Pandas Filtering Multiple Conditions
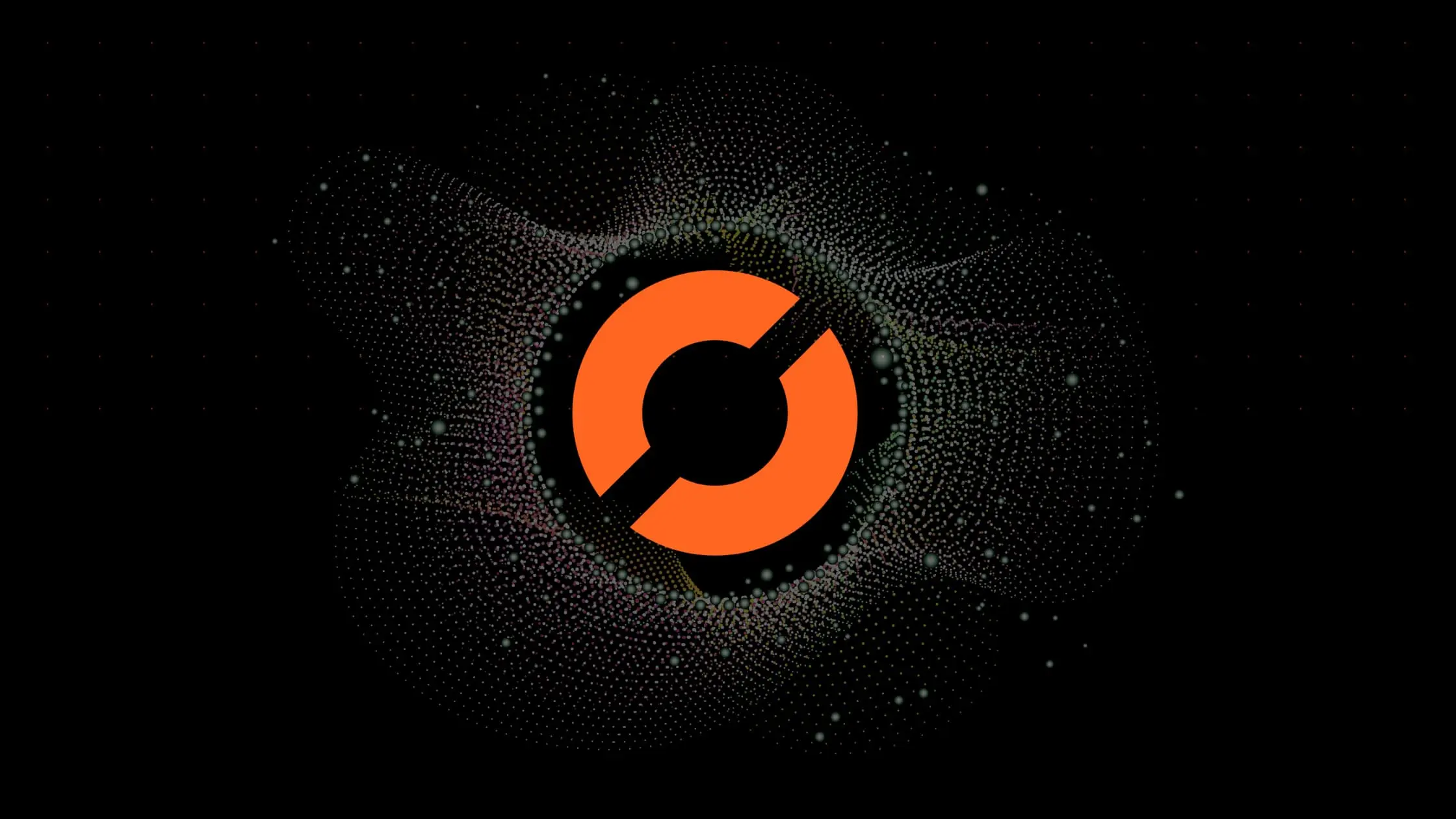
As a data scientist or software engineer, you may need to filter data based on multiple conditions to extract meaningful insights. Pandas, a popular data analysis library in Python, provides powerful tools for filtering data based on multiple conditions. In this article, we will explore how to filter data based on multiple conditions using Pandas.
Table of Contents
- Introduction
- What is Pandas?
- How to Filter Data based on Multiple Conditions using Pandas?
