Multivariate Polynomial Regression with Python
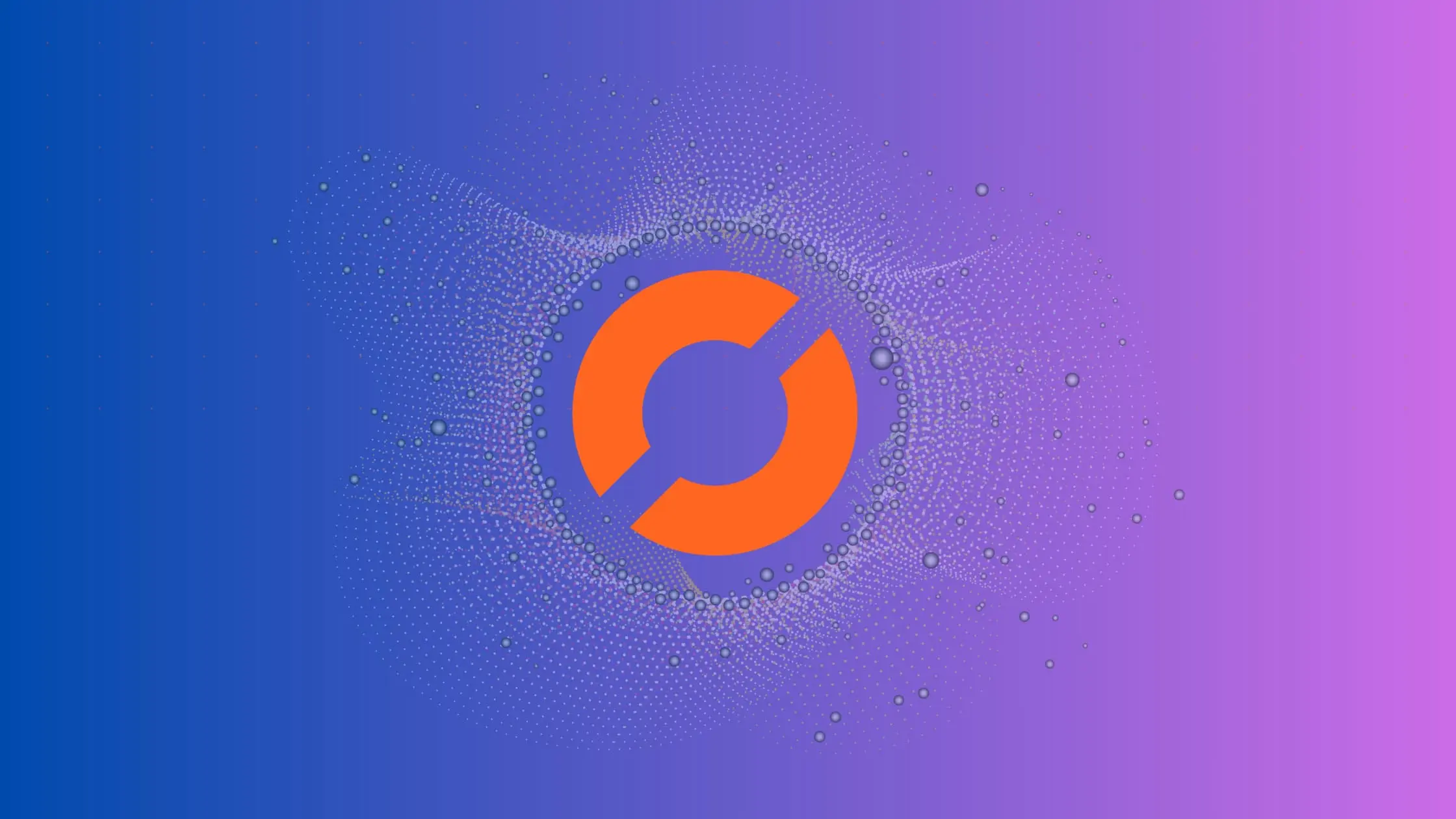
If you’re a data scientist or software engineer, you’ve likely encountered a problem where a linear regression model doesn’t quite fit the data. In such cases, multivariate polynomial regression can be a powerful tool to capture more complex relationships between variables. In this post, we’ll explore how to implement multivariate polynomial regression in Python using the scikit-learn library.
Table of Contents
- Introduction
- What is Multivariate Polynomial Regression?
- How to Implement Multivariate Polynomial Regression
- Step 1: Import Libraries
- Step 2: Load the Data
- Step 3: Create the Feature Matrix and Target Vector
- Step 4: Generate Polynomial Features
- Step 5: Fit the Model
- Step 6: Make Predictions
What is Multivariate Polynomial Regression?
Multivariate polynomial regression is an extension of linear regression that allows for multiple input variables and non-linear relationships between the input variables and the target variable. In a multivariate polynomial regression model, the input variables are raised to different powers, creating a polynomial equation. The coefficients of the polynomial equation are determined using a least-squares optimization process, just like in linear regression.
