Pandas TypeError not supported between instances of int and str when selecting on date column
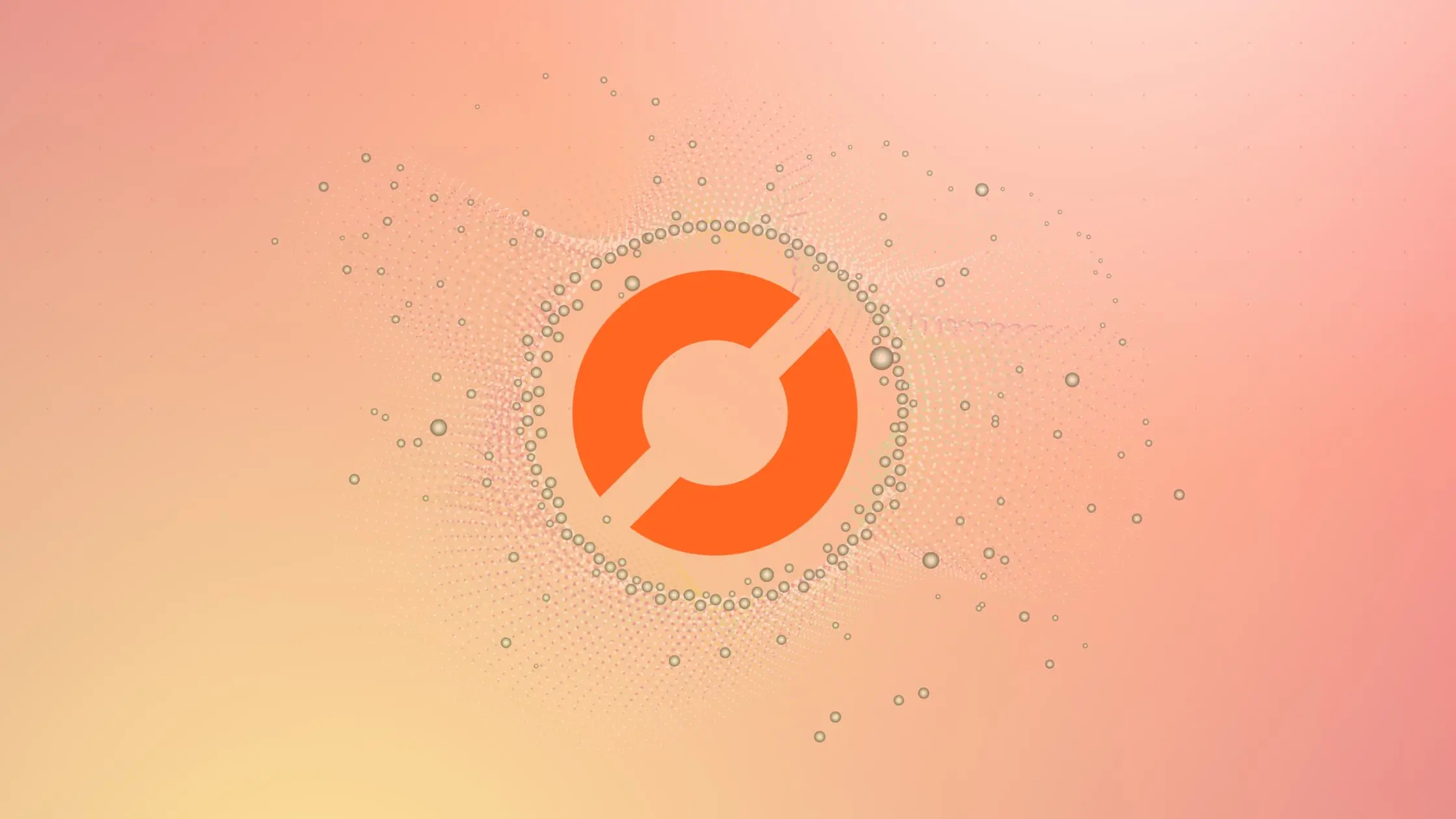
If you’re a data scientist or software engineer who frequently works with data, chances are you’ve used Pandas before. Pandas is a powerful Python library used for data manipulation and analysis. It’s an essential tool for any data professional, but sometimes you can run into errors that can be frustrating to debug. In this article, we’ll discuss a common error you might encounter when selecting data on a date column in Pandas: “TypeError: ‘>’ not supported between instances of ‘int’ and ‘str’”. We’ll explain what this error means and how to fix it.
What is the "TypeError: '>' not supported between instances of 'int' and 'str'"
error?
The "TypeError: '>' not supported between instances of 'int' and 'str'"
error is a common error in Pandas that occurs when you try to compare a date column with a string or integer. This error message is telling you that you’re trying to compare values of different types, which is not allowed. For example, if you have a date column in your DataFrame and you try to select all rows where the date is greater than a certain value, you might encounter this error if the value you’re comparing it to is a string or integer.
How to fix the "TypeError: '>' not supported between instances of 'int' and 'str'"
error?
There are a few ways to fix the "TypeError: '>' not supported between instances of 'int' and 'str'"
error in Pandas. One solution is to convert the date column to the correct data type. By default, Pandas will often read in date columns as strings, so you need to explicitly convert them to datetime objects. You can do this using the pd.to_datetime()
function. Here’s an example:
import pandas as pd
# Creating a DataFrame
data = {'ID': [1, 2, 3, 4],
'Date': ['2022-01-01', '2022-02-01', 20220301, '2022-04-01']}
df = pd.DataFrame(data)
# Attempting to filter data based on the 'Date' column
selected_data = df[df['Date'] > '2022-02-01']
Output:
TypeError: '>' not supported between instances of 'int' and 'str'
In this example, the Date
column contains a mix of string and integer values. When trying to filter the DataFrame based on the condition 'Date' > '2022-02-01'
, the TypeError will be raised.
To resolve this issue, we need to ensure that the data type of the Date
column is consistent. We can achieve this by converting the entire column to a specific data type, such as datetime.
import pandas as pd
# Creating a DataFrame
data = {'ID': [1, 2, 3, 4],
'Date': ['2022-01-01', '2022-02-01', 20220301, '2022-04-01']}
df = pd.DataFrame(data)
df['Date'] = pd.to_datetime(df['Date'])
# Attempting to filter data based on the 'Date' column
selected_data = df[df['Date'] > '2022-02-01']
print(selected_data)
Output:
ID Date
3 4 2022-04-01
In this example, we create a DataFrame with a date column and a value column. We then convert the date column to a datetime object using the pd.to_datetime()
function. Finally, we select all rows where the date is greater than ‘2022-02-01’. This should work without any errors.
Conclusion
The "TypeError: '>' not supported between instances of 'int' and 'str'"
error is a common error you might encounter when selecting data on a date column in Pandas. This error occurs when you try to compare a date column with a string or integer. To fix this error, you can convert the date column to a datetime object using the pd.to_datetime()
function or make sure that the value you’re comparing the date column with is also a datetime object. Hopefully, this article has helped you understand this error and how to fix it.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.