modify the original DataFrame is to use the .loc
accessor. The .loc
accessor allows you to modify a subset of the DataFrame in place, rather than creating a copy.
For example, consider the following code:
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df.loc[df['A'] > 1, 'B'] = 0
This code modifies the B
column of df
to 0 for all rows where A
is greater than 1. Importantly, this code modifies the original DataFrame df
in place, rather than creating a copy.
2. Use .copy() to explicitly create a copy of the DataFrame
Another way to avoid the SettingWithCopyWarning
is to explicitly create a copy of the DataFrame using the .copy()
method. This ensures that any modifications you make to the DataFrame will not affect the original DataFrame.
For example, consider the following code:
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = df[df['A'] > 1].copy()
df2['B'] = 0
This code creates a copy of the slice of df
where A
is greater than 1 and assigns it to df2
. Then, it sets the value of the B
column in df2
to 0. Importantly, this code modifies the copy df2
, rather than modifying the original DataFrame df
.
3. Use .apply() instead of .map()
Finally, you can avoid the SettingWithCopyWarning
by using the .apply()
method instead of the .map()
method. The .apply()
method applies a function to each row or column of a DataFrame, rather than each element in a specified column.
For example, consider the following code:
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df['C'] = df['A'].apply(lambda x: x * 2)
This code creates a new column C
in df
by applying a lambda function to each element in the A
column. Because we’re using .apply()
instead of .map()
, we avoid the SettingWithCopyWarning
.
Conclusion
The SettingWithCopyWarning
can be a frustrating warning message to encounter when working with Pandas. However, by understanding why this warning occurs and how to avoid it, you can write more robust and error-free code.
In summary, you can avoid the SettingWithCopyWarning
by using .loc
to modify the original DataFrame, using .copy()
to explicitly create a copy of the DataFrame, or using .apply()
instead of .map()
. By following these strategies, you can ensure that your Pandas code is more reliable and easier to maintain.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.