How to Implement Multivariate Polynomial Regression
To implement multivariate polynomial regression in Python, we’ll use the scikit-learn library, which provides a range of machine learning algorithms and tools. Here are the steps to implement multivariate polynomial regression in Python:
Step 1: Import Libraries
import numpy as np
import pandas as pd
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import PolynomialFeatures
We’ll need the NumPy and pandas libraries for data manipulation, the scikit-learn’s LinearRegression class to perform the regression, and the PolynomialFeatures class to generate the polynomial features.
Step 2: Load the Data
We’ll use a sample dataset from scikit-learn to demonstrate multivariate polynomial regression. The dataset contains information about the California housing market, including the price of the house and various features such as crime rate, number of rooms, and distance from the city center. Here’s how to load the data:
from sklearn.datasets import fetch_california_housing
# Load the California housing dataset
california = fetch_california_housing()
df = pd.DataFrame(california.data, columns=california.feature_names)
df['PRICE'] = california.target
Step 3: Create the Feature Matrix and Target Vector
We’ll create the feature matrix X and the target vector y from the loaded data:
# Select appropriate features from the dataset
# Assuming you want to use features like 'MedInc' (median income), 'HouseAge', and 'AveRooms' (average rooms)
X = df[['MedInc', 'HouseAge', 'AveRooms']].values
y = df['PRICE'].values
Step 4: Generate Polynomial Features
Next, we’ll generate the polynomial features using the PolynomialFeatures class:
# Polynomial features
poly = PolynomialFeatures(degree=2)
X_poly = poly.fit_transform(X)
The degree parameter specifies the degree of the polynomial equation. In this case, we’ve set it to 2, which means that we’ll generate a quadratic equation.
Step 5: Fit the Model
Now, we’ll fit the multivariate polynomial regression model using the LinearRegression class:
# Linear Regression model
model = LinearRegression()
model.fit(X_poly, y)
The model is now trained and can be used to make predictions.
Step 6: Make Predictions
To make predictions using the trained model, we’ll first generate the polynomial features for new data:
# New data with median income, house age, and average number of rooms
new_data = np.array([[3, 20, 5]]) # Example values for median income, house age, and average rooms
new_data_poly = poly.transform(new_data)
Then, we’ll use the predict() method of the model to make predictions:
# Predicting the price
predicted_price = model.predict(new_data_poly)
print(predicted_price)
Output:
[1.54330623]
The model output [1.54330623]
is the predicted price (in hundreds of thousands of dollars, which is a common scale in the California housing dataset) for a house with the specified features (median income of 3, house age of 20 years, and average of 5 rooms). This means the model predicts that a house with these characteristics would cost approximately $154,330.62 (since the dataset typically represents prices in $100,000s).
Pros and Cons of Multivariate Polynomial Regression
Pros
Captures Non-Linear Relationships: Multivariate polynomial regression is effective in capturing complex and non-linear relationships between input variables and the target variable. This makes it a valuable tool when linear regression models fall short.
Flexibility: The degree of the polynomial equation can be adjusted to control the complexity of the model. Higher degrees allow the model to fit more intricate patterns in the data.
Utilizes Linear Regression Framework: The implementation builds upon the linear regression framework, leveraging the familiar concepts of least-squares optimization. This makes it easier for practitioners already familiar with linear regression to transition to multivariate polynomial regression.
Widely Supported Libraries: The availability of libraries like scikit-learn simplifies the implementation process, allowing users to access a variety of machine learning tools for data manipulation and model development.
Cons
Overfitting Risk: As the degree of the polynomial increases, the model becomes more prone to overfitting, capturing noise in the data rather than the underlying patterns. Care must be taken to select an appropriate degree to balance model complexity and generalization.
Computational Intensity: Higher degrees of polynomial features can lead to a significant increase in the computational complexity of the model. This may result in longer training times and increased resource requirements.
Interpretability Challenges: While polynomial regression models provide accurate predictions, they often lack the interpretability of simpler models. Understanding the impact of individual features on the target variable becomes more challenging as the model complexity grows.
Error Handling
Data Quality Check: Before implementing the model, it’s essential to check and handle any missing or inconsistent data. Missing values or outliers can significantly impact the performance of the model.
Feature Selection: Carefully choose the input features based on domain knowledge and relevance. Including irrelevant or redundant features may lead to suboptimal model performance.
Hyperparameter Tuning: Experiment with different degrees of the polynomial (hyperparameter tuning) to find the optimal complexity for the model. Use techniques like cross-validation to evaluate the model’s performance on different subsets of the data.
Regularization Techniques: Consider incorporating regularization techniques like Ridge or Lasso regression to prevent overfitting, especially when dealing with high-degree polynomials.
Monitoring Model Performance: Continuously monitor the model’s performance on new data and be prepared to reevaluate the model if it exhibits signs of degradation or overfitting.
Conclusion
Multivariate polynomial regression is a powerful tool for capturing non-linear relationships between variables. In this post, we’ve shown how to implement multivariate polynomial regression in Python using the scikit-learn library. By following the steps outlined above, you can use multivariate polynomial regression to build models that better capture complex relationships in your data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.