Pandas How to concatenate dataframes with different columns
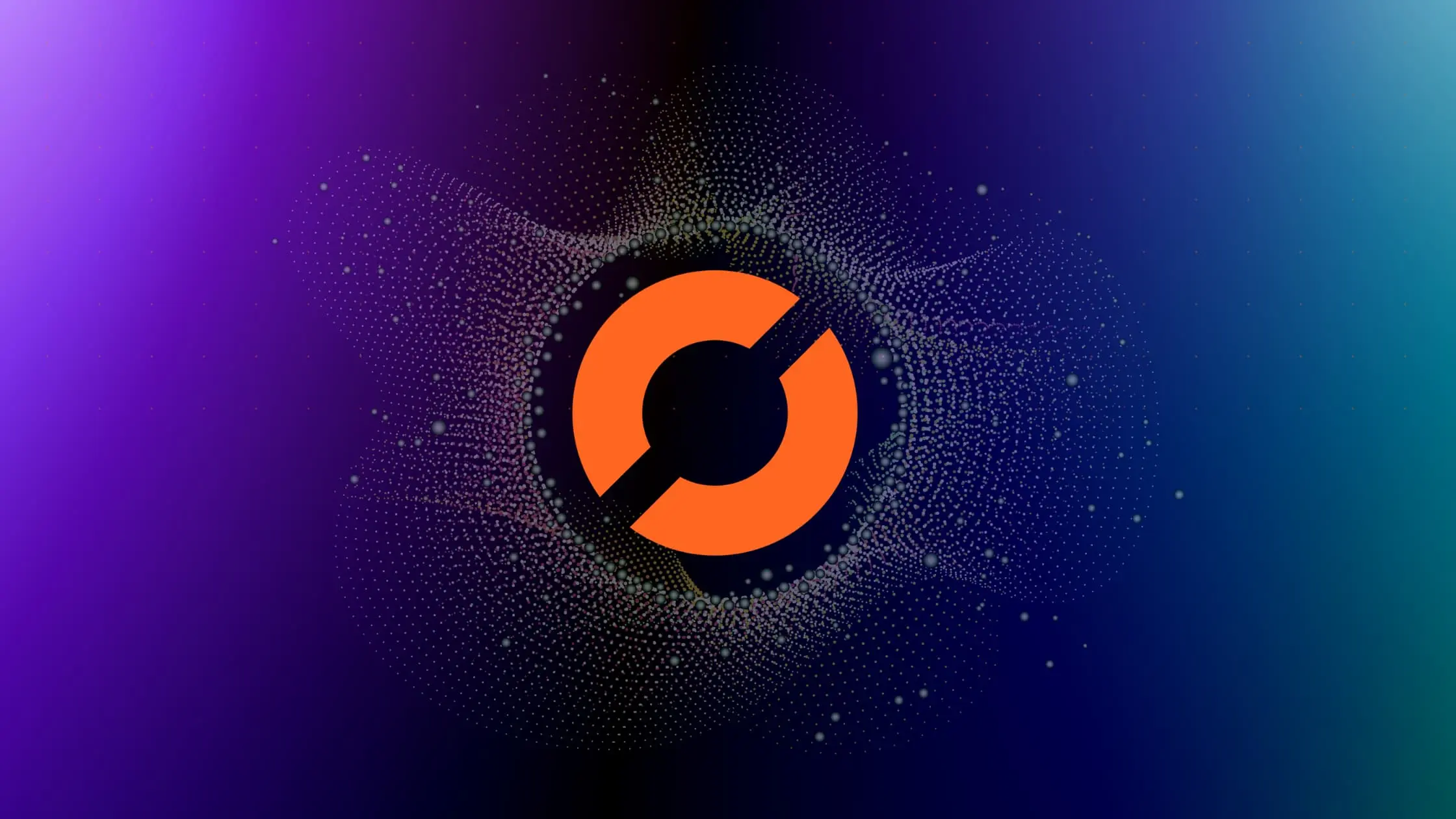
Pandas How to concatenate dataframes with different columns
As a data scientist or software engineer, you may have encountered a situation where you need to combine different dataframes into one. Concatenation is a common operation in data processing, and Pandas provides a function called concat()
that allows you to combine two or more dataframes.
However, concatenating dataframes with different columns can be a bit tricky. In this blog post, we will walk through how to concatenate dataframes with different columns using Pandas.
