Pandas Convert Timestamp to datetimedate
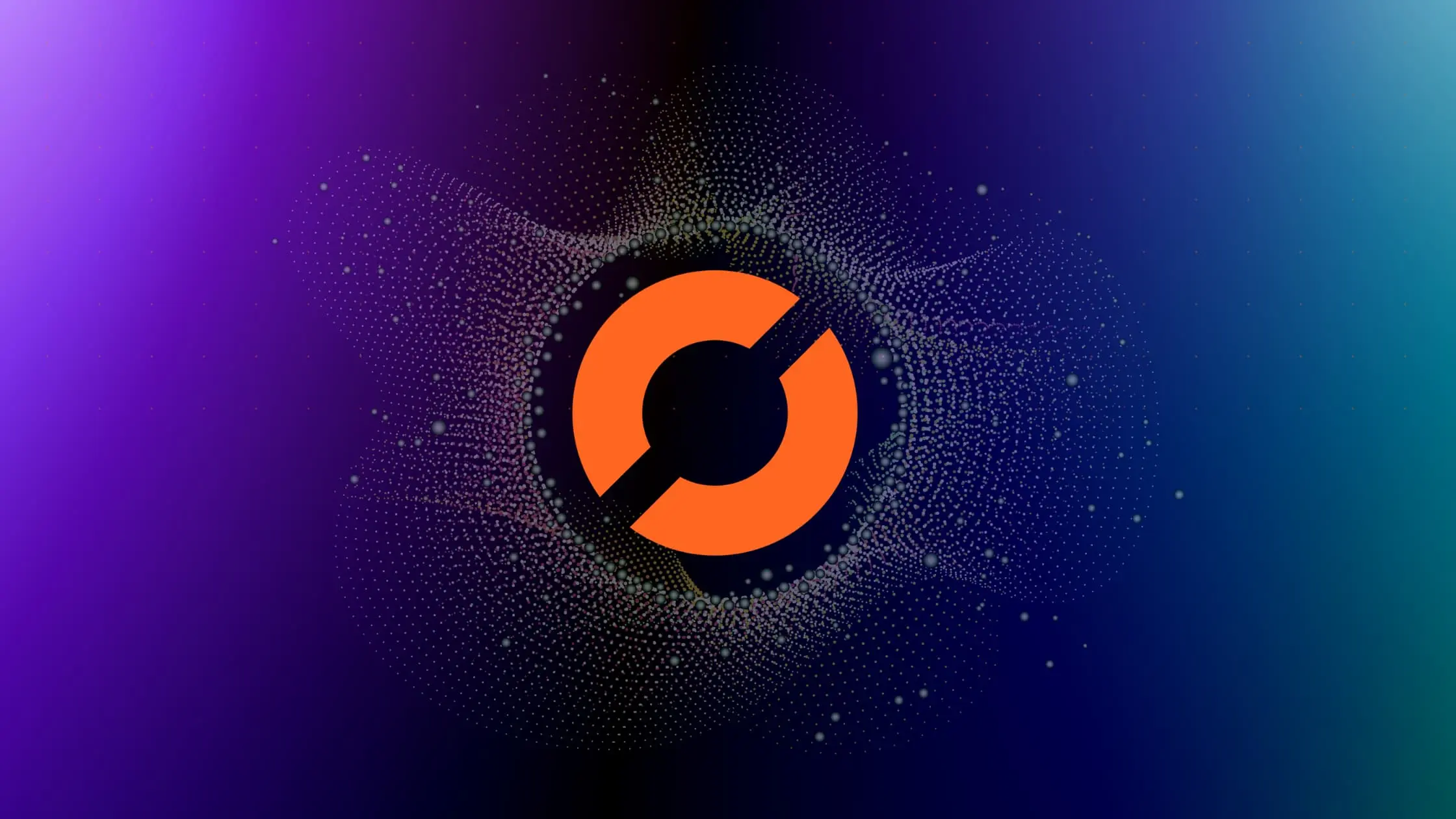
Pandas Convert Timestamp to datetimedate
As a data scientist or software engineer, you may often encounter datasets that contain timestamps. These timestamps can be in different formats, such as Unix time, ISO 8601, or custom formats. In Python, the Pandas library provides powerful tools to manipulate and transform time-series data.