- Error Handling
- Conclusion
What is Pandas?
Pandas is a popular open-source data analysis library in Python. It provides data structures and functions for data manipulation, analysis, and visualization. Pandas is widely used in data science, machine learning, and artificial intelligence applications for cleaning, transforming, and analyzing data.
How to Filter Data based on Multiple Conditions using Pandas?
Pandas provides several functions for filtering data based on multiple conditions. The most commonly used functions are loc
and iloc
. The loc
function is used to filter data based on labels, while the iloc
function is used to filter data based on integer positions. In this article, we will focus on the loc
function.
Filtering Data based on Multiple Conditions using the loc function
The loc
function is used to filter data based on labels. It accepts one or more Boolean conditions that evaluate to True or False. The Boolean conditions are combined using logical operators such as &
(and) and |
(or) to filter data based on multiple conditions.
Let’s consider an example to understand how to filter data based on multiple conditions using the loc
function. Suppose we have a Pandas DataFrame df
that contains information about students' grades in different subjects.
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emily'],
'Math': [85, 76, 92, 68, 91],
'Science': [78, 92, 88, 76, 85],
'English': [80, 85, 82, 79, 88]}
df = pd.DataFrame(data)
The DataFrame df
looks like this:
Name | Math | Science | English | |
---|---|---|---|---|
0 | Alice | 85 | 78 | 80 |
1 | Bob | 76 | 92 | 85 |
2 | Charlie | 92 | 88 | 82 |
3 | David | 68 | 76 | 79 |
4 | Emily | 91 | 85 | 88 |
Suppose we want to filter the DataFrame df
to extract the rows where a student’s grade in Math is greater than 80 and their grade in Science is greater than 85. We can use the following code to achieve this:
condition1 = df['Math'] > 80
condition2 = df['Science'] > 85
filtered_df = df.loc[condition1 & condition2]
In the above code, we first define two Boolean conditions condition1
and condition2
using the column labels Math
and Science
. We then combine these conditions using the &
operator to create a single Boolean condition. Finally, we use the loc
function to filter the DataFrame df
based on this Boolean condition.
The resulting filtered DataFrame looks like this:
Name | Math | Science | English | |
---|---|---|---|---|
2 | Charlie | 92 | 88 | 82 |
We can see that the filtered DataFrame contains only one row that satisfies both conditions.
Filtering Data based on Multiple Conditions using the query function
Pandas also provides a query
function to filter data based on multiple conditions. The query
function accepts a string that contains the Boolean conditions to filter the data. The Boolean conditions are specified using the column labels enclosed in backticks () and combined using logical operators such as
andand
or`.
Let’s consider the same example as before and use the query
function to filter the DataFrame df
based on multiple conditions.
filtered_df = df.query('Math > 80 and Science > 85')
In the above code, we use the query
function to filter the DataFrame df
based on the Boolean condition Math > 80 and Science > 85
. The resulting filtered DataFrame is the same as before.
Error Handling
- Check DataFrame Structure: Before applying conditions, ensure that the DataFrame has the expected structure. Add a check to verify the existence of required columns (‘Math’, ‘Science’, etc.):
required_columns = ['Name', 'Math', 'Science', 'English']
if not all(column in df.columns for column in required_columns):
raise ValueError("DataFrame is missing required columns.")
- Handle Empty DataFrames: If the DataFrame is empty after filtering, it may cause issues in downstream operations. Check for an empty DataFrame and handle it appropriately:
if filtered_df.empty:
print("No rows satisfy the given conditions.")
# or raise an exception based on the specific use case
- Ensure Numeric Values for Filtering: Check if the columns used for filtering contain numeric values. This is crucial to prevent unexpected errors:
numeric_columns = ['Math', 'Science']
if not all(df[column].dtype == 'int64' for column in numeric_columns):
raise TypeError("Numeric values are required for filtering.")
- Handle Missing Values: If there are missing values in the DataFrame, they might interfere with filtering. Consider handling or removing missing values before applying conditions:
if df.isnull().any().any():
df = df.dropna() # or handle missing values based on the specific use case
Conclusion
Filtering data based on multiple conditions is a common task in data analysis and data science. Pandas provides powerful tools for filtering data based on multiple conditions using the loc
and query
functions. By using these functions, you can easily extract meaningful insights from your data based on complex filtering criteria.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.