Pandas Looking up the list of sheets in an excel file
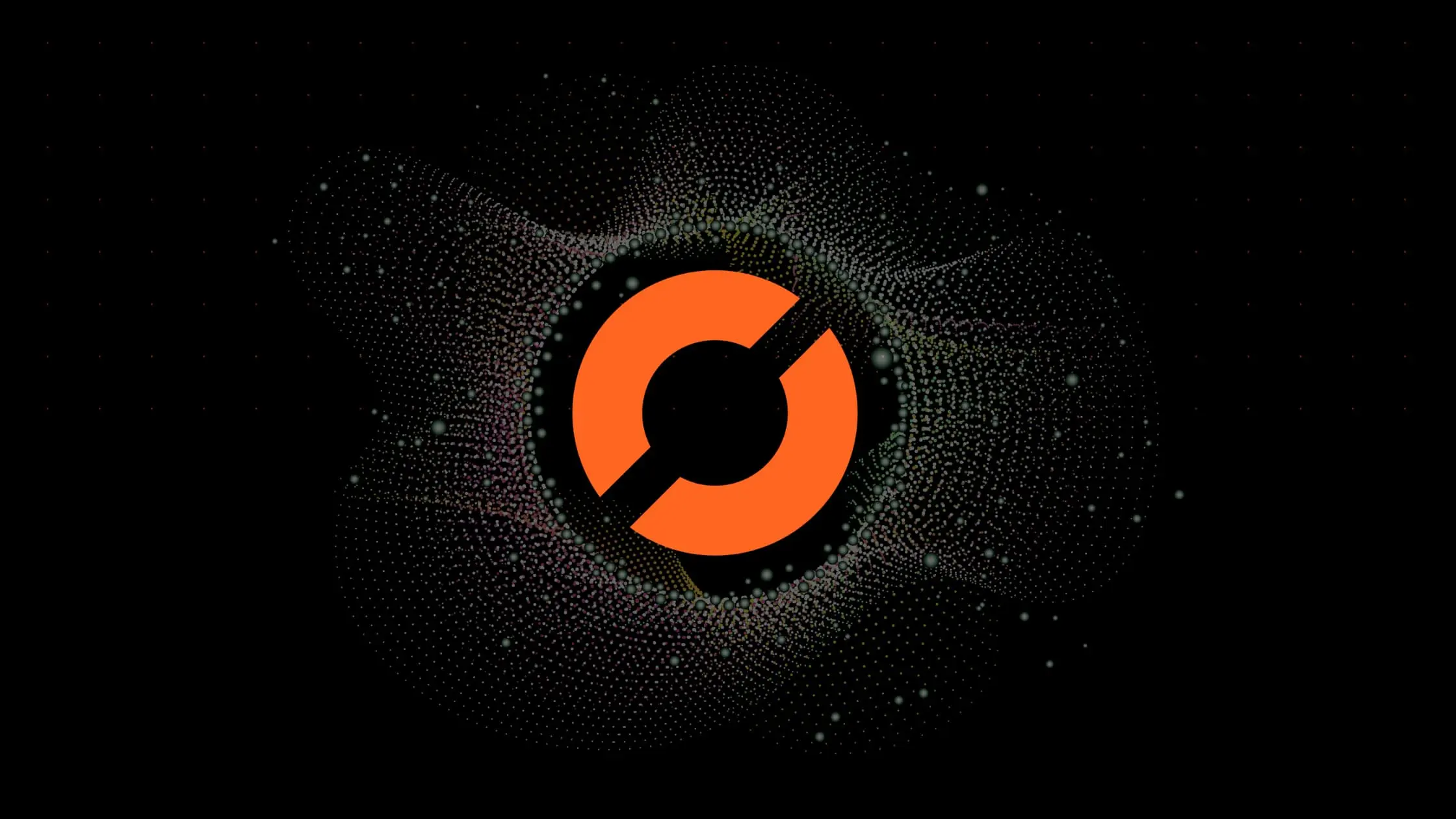
As a data scientist or software engineer, you may frequently encounter situations where you need to work with Excel files. Pandas is a powerful Python library that makes working with Excel files a breeze. One common task when working with Excel files is to look up the list of sheets within the file. In this blog post, we will explore how to do just that using Pandas.
Table of Contents
- What is Pandas?
- How to Look up the List of Sheets in an Excel File using Pandas
- Pros and Cons of Using Pandas to List Sheets in an Excel File
- Error Handling
- Conclusion
What is Pandas?