One common task when working with timestamps is converting them to date objects. This can be useful when you want to perform date-based calculations or group data by date. In this article, we will show you how to use Pandas to convert timestamps to datetime.date objects.
What is a Timestamp?
Before we dive into the details of converting timestamps to date objects, let’s first define what a timestamp is. In general, a timestamp is a sequence of characters or binary digits that represents a specific point in time. In the context of data analysis, timestamps are often used to represent the time at which an event occurred, such as a purchase, a website visit, or a sensor reading.
In Pandas, timestamps are represented using the Timestamp
class, which is a subclass of the Python datetime.datetime
class. The Timestamp
class provides additional functionality for working with time-series data, such as time zone handling, date arithmetic, and resampling.
Converting Timestamps to datetime.date Objects
To convert a Pandas Timestamp
object to a Python datetime.date
object, you can use the date()
method of the Timestamp
class. This method returns a new datetime.date
object that represents the same date as the Timestamp
object.
import pandas as pd
# Create a Pandas Timestamp object
ts = pd.Timestamp('2022-06-18 12:34:56')
# Convert the Timestamp to a Python datetime.date object
date = ts.date()
print(date)
Output:
2022-06-18
In this example, we first create a Timestamp
object using the pd.Timestamp()
function and passing a string in the ISO 8601 format. We then call the date()
method of the Timestamp
object to obtain a datetime.date
object that represents the same date.
Note that the date()
method returns a new datetime.date
object and does not modify the original Timestamp
object.
Handling Time Zones
When working with timestamps, it’s important to consider time zones. A timestamp represents a specific point in time, but the actual time represented by the timestamp may be different depending on the time zone.
In Pandas, timestamps can be associated with time zones using the tz
parameter. When a timestamp has a time zone, converting it to a date object requires additional steps to ensure that the correct date is obtained.
import pandas as pd
import pytz
# Create a Pandas Timestamp object with timezone information
ts = pd.Timestamp('2022-06-18 12:34:56', tz='US/Pacific')
# Convert the Timestamp to a Python datetime.date object in the same timezone
date = ts.tz_convert('US/Pacific').date()
print(date)
# Format the datetime object as a string including date and time to see the hour changed
formatted_datetime = dt.strftime('%Y-%m-%d %H:%M:%S %Z')
print(formatted_datetime)
Output:
2022-06-18
2022-06-18 20:34:56 BST
In this example, we create a Timestamp
object with time zone information using the tz
parameter. We then use the tz_convert()
method to convert the timestamp to the same time zone before calling the date()
method to obtain the date object.
If you want to convert the timestamp to a date object in a different time zone, you can pass the desired time zone to the tz_convert()
method.
import pandas as pd
import pytz
# Create a Pandas Timestamp object with timezone information
ts = pd.Timestamp('2022-06-18 12:34:56', tz='US/Pacific')
# Convert the Timestamp to a Python datetime.date object in a different timezone
date = ts.tz_convert('Europe/London').date()
print(date)
Output:
2022-06-18
In this example, we convert the Timestamp
object to the Europe/London
time zone before calling the date()
method to obtain the date object.
Conclusion
In this article, we have shown you how to use Pandas to convert timestamps to datetime.date
objects. We have also discussed how to handle time zones when converting timestamps to date objects.
Converting timestamps to date objects is a common task when working with time-series data. By using Pandas, you can easily manipulate and transform time-series data in Python.
We hope this article has been helpful in your data analysis or software engineering endeavors. If you have any questions or comments, feel free to leave them below!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.