Pandas every nth row A Guide for Data Scientists
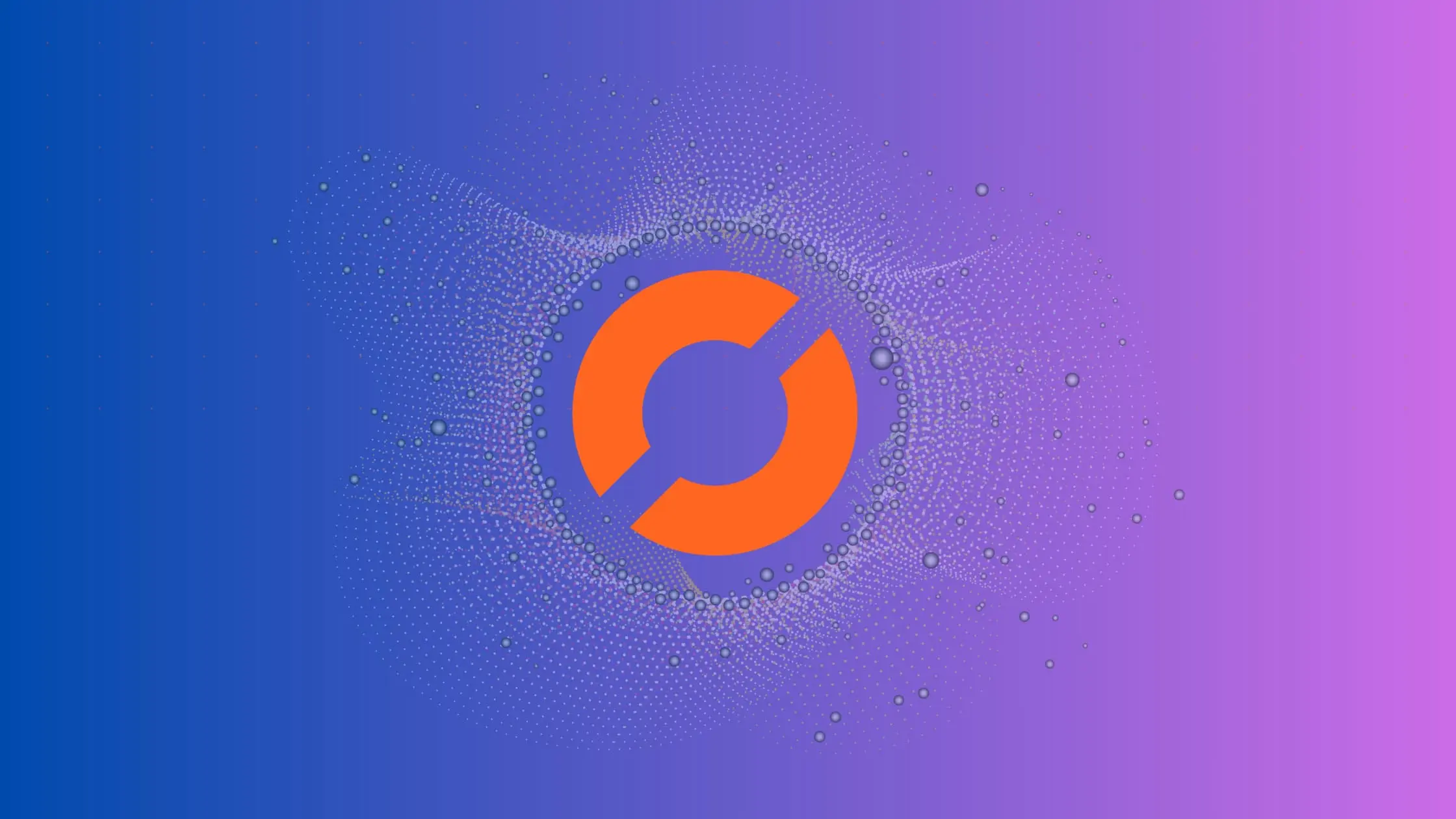
As a data scientist, you know that working with large datasets can be a challenging task. One of the most common problems that data scientists face is the need to filter data based on specific criteria. In this article, we will explore how to use Pandas to filter every nth row from a dataset.