Pandas is an open-source data manipulation library for Python. It is built on top of NumPy, which is another Python library that provides support for large, multi-dimensional arrays and matrices. Pandas is specifically designed to work with structured data, such as spreadsheets, SQL tables, and databases. It provides data structures for efficiently storing and manipulating data, as well as tools for data cleaning, transformation, and analysis.
How to Look up the List of Sheets in an Excel File using Pandas
Pandas provides a simple way to read an Excel file into a Pandas DataFrame. To do so, we can use the ExcelFile()
class. Tin pandas is used to efficiently parse and analyze Excel files. It is part of the pandas library and provides an interface for working with Excel files without repeatedly opening and closing the file for each operation:
import pandas as pd
# Create an ExcelFile object
excel_file = pd.ExcelFile('path/to/excel/file.xlsx')
Once you have an ExcelFile
object, you can use the sheet_names attribute to get a list of sheet names in the Excel file:
# Get the list of sheet names
sheet_names = excel_file.sheet_names
# Print the sheet names
print(sheet_names)
Output:
['Personal Information', 'City Statistics', 'Company Data', 'Product Inventory', 'Employee Feedback']
This will output a list of all the sheet names in the Excel file.
Now you can use read_excel()
function to get a Pandas DataFrame with the contents of the file. Here’s an example:
# Read Excel file into a Pandas DataFrame
df = pd.read_excel('path/to/excel/file.xlsx', sheet_name='Sheet1')
print(df)
Output:
Name Age Occupation
0 John 25 Data Scientist
1 Jane 30 Software Engineer
2 Bob 28 Analyst
Pros and Cons of Using Pandas to list of sheets in an excel file
Pros
Ease of Use: Pandas provides a straightforward and user-friendly interface for working with Excel files. Reading an Excel file into a DataFrame is a one-liner using
pd.read_excel()
.Flexibility: Pandas allows you to easily explore and manipulate the data within each sheet once loaded into a DataFrame. You can read specific sheets or the entire Excel file based on your requirements.
Efficiency: The
ExcelFile
class allows for more efficient handling of Excel files when working with multiple sheets, as it avoids reopening the file for each operation. Reading only the necessary sheets can save memory and processing time.**Integration with Other Pandas Features:**Pandas seamlessly integrates with other data analysis and manipulation functionalities, providing a comprehensive toolkit for data scientists and analysts.
Cons
Memory Usage: Loading large Excel files into memory can consume a significant amount of RAM, especially if you are working with multiple sheets simultaneously.
Limited Support for Complex Excel Features: Pandas might not support all the features of complex Excel files, such as macros, certain formulas, or specialized formatting.
**Not Ideal for Real-time Data:**If your Excel file is frequently updated and you need real-time data, Pandas may not be the best solution as it involves reading the file into memory.
Error Handling
- File Not Found: Handle cases where the specified Excel file path is incorrect or the file does not exist.
try:
df = pd.read_excel('path/to/excel/file.xlsx')
except FileNotFoundError:
print("File not found. Please provide a valid file path.")
- Sheet Name Not Found: If you are using a specific sheet name and it does not exist in the Excel file, handle the exception.
try:
df = pd.read_excel('path/to/excel/file.xlsx', sheet_name='Sheet1')
except pd.errors.XLRDError as e:
print(f"Error reading sheet: {e}")
- Insufficient Memory: In cases where loading the Excel file consumes too much memory, consider handling MemoryError by reading the file in chunks or optimizing memory usage.
try:
df = pd.read_excel('path/to/excel/file.xlsx', chunksize=1000)
except MemoryError:
print("Insufficient memory. Consider reading the file in smaller chunks.")
- General Exception Handling: Catching general exceptions can help capture unexpected errors.
try:
df = pd.read_excel('path/to/excel/file.xlsx')
sheet_names = df.sheet_names
print(sheet_names)
except Exception as e:
print(f"An unexpected error occurred: {e}")
Conclusion
In conclusion, Pandas provides a simple and efficient way to work with Excel files. To look up the list of sheets in an Excel file using Pandas, we can read the file into a Pandas DataFrame using the ExcelFile()
class and then use the sheet_names
attribute to get a list of all the sheets in the file. This is just one of many tasks that Pandas can help you accomplish when working with structured data. So, if you are a data scientist or software engineer working with Excel files, be sure to check out Pandas for your data manipulation needs.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.