Multiply Every Element in a Numpy Array: A Guide
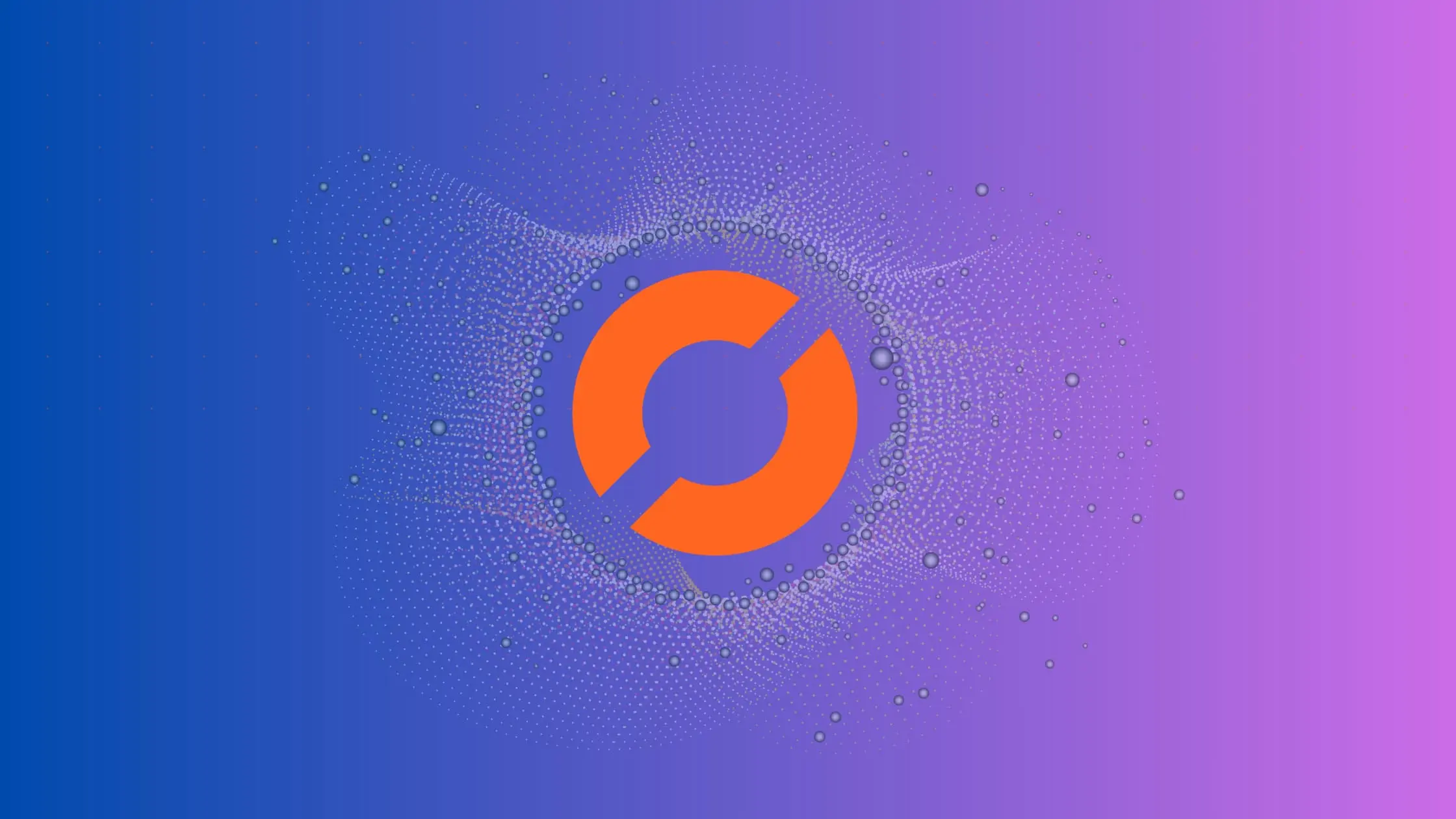
Introduction to Numpy Arrays
Numpy arrays are a core part of the Numpy library. They are grid-like data structures that can hold values, similar to lists in Python. However, unlike lists, numpy arrays allow for efficient numerical operations on large amounts of data.
import numpy as np
# Create a numpy array
arr = np.array([1, 2, 3, 4, 5])
print(arr)
[1 2 3 4 5]
Multiplying Every Element in a Numpy Array
One of the most common operations in data science is element-wise multiplication, where each element in an array is multiplied by a certain value. This can be done easily in Numpy using the *
operator or the np.multiply()
function.
Using the Operator
The *
operator can be used to multiply every element in a numpy array by a scalar.
# Multiply every element in the array by 2
arr2 = arr * 2
print(arr2)
[ 2 4 6 8 10]
Using the np.multiply() Function
The np.multiply()
function can also be used to perform element-wise multiplication. This function takes two arguments: the array and the scalar to multiply with.
# Multiply every element in the array by 2
arr3 = np.multiply(arr, 2)
print(arr3)
[ 2 4 6 8 10]
Both methods will give the same result. The choice between the two often comes down to personal preference and the specific requirements of your code.
Multiplying Two Numpy Arrays Element-Wise
Numpy also allows for element-wise multiplication between two arrays of the same shape. This can be done using the *
operator or the np.multiply()
function.
# Create a second numpy array
arr4 = np.array([6, 7, 8, 9, 10])
# Multiply the two arrays element-wise
arr5 = arr * arr4
print(arr5)
# Using np.multiply()
arr6 = np.multiply(arr, arr4)
print(arr6)
Again, both methods will give the same result.
[ 6 14 24 36 50]
Conclusion
Numpy provides a powerful and efficient way to perform operations on large amounts of data. Whether you’re multiplying every element in an array by a scalar or performing element-wise multiplication between two arrays, Numpy has you covered.
Remember, the key to efficient data science in Python is understanding and effectively using the tools at your disposal. Numpy is one such tool, and mastering it will greatly enhance your data science capabilities.
Further Reading
If you’re interested in learning more about Numpy and its capabilities, check out the official Numpy documentation. It’s a comprehensive resource that covers everything you need to know about this powerful library.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.