Pandas Convert Column to List
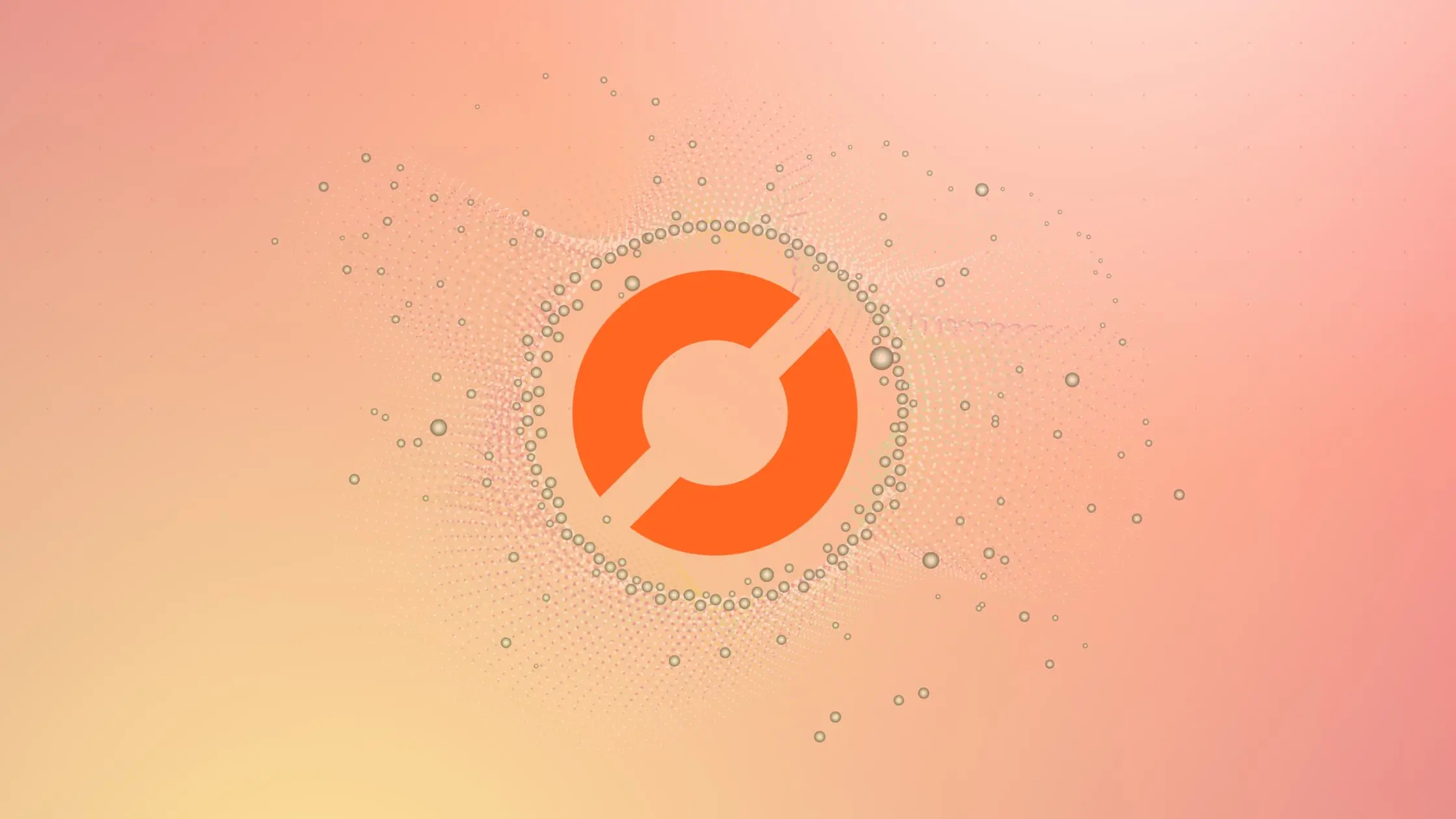
As a data scientist or software engineer, you may often work with datasets that require manipulation and transformation. One common task is converting a column of a Pandas DataFrame into a list. This can be useful for various purposes, such as passing the data to a function or using it for visualization. In this article, we will explore a simple way to convert a column to a list using Pandas.
What is Pandas?
Pandas is an open-source library for data manipulation and analysis in Python. It provides data structures for efficiently handling large datasets, such as Series and DataFrame, which are designed to handle tabular data. Pandas is widely used for data cleaning, transformation, and analysis tasks in various domains, including finance, healthcare, and engineering.
Why Convert a Column to a List?
Converting a Pandas DataFrame column to a Python list is a common operation in data analysis and manipulation tasks. There are several reasons why you might want to do this:
Interoperability: You might need to work with other Python libraries or modules that expect data in list format. Converting a Pandas column to a list makes it easier to pass the data to these external functions or libraries.
Simpler Iteration: Lists are easier to work with when iterating through the data in a column, whether it’s for computation, visualization, or any other data processing task.
Functional Programming: Some Python libraries or functional programming paradigms favor working with lists as they offer more flexibility and versatility.
Converting a Column to a List
The easiest way to convert a column of a Pandas DataFrame to a list is to use the tolist()
method. Let’s see an example:
import pandas as pd
# create a DataFrame
data = {'name': ['John', 'Alice', 'Bob'], 'age': [25, 30, 35]}
df = pd.DataFrame(data)
# convert the 'name' column to a list
name_list = df['name'].tolist()
print(name_list)
This is the simplest and most straightforward way to convert a Pandas DataFrame column to a list. This method can be applied to a Pandas Series, which represents a single column in a DataFrame.
Output:
['John', 'Alice', 'Bob']
Conclusion
Converting a Pandas DataFrame column to a list is a simple task with several methods at your disposal. Whether you choose to use the .tolist()
method, the .values
attribute, or list comprehension, the choice depends on your specific needs and coding style.
These methods enable you to extract data from Pandas DataFrames for various purposes, such as further analysis, visualization, or integration with other Python libraries.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.