Pandas ValueError cannot convert float NaN to integer
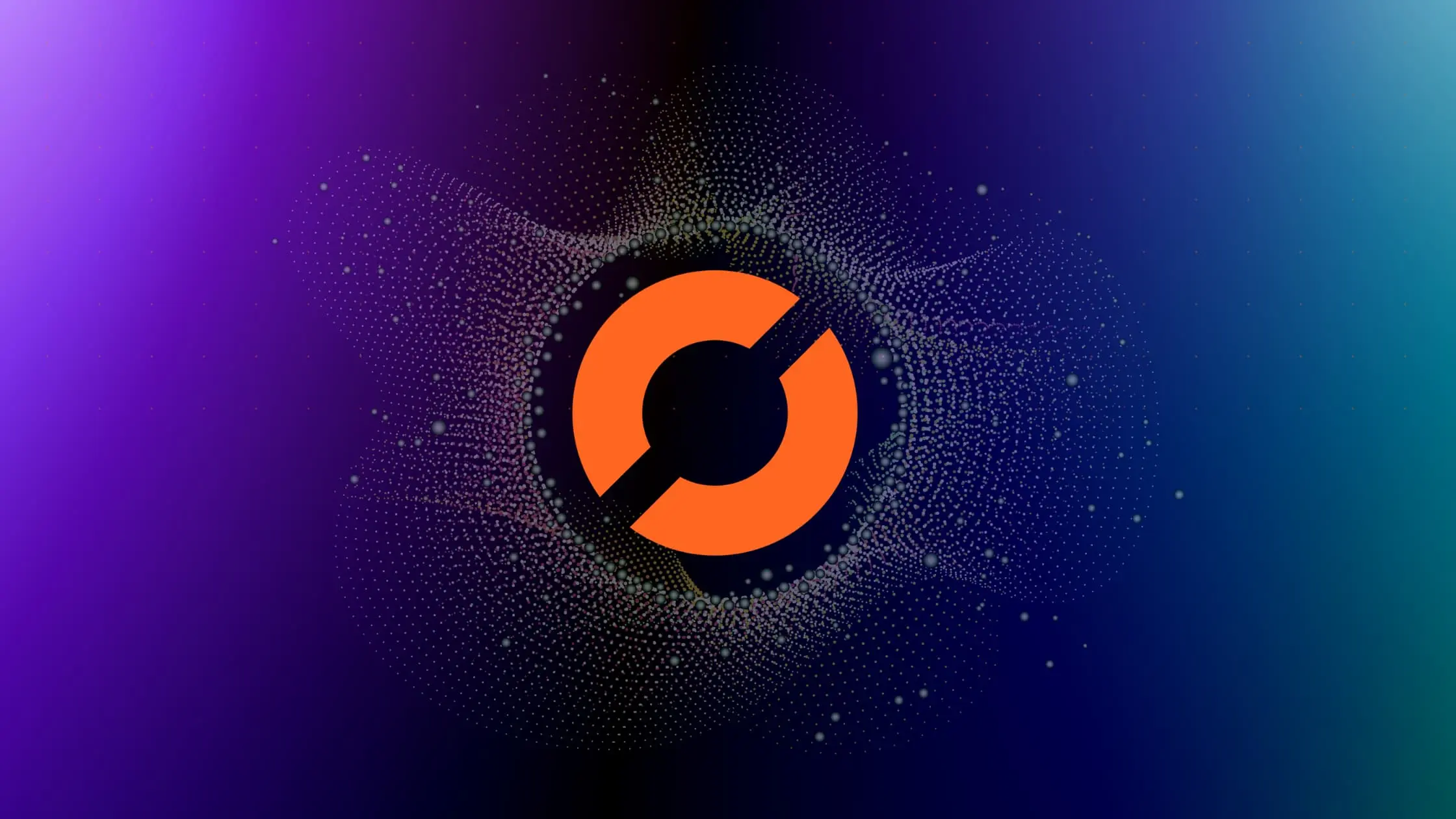
As a data scientist or software engineer, there are few things more frustrating than encountering an error in your code. One such error that you may have come across while working with Pandas is the ValueError: cannot convert float NaN to integer
error. In this article, we will explain what this error means, why it occurs, and how you can fix it.
What is Pandas?
Before diving into the specifics of the error message, it is important to understand what Pandas is and why it is so widely used in data science. Pandas is a Python library that is used for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets, as well as a wide range of functions for working with those datasets.
Pandas is particularly useful for working with tabular data, such as spreadsheets or databases. It allows you to load data from a variety of sources, manipulate it in various ways, and then export it to a new format or database for further analysis.
Understanding the Error Message
Now that we have a basic understanding of what Pandas is, let’s take a closer look at the error message itself. The ValueError: cannot convert float NaN to integer
error typically occurs when you are trying to convert a column of data that contains NaN (Not a Number) values from float to integer.
The reason for this error is that NaN values are not considered valid integers, and therefore cannot be converted to integers. When Pandas encounters a NaN value in a column that is being converted to an integer, it raises the ValueError
exception.
How to Fix the Error
If you encounter the ValueError: cannot convert float NaN to integer
error in your code, there are a few ways to fix it. Here are some possible solutions:
Solution 1: Replace NaN Values
The most straightforward solution to this error is to replace any NaN values in the column with a valid integer value. You can do this using the fillna()
function in Pandas.
For example, let’s say you have a DataFrame df
with a column called my_column
that contains NaN values. You could replace those NaN values with a value of 0 using the following code:
import pandas as pd
import numpy as np
# Create a sample DataFrame with NaN values
data = {'my_column': [1, 2, np.nan, 4, 5, np.nan, 7, 8, 9]}
df = pd.DataFrame(data)
df['my_column'] = df['my_column'].fillna(0).astype(int)
Output:
my_column
0 1
1 2
2 0
3 4
4 5
5 0
6 7
7 8
8 9
This code first fills any NaN values in the my_column
column with a value of 0 using the fillna()
function. It then converts the column to an integer using the astype()
function.
Solution 2: Convert to Float
If you cannot replace the NaN values with a valid integer value, another solution is to convert the column to a float instead of an integer. NaN values are considered valid floats, so you will not encounter the ValueError
exception when converting the column.
For example, you could convert the my_column
column to a float using the following code:
df['my_column'] = df['my_column'].astype(float)
Output:
my_column
0 1.0
1 2.0
2 NaN
3 4.0
4 5.0
5 NaN
6 7.0
7 8.0
8 9.0
Solution 3: Use a Different Data Type
Finally, if neither of the above solutions work for your specific use case, you may need to consider using a different data type altogether. For example, you could use a string data type instead of an integer or float, which would allow you to include NaN values in the column.
Conclusion
The ValueError: cannot convert float NaN to integer
error can be frustrating to encounter, but there are several ways to fix it. By understanding the underlying cause of the error and using the appropriate solution for your specific use case, you can avoid this error and continue working with your data in Pandas.
Remember, Pandas is a powerful tool for data manipulation and analysis, but it requires a solid understanding of its functions and parameters to use effectively. With practice and experience, you will become better equipped to handle errors like this and work more efficiently with your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.