Pandas: Selecting Multiple Columns from One Row
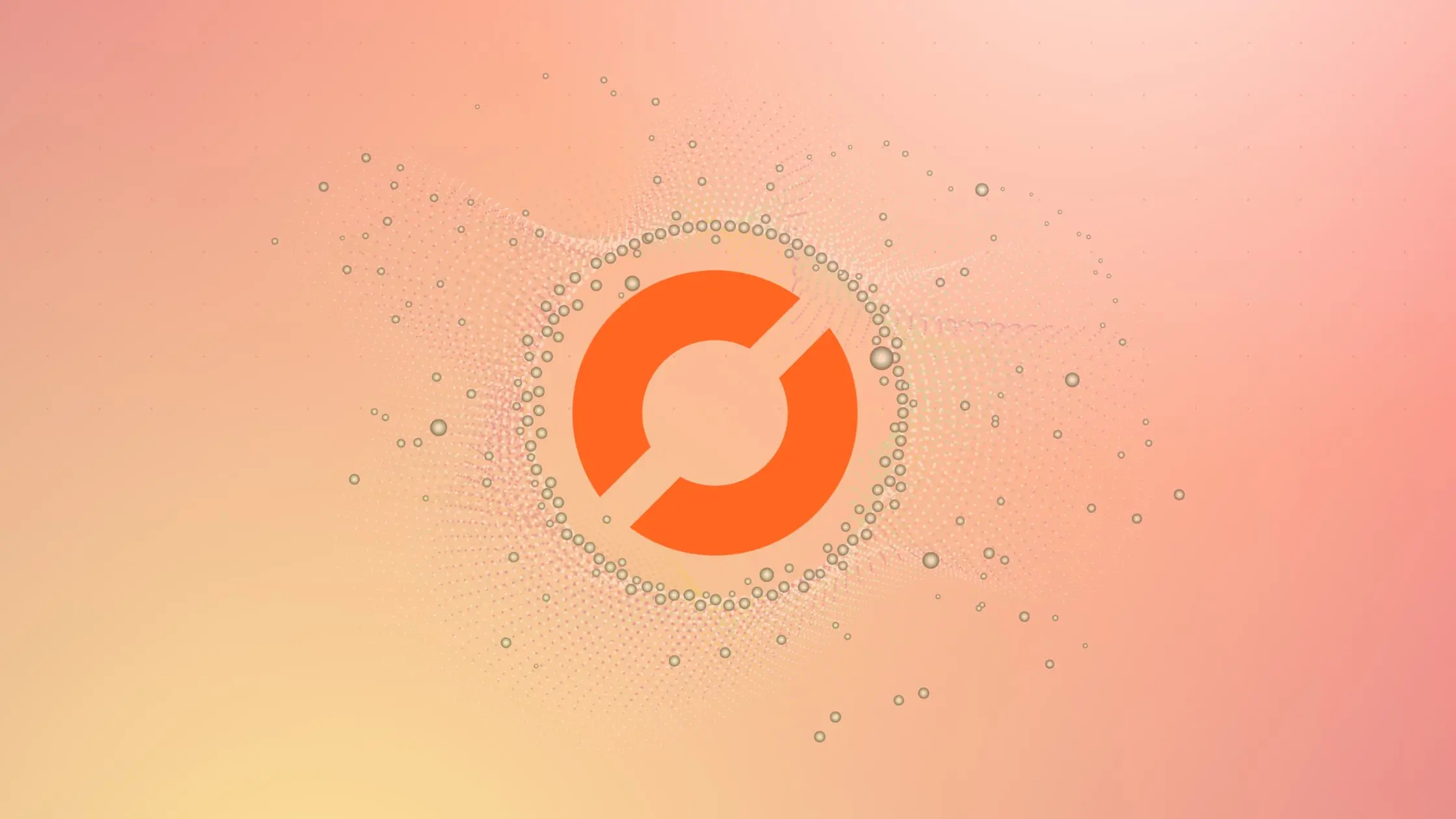
If you are working with large datasets in the field of data science or software engineering, you are likely to come across the need to extract specific information from a given dataset. Pandas is a powerful and widely used Python library that provides a range of data manipulation capabilities. One such capability is the ability to select multiple columns from one row of a pandas dataframe. In this blog post, we will discuss how to do this efficiently.
Table of Contents
What is Pandas?
