Pandas Get Day of Week from Date Type Column
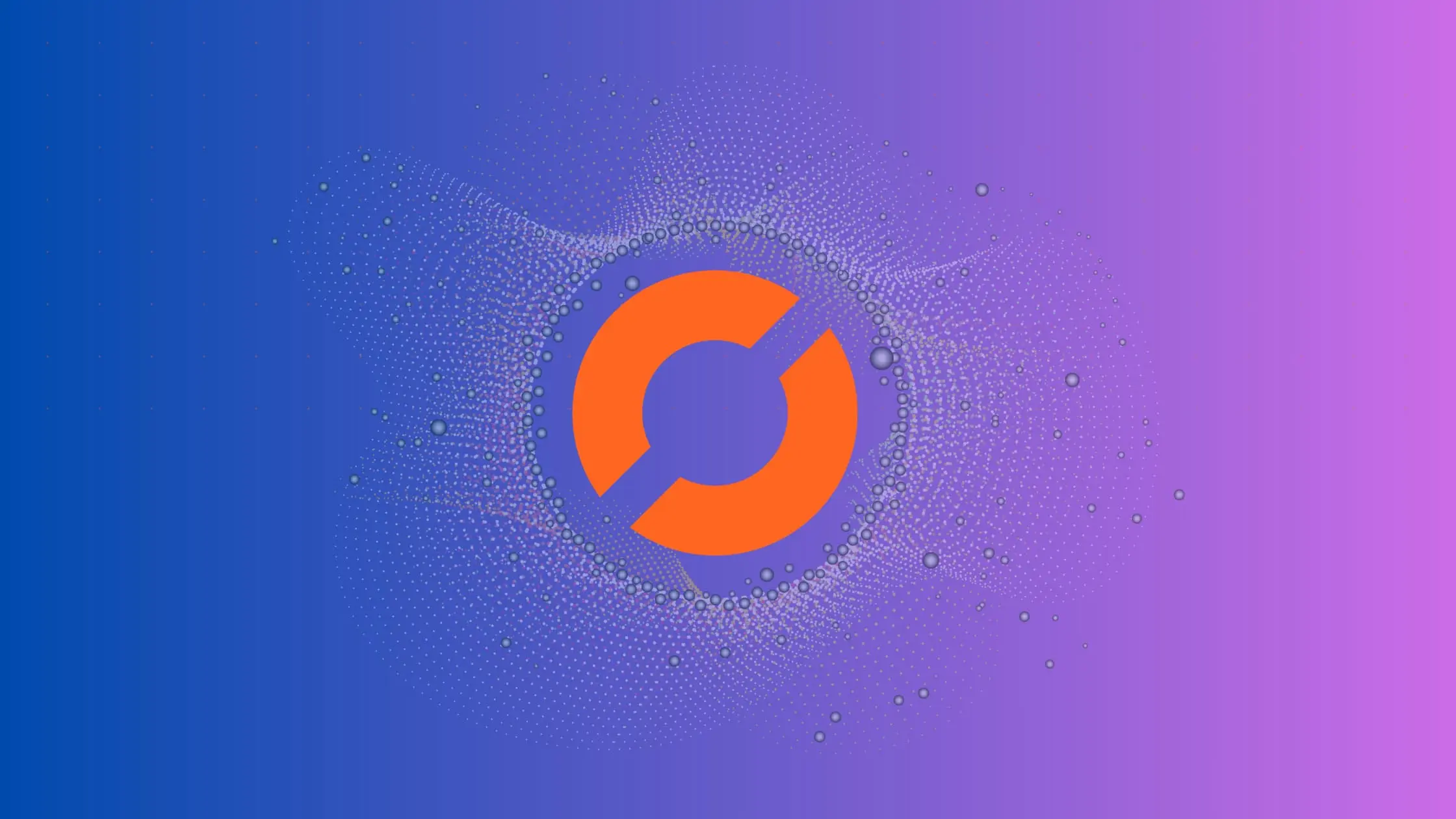
As a data scientist or software engineer, you will often encounter datasets that contain date and time information. In many cases, you will need to extract specific information from these dates, such as the day of the week. In this article, we will explore how to extract the day of the week from a date type column in a Pandas DataFrame.
