Pandas Long to Wide Reshape A Data Scientists Guide
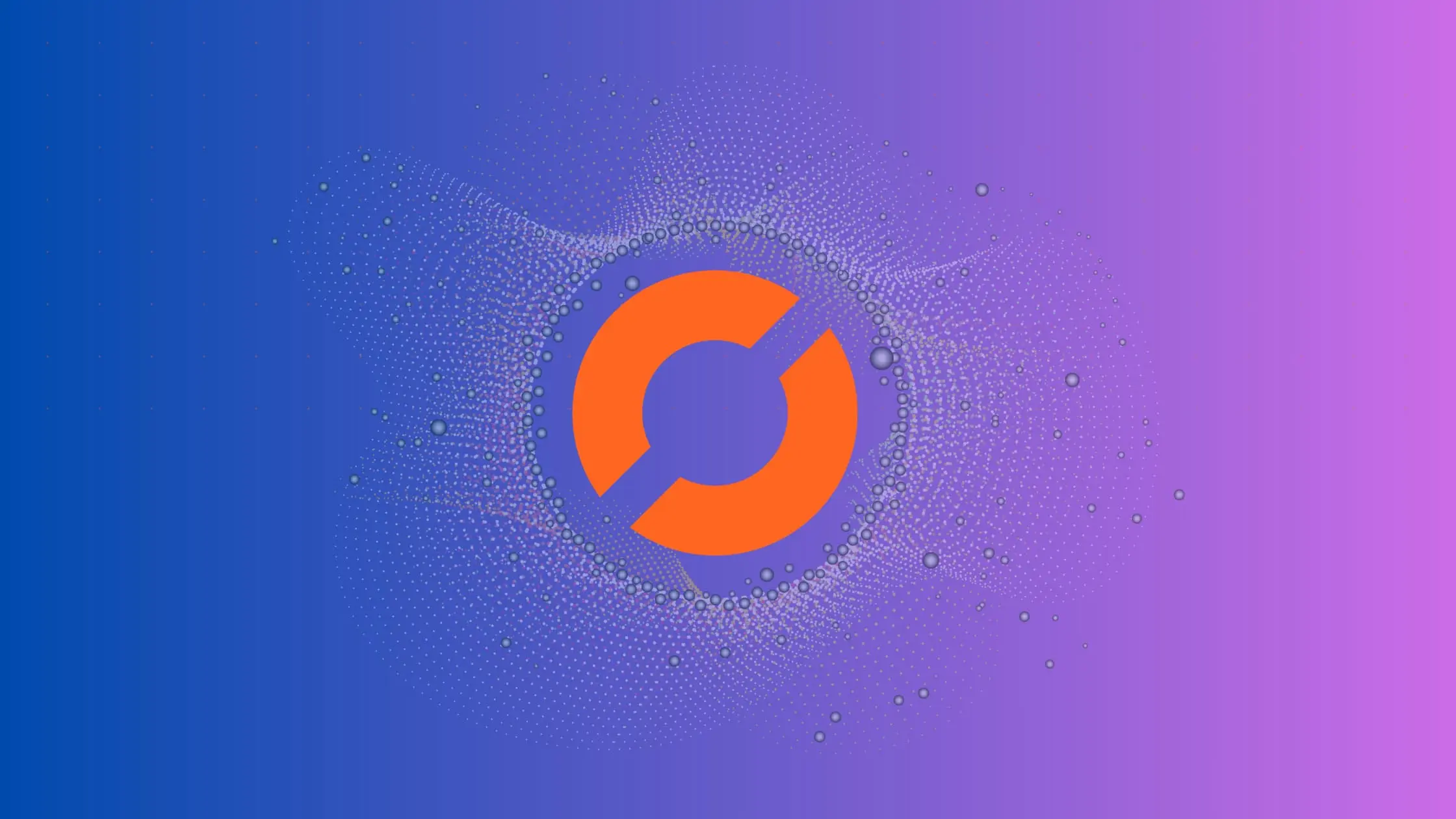
As a data scientist, you’re probably familiar with the concept of “tidy data.” Tidy data is a standard way of organizing data that makes it easy to work with. When data is in a tidy format, each variable is a column, each observation is a row, and each type of observational unit is a separate table. However, data often comes in a “messy” format, where variables are spread across multiple columns and rows. In this blog post, we’ll focus on how to reshape messy data from a long format to a wide format using Pandas.