Pandas is a Python library that is used for data manipulation and analysis. It provides a range of functions and tools for working with structured data, such as spreadsheets or SQL tables. Pandas is built on top of NumPy, another popular Python library that is used for scientific computing.
Pandas dataframes are similar to spreadsheets in that they are two-dimensional tables with labeled columns and rows. Each column can have a different datatype, such as integers, floats, or strings.
How to Select Multiple Columns from One Row
Using loc
method
In Pandas, you can select multiple columns from a dataframe by specifying a list of column names. This is also true when selecting columns from a single row. To select multiple columns from one row, you can use the loc
method.
Consider the following example dataframe:
import pandas as pd
data = {'Name': ['John', 'Jane', 'Alice', 'Bob', 'Chris'],
'Age': [25, 32, 18, 47, 29],
'Country': ['USA', 'Canada', 'Australia', 'USA', 'UK'],
'Salary': [50000, 75000, 40000, 90000, 60000]}
df = pd.DataFrame(data)
This dataframe has four columns: Name
, Age
, Country
, and Salary
. Let’s say we want to select the Age
, Country
, and Salary
columns for the row where Name
is ‘Alice’. We can do this as follows:
row = df.loc[df['Name'] == 'Alice', ['Age', 'Country', 'Salary']]
print(row)
Output:
Age Country Salary
2 18 Australia 40000
Here, we first use the loc
method to select the row where Name
is ‘Alice’. We do this by specifying the condition df['Name'] == 'Alice'
inside the loc
method.
Next, we specify a list of column names that we want to select from this row. We do this by using the []
operator and passing a list of column names: ['Age', 'Country', 'Salary']
.
After executing this code, the row
variable will contain the selected columns for the row where Name
is Alice
.
Using iloc
method
The iloc
method in Pandas allows you to select data by integer location. You can use it to select specific columns from a particular row by specifying the row index and column indices.
# Example using iloc
row_index = df.index[df['Name'] == 'Alice'].tolist()[0] # Get the row index where Name is 'Alice'
columns_to_select = [1, 2, 3] # Indices of columns 'Age', 'Country', 'Salary'
row = df.iloc[row_index, columns_to_select]
print(row)
Output:
Age 18
Country Australia
Salary 40000
Name: 2, dtype: object
Using at
method
The at
method is used for fast label-based scalar access. You can use it to directly access a single value in the dataframe based on row and column labels.
# Example using at
row_index = df.index[df['Name'] == 'Alice'].tolist()[0] # Get the row index where Name is 'Alice'
selected_data_age = df.at[row_index, 'Age']
selected_data_country = df.at[row_index, 'Country']
selected_data_salary = df.at[row_index, 'Salary']
row = pd.Series({'Age': selected_data_age, 'Country': selected_data_country, 'Salary': selected_data_salary})
print(row)
Output:
Age 18
Country Australia
Salary 40000
dtype: object
Common Errors and Solutions
Error 1: KeyError - Column Name Not Found
# Error: 'City' column does not exist
row_error = df.loc[df['Name'] == 'Alice', ['Age', 'City', 'Salary']]
Solution: Ensure that the column names specified in the list are accurate and exist in the dataframe. Double-check for typos or use the columns
attribute to get the list of valid column names.
# Solution: Correcting the column name to 'Country'
row_solution = df.loc[df['Name'] == 'Alice', ['Age', 'Country', 'Salary']]
Error 2: IndexError - Row Not Found
# Error: IndexError as there is no row where Name is 'Eve'
row_error = df.loc[df['Name'] == 'Eve', ['Age', 'Country', 'Salary']]
Solution: Check if the condition for row selection is met. Handle cases where the specified row does not exist to avoid IndexError.
# Solution: Adding a check for the existence of the row
if not df[df['Name'] == 'Eve'].empty:
row_solution = df.loc[df['Name'] == 'Eve', ['Age', 'Country', 'Salary']]
else:
print("Row not found.")
Conclusion
In conclusion, selecting multiple columns from one row in a pandas dataframe is a simple and straightforward process. You can use the loc
method to select a specific row and then specify a list of column names to extract the desired information. This capability is a powerful tool for data manipulation and analysis in the field of data science and software engineering.
By using Pandas, data scientists and software engineers can streamline their data analysis workflows and quickly extract specific information from large datasets. If you are interested in learning more about Pandas or data manipulation in Python, I encourage you to explore the official Pandas documentation and experiment with your own code examples.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.