Pandas Read specific Excel cell value into a variable
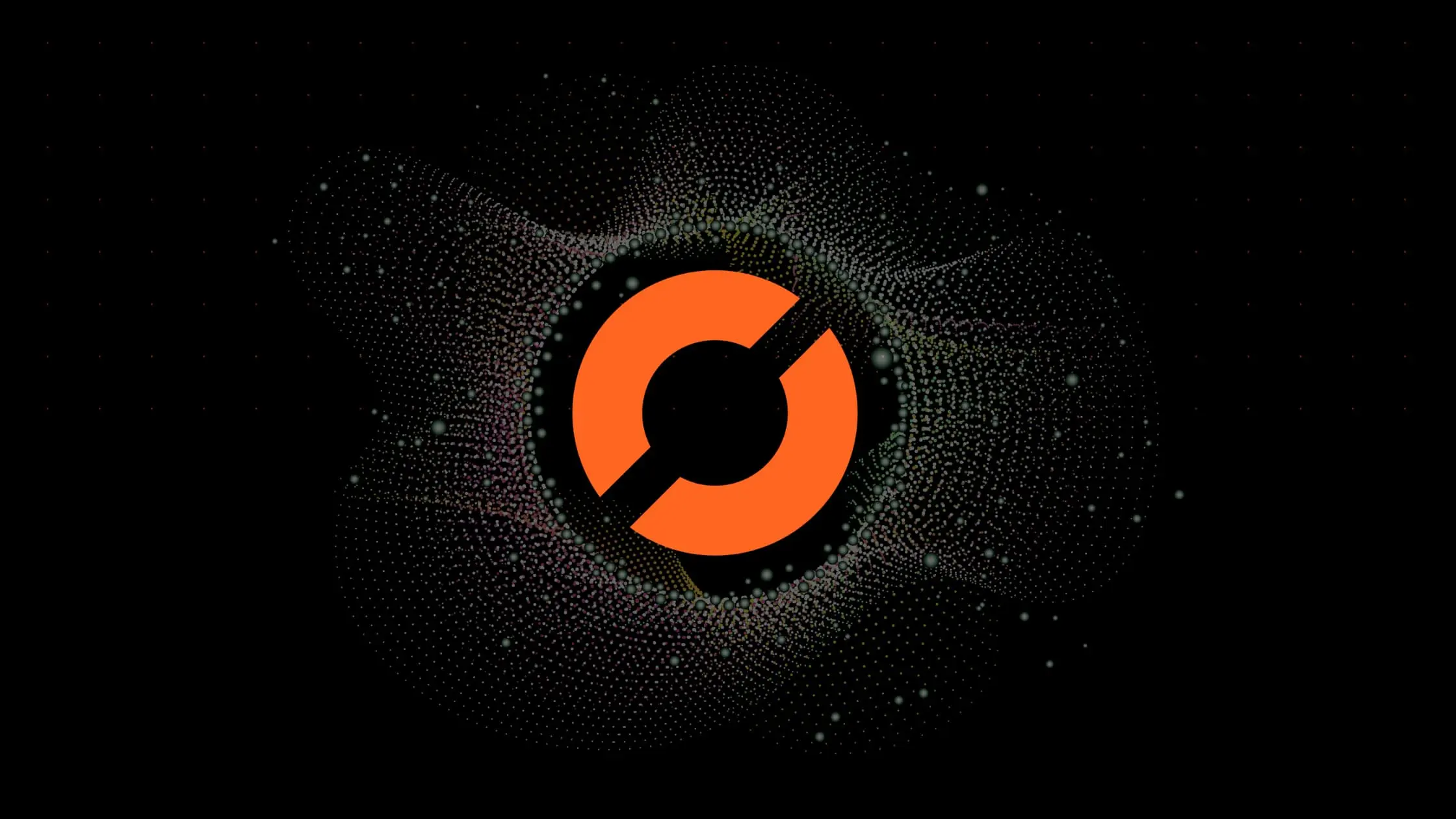
As a data scientist or software engineer, you may have encountered a situation where you need to read a specific Excel cell value into a variable. To do this, you can use the powerful Python library, pandas.
Pandas is a widely used open-source data analysis and manipulation library. It provides fast and efficient data structures for handling large datasets and has functions for reading and writing data in many different file formats, including Excel.
In this blog post, we will walk you through the process of reading a specific Excel cell value into a variable using pandas. We will assume that you have some basic knowledge of Python and pandas.
Table of Contents
Prerequisites
Before we dive into the solution, make sure you have the following prerequisites in place:
- Python 3.x installed on your system
- pandas library installed on your system
- An Excel file with data that you want to read
Step-by-Step Guide
Follow these steps to read a specific Excel cell value into a variable:
- Import the pandas library
First, you need to import the pandas library by using the following code:
import pandas as pd
This will import the pandas library and assign it to the variable pd
.
- Read the Excel file
Next, you need to read the Excel file into a pandas DataFrame. To do this, use the following code:
df = pd.read_excel("path/to/your/excel/file.xlsx")
print(df)
Replace path/to/your/excel/file.xlsx
with the path to your Excel file. Let’s assumme that we have the following data:
Name Age City
0 John 25 New York
1 Alice 30 San Francisco
2 Bob 22 Los Angeles
- Access the cell value
- Method 1: Using
iloc
To access the value of a specific cell in the Excel file, you need to specify the row and column indices of the cell. In pandas, you can do this by using the.iloc
method.
For example, if you want to read the value of cell A1, you can use the following code:
cell_value = df.iloc[0, 0]
This will read the value of the first row and first column of the DataFrame and assign it to the variable cell_value
.
If you want to read the value of a cell in a different row or column, simply replace the row and column indices in the .iloc
method.
- Method 1: Using
loc
andat
# Example: Reading cell at row 0, column 'Name'
cell_value = df.loc[0, 'Name']
- Print the cell value
Finally, you can print the value of the cell by using the following code:
print(cell_value)
This will print the value of the cell to the console.
Output:
Jone
Best Practices
Tip 1: Specify Sheet Name
Always specify the sheet name when reading an Excel file. This helps avoid confusion if the file contains multiple sheets.
Tip 2: Handling Header Rows
Consider the presence of header rows when reading specific cell values. Adjust the header
parameter accordingly.
Tip 3: Data Type Considerations
Be mindful of the data types in your Excel file. Pandas may infer data types, but specifying them explicitly can prevent issues.
Common Errors and How to Handle Them
Error 1: Incorrect Sheet Name
If encountering an “invalid sheet name” error, double-check and ensure the sheet name is correct.
Error 2: Missing Header Information
For missing header information, adjust the header
parameter accordingly.
Error 3: Data Type Mismatch
Handle data type mismatches by explicitly specifying data types or using conversion methods.
Conclusion
In this blog post, we have shown you how to read a specific Excel cell value into a variable using pandas. By following the steps outlined above, you should now be able to read any cell value from an Excel file and use it in your Python code.
With pandas, you can easily manipulate and analyze large datasets in Python, making it a valuable tool for data scientists and software engineers alike. We hope that this blog post has been helpful in demonstrating one of the many useful functions of this powerful library.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.