Table of Contents
- Introduction to Pandas
- Filtering every nth row using Pandas
- Pros and Cons of each Method
- Common Errors and How to Handle
- Conclusion
Introduction to Pandas
Pandas is a popular Python library used for data manipulation and analysis. It provides various data structures like data frames and series to store and manipulate data. Pandas is widely used in data science and machine learning projects.
Filtering every nth row using Pandas
Using iloc()
Filtering every nth row from a dataset can be useful in many scenarios. For example, you may want to sample a subset of the data or extract only specific rows based on a certain criterion. Fortunately, Pandas provides an easy way to filter every nth row using the iloc
method.
The iloc
method allows you to select rows and columns by their integer positions. You can use the iloc
method to select every nth row from a dataset by specifying the start, stop, and step parameters.
import pandas as pd
# Sample DataFrame
data = {'Column1': range(1, 11)}
df = pd.DataFrame(data)
# Select every 2nd row using iloc
every_nth_row = df.iloc[::2]
print(every_nth_row)
The above code will select every 2nd row starting from the 0th row and output the following:
A B
0 1 11
2 3 13
4 5 15
6 7 17
8 9 19
You can change the start and stop parameters to select a specific range of rows. For example, to select every 2nd row starting from the 1st row and ending at the 5th row, you can modify the code as follows:
# select every 2nd row starting from the 1st row and ending at the 5th row
df.iloc[1:6:2]
The above code will select every 2nd row between the 1st and 5th row and output the following:
A B
1 2 12
3 4 14
5 6 16
Using Modulo Operator to Select Every nth Row
Another approach is to use the modulo operator (%) to filter rows based on their index.
import pandas as pd
# create a sample dataframe
df = pd.DataFrame({'A': range(1, 11), 'B': range(11, 21)})
# Select every 2nd row using modulo
every_nth_row_modulo = df[df.index % 2 == 0]
print(every_nth_row_modulo)
Output:
A B
0 1 11
2 3 13
4 5 15
6 7 17
8 9 19
Pros and Cons of each Method
Pros and Cons of iloc()
Pros
- Simple and intuitive syntax: The iloc method provides an easy-to-understand syntax for selecting every nth row.
- Efficiency with Numeric Index: This method is highly efficient when working with DataFrames having a numeric index.
Cons
- Limited Flexibility: The iloc method may not be as flexible when dealing with DataFrames that have a non-integer index or irregularly spaced index values.
- Potential Inefficiency: It may not be the most efficient choice for very large datasets compared to other methods.
Pros and Cons of Modulo Operator
Pros:
- Flexibility with Index Types: Works well with both numeric and non-numeric indices, providing adaptability for diverse datasets.
- Custom Conditions: Enables selection of rows based on custom conditions, offering greater flexibility.
Cons:
- Complex Syntax: The modulo method may have a slightly more complex syntax compared to the straightforward iloc approach.
- Potential Inefficiency: Depending on the size of the DataFrame, this method might be less efficient for very large datasets.
Common Errors and How to Handle
Incorrect Syntax:
# Incorrect Syntax
every_nth_row = df.iloc[::n]
To avoid this error, make sure that n
is a valid integer.
Incorrect Condition
# Incorrect Condition
every_nth_row_modulo = df[df.index % n == 0]
To avoid this error, ensure the use of ==
to check for equality in the condition.
Conclusion
In this article, we explored how to use Pandas to filter every nth row from a dataset. Pandas provides an easy way to filter rows based on specific criteria using the iloc
method. By specifying the start, stop, and step parameters, you can select every nth row from a dataset. This technique can be useful in many scenarios, such as sampling a subset of the data or extracting specific rows based on a certain criterion. With the help of Pandas, data scientists can easily manipulate and analyze large datasets.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.