Table of Contents
- What is Pandas?
- How to Extract the Day of the Week from a Date Type Column in Pandas
- Common Errors and Solutions
- Best Practices
- Conclusion
What is Pandas?
Pandas is a popular Python library for data manipulation and analysis. It provides easy-to-use data structures and data analysis tools for handling tabular data, time series, and more. Pandas is widely used in data science and machine learning for data preprocessing and data wrangling.
How to Extract the Day of the Week from a Date Type Column in Pandas
To extract the day of the week from a date type column in a Pandas DataFrame, we can use the dt
accessor. The dt
accessor provides access to several datetime properties and methods, including the day_name()
method, which returns the name of the day of the week.
Let’s start by creating a sample DataFrame with a date type column:
import pandas as pd
df = pd.DataFrame({
'date': ['2021-06-01', '2021-06-02', '2021-06-03', '2021-06-04', '2021-06-05']
})
df['date'] = pd.to_datetime(df['date'])
In this example, we create a DataFrame with a date
column containing five dates. We then convert the date
column to a datetime type using the to_datetime()
method.
Method 1: Using day_name()
method
To extract the day of the week from the date
column, we can use the day_name()
method:
df['day_of_week'] = df['date'].dt.day_name()
print(df)
This will create a new column called day_of_week
in the DataFrame, containing the day of the week for each date in the date
column.
Output:
date day_of_week
0 2021-06-01 Tuesday
1 2021-06-02 Wednesday
2 2021-06-03 Thursday
3 2021-06-04 Friday
4 2021-06-05 Saturday
In addition to using the dt.day_name()
method, Pandas provides alternative methods to extract the day of the week from a date type column.
Method 2: Using dt.weekday
Method:
Another approach is to use the dt.weekday
method, which returns the day of the week as an integer (Monday is 0 and Sunday is 6). You can then map these integers to the corresponding day names.
df['day_of_week'] = df['date'].dt.weekday.map({0: 'Monday', 1: 'Tuesday', 2: 'Wednesday', 3: 'Thursday', 4: 'Friday', 5: 'Saturday', 6: 'Sunday'})
Output:
date day_of_week
0 2021-06-01 Tuesday
1 2021-06-02 Wednesday
2 2021-06-03 Thursday
3 2021-06-04 Friday
4 2021-06-05 Saturday
Method 3: Using dt.strftime
Method:
The dt.strftime
method allows you to format the date as a string, and you can use the %A
directive to get the full weekday name.
df['day_of_week'] = df['date'].dt.strftime('%A')
This method provides flexibility in customizing the output format.
Output:
date day_of_week
0 2021-06-01 Tuesday
1 2021-06-02 Wednesday
2 2021-06-03 Thursday
3 2021-06-04 Friday
4 2021-06-05 Saturday
Common Errors and Solutions
Error: "AttributeError: Can only use .dt accessor with datetimelike values."
This error occurs when the column is not converted to datetime type before applying the dt
accessor. Ensure that you have converted the column to datetime using pd.to_datetime()
.
# Generate the error
df['day_of_week'] = df['date'].dt.day_name() # This will raise an AttributeError if 'date' is not a datetime type column.
Solution:
# Convert the column to datetime before using dt accessor
df['date'] = pd.to_datetime(df['date'])
df['day_of_week'] = df['date'].dt.day_name()
Best Practices
Consistent Date Format:
Ensure consistency in the date format within the date column. This helps prevent errors during conversion and manipulation.
Handling Missing Values:
Check for and handle missing values in the date column before performing operations. Use df.dropna(subset=['date'])
to remove rows with missing dates.
Conclusion
In this article, we have explored how to extract the day of the week from a date type column in a Pandas DataFrame. We have seen how the dt
accessor can be used to access datetime properties and methods, including the day_name()
method for extracting the day of the week.
As a data scientist or software engineer, it is important to have a good understanding of data manipulation tools like Pandas in order to efficiently handle and analyze large datasets. Knowing how to extract specific information from date and time data is a valuable skill that can help you gain insights into your data and make better-informed decisions.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.