Table of Contents
Long and Wide Format Explained 2.1 Long Format 2.2 Wide Format
Why Reshape Data? 3.1 Ease of Use 3.2 Visualization 3.3 Modeling 3.4 Compatibility
Pandas Long to Wide Reshape 4.1 Using
pivot()
4.1.1 Syntax 4.1.2 Example 4.2 Reshaping Data with Multiple Variables 4.2.1 Usingpivot_table()
4.2.2 Example
What is Long and Wide Format?
Before diving into the details of Pandas long to wide reshape, it’s important to understand what is meant by long format and wide format.
In long format, the data is organized such that each row represents a single observation, and each variable is represented by a separate column. This format is sometimes called “stacked” or “molten” data.
In wide format, each observation is still represented by a row, but each variable is represented by a separate column. This format is sometimes called “unstacked” or “cast” data.
Why Reshape Data?
There are several reasons why you might want to reshape data from long to wide format:
- Ease of use: Wide format can be easier to work with than long format, especially when dealing with large datasets or complex analyses.
- Visualization: Some types of visualizations are easier to create with wide format data.
- Modeling: Certain types of models require data in wide format.
- Compatibility: Some software packages or systems require data in wide format.
Pandas Long to Wide Reshape
Pandas is a popular Python library for data manipulation and analysis. It provides a variety of functions for reshaping data, including long to wide reshape. The pivot()
function is used for this purpose.
The pivot()
function takes three arguments: index
, columns
, and values
. The index
argument specifies the column(s) to use as the index of the resulting DataFrame. The columns
argument specifies the column(s) to use as the columns of the resulting DataFrame. The values
argument specifies the column(s) to use as the values of the resulting DataFrame.
Here’s an example of how to use pivot()
to reshape data from long to wide format:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({
'year': [2010, 2010, 2011, 2011],
'city': ['New York', 'Los Angeles', 'New York', 'Los Angeles'],
'population': [8175133, 3792621, 8491079, 3971883]
})
# reshape the DataFrame from long to wide format
df_wide = df.pivot(index='year', columns='city', values='population')
print(df_wide)
This code will output the following DataFrame:
city Los Angeles New York
year
2010 3792621 8175133
2011 3971883 8491079
In this example, the original DataFrame is in long format, with the year
and city
variables spread across multiple rows. The pivot()
function reshapes the data so that the year
variable becomes the index of the resulting DataFrame, the city
variable becomes the columns, and the population
variable becomes the values.
Reshaping Data with Multiple Variables
Sometimes you’ll need to reshape data with multiple variables. In this case, you can use the pivot_table()
function instead of pivot()
. The pivot_table()
function is similar to pivot()
, but it can handle multiple index and value columns.
Here’s an example of how to use pivot_table()
to reshape data with two variables:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({
'year': [2010, 2010, 2011, 2011],
'city': ['New York', 'Los Angeles', 'New York', 'Los Angeles'],
'measure': ['population', 'population', 'area', 'area'],
'value': [8175133, 3792621, 468.9, 1213.9]
})
# reshape the DataFrame from long to wide format
df_wide = df.pivot_table(index=['year', 'measure'], columns='city', values='value')
print(df_wide)
This code will output the following DataFrame:
city Los Angeles New York
year measure
2010 population 3792621.0 8175133.0
2011 area 1213.9 468.9
In this example, the original DataFrame has two variables, measure
and value
, in addition to year
and city
. The pivot_table()
function reshapes the data so that year
and measure
become the index of the resulting DataFrame, city
becomes the columns, and value
becomes the values.
Conclusion
Reshaping data from long to wide format is a common task in data science. Pandas provides a variety of functions for this purpose, including pivot()
and pivot_table()
. These functions make it easy to work with messy data and prepare it for analysis or visualization. By following these examples, you should be able to reshape your own data from long to wide format using Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.