Why Concatenate Dataframes with Different Columns?
Before we dive into the technical details of concatenating dataframes with different columns, let’s first understand why we may need to do this in the first place.
One common scenario is when we have data from different sources that we want to combine into a single dataframe. For example, suppose we have two datasets, one containing information about customers' demographics and another containing their purchasing behavior. We may want to combine these two datasets to analyze how customer demographics relate to their purchasing behavior.
Another scenario is when we have data in different formats that we want to unify. For instance, suppose we have data in CSV format and Excel format. We may want to concatenate these two data sources to simplify our analysis.
In both cases, the dataframes may have different columns, and we need to concatenate them while preserving the information in each column.
Concatenating Dataframes with Different Columns
To concatenate dataframes with different columns, we use the concat()
function in Pandas. The concat()
function takes two or more dataframes as arguments and returns a new dataframe that combines them.
Here is the general syntax of the concat()
function:
pd.concat([df1, df2, df3, ...], axis=0, join='outer')
Let’s break down each argument:
[df1, df2, df3, ...]
: A list of dataframes to concatenate.axis
: Specifies the axis along which to concatenate.axis=0
means we concatenate along the rows (i.e., stack vertically), whileaxis=1
means we concatenate along the columns (i.e., stack horizontally).join
: Specifies how to handle columns that are not present in all dataframes.join='outer'
means we include all columns in the output dataframe, and missing values are filled with NaN.join='inner'
means we only include columns that are present in all dataframes.
When we concatenate dataframes with different columns, we need to specify the axis
argument carefully. If we concatenate along the rows, Pandas will align the columns based on their names. If a column is present in one dataframe but not in another, Pandas will fill the missing values with NaN. This approach can be useful when we want to combine data with different column names or data types.
On the other hand, if we concatenate dataframes along the columns, we need to ensure that the rows are aligned correctly. We also need to handle duplicate columns and missing values carefully.
Example: Concatenating Dataframes with Different Columns
Let’s illustrate the process of concatenating dataframes with different columns using an example. Suppose we have two dataframes, df1
and df2
, with different columns:
import pandas as pd
# create first dataframe
df1 = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'gender': ['F', 'M', 'M']
})
# create second dataframe
df2 = pd.DataFrame({
'name': ['Alice', 'Bob', 'Dave'],
'income': [50000, 60000, 70000],
'occupation': ['Engineer', 'Manager', 'Analyst']
})
Here is what df1
and df2
look like:
name age gender
0 Alice 25 F
1 Bob 30 M
2 Charlie 35 M
name income occupation
0 Alice 50000 Engineer
1 Bob 60000 Manager
2 Dave 70000 Analyst
Suppose we want to concatenate these two dataframes along the rows and include all columns. We can use the following code:
# concatenate dataframes along the rows
result = pd.concat([df1, df2], axis=0, join='outer')
Here is what the result
dataframe looks like:
name age gender income occupation
0 Alice 25 F NaN NaN
1 Bob 30 M NaN NaN
2 Charlie 35 M NaN NaN
0 Alice NaN NaN 50000 Engineer
1 Bob NaN NaN 60000 Manager
2 Dave NaN NaN 70000 Analyst
As you can see, Pandas has concatenated the dataframes along the rows and filled in missing values with NaN. The resulting dataframe includes all columns from both dataframes.
Suppose we want to concatenate these two dataframes along the columns. We can use the following code:
# concatenate dataframes along the columns
result = pd.concat([df1, df2], axis=1, join='outer')
Here is what the result
dataframe looks like:
name age gender name income occupation
0 Alice 25 F Alice 50000 Engineer
1 Bob 30 M Bob 60000 Manager
2 Charlie 35 M Dave 70000 Analyst
As you can see, Pandas has concatenated the dataframes along the columns and aligned the rows based on their indexes. The resulting dataframe includes all columns from both dataframes, and duplicate columns are appended with a suffix.
Conclusion
In this blog post, we have learned how to concatenate dataframes with different columns using Pandas. We have seen that Pandas provides a concat()
function that allows us to combine two or more dataframes. We have also seen that we need to specify the axis
and join
arguments carefully when concatenating dataframes with different columns.
Concatenating dataframes with different columns can be a useful technique for combining data from different sources or in different formats. By understanding how to concatenate dataframes with different columns, you can simplify your data processing workflows and gain new insights from your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.