Linear Regression with Pandas Dataframe
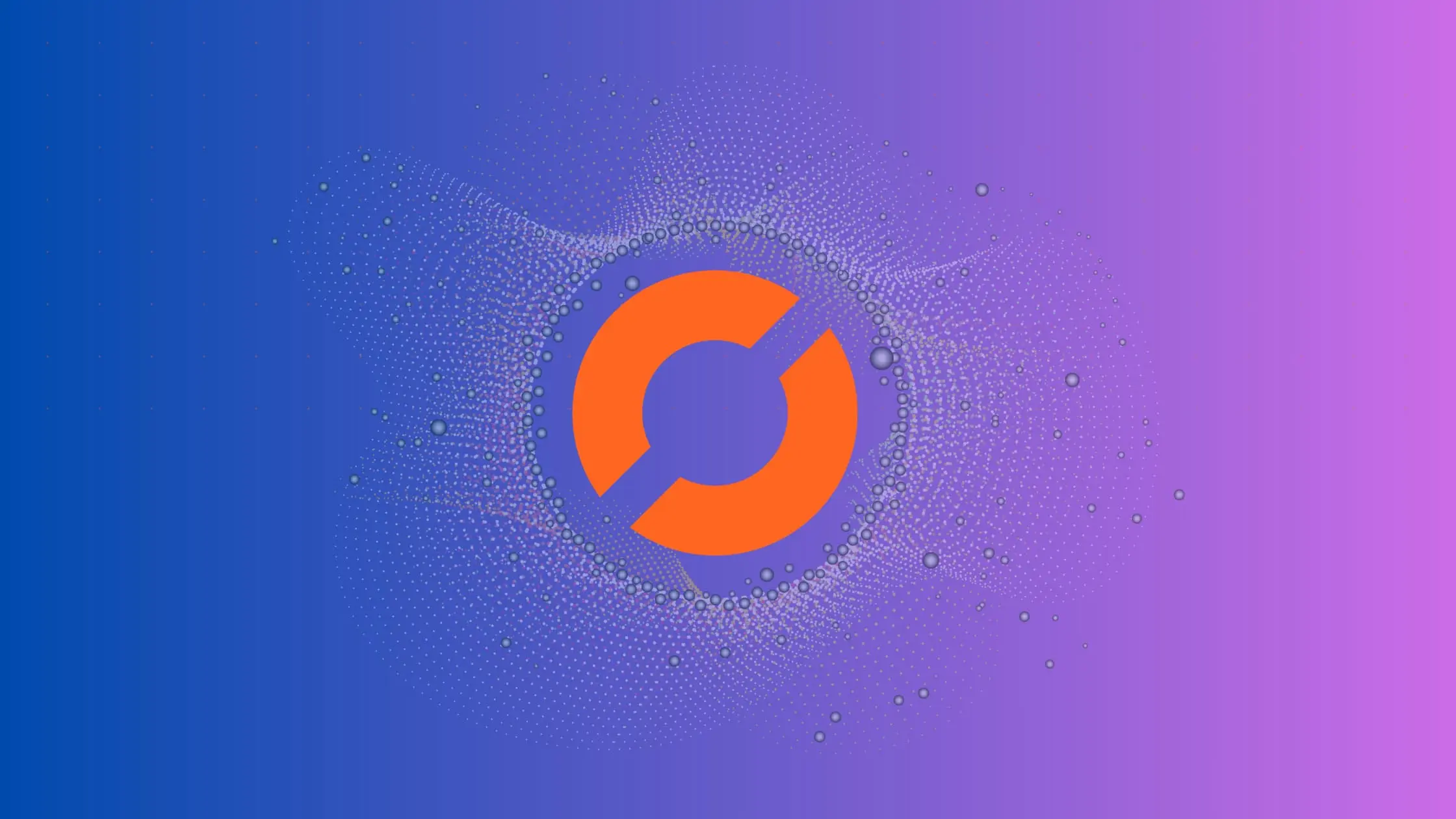
Linear Regression with Pandas Dataframe
As a data scientist or software engineer, you are likely to work with large amounts of data and need to extract insights from it. One of the most common tasks in data science is to predict a continuous variable based on one or more features. Linear regression is a popular and powerful tool for this purpose, and with the help of pandas, it becomes even easier to perform linear regression on your data.
What is Linear Regression?
Linear regression is a statistical method used to model the relationship between a dependent variable and one or more independent variables. The goal of linear regression is to find the best line that fits the data in a way that minimizes the error between the predicted values and the actual values.
In its simplest form, linear regression can be represented by the formula:
y = mx + b
where y
is the dependent variable, x
is the independent variable, m
is the slope of the line, and b
is the y-intercept.
How to Perform Linear Regression with Pandas Dataframe
Performing linear regression with pandas is a simple process that can be broken down into four steps:
- Load the data into a pandas dataframe
- Prepare the data for linear regression by separating the dependent variable and the independent variable(s)
- Create a linear regression model using the
sklearn
library - Train the model and evaluate its performance
Step 1: Load the Data into a Pandas Dataframe
Start by loading your data into a pandas dataframe. The read_csv
function is handy for reading CSV files and creating a dataframe.
import pandas as pd
data = pd.read_csv("D:\SamNewLocation\Desktop\data.csv", delimiter=';')
print(data)
Make sure to replace “D:\SamNewLocation\Desktop\data.csv” with the actual path to your CSV file.
If your CSV file is in the same directory as your script or notebook, you can simply specify the file name without the full path:
data = pd.read_csv("data.csv")
OUTPUT :
x y
0 1 2
1 2 4
2 3 5
3 4 4
4 5 5
Step 2: Prepare the Data for Linear Regression
Prepare the data by separating the dependent variable and independent variable(s). For example, let’s assume we want to predict the ‘Gender’ variable based on the ‘Age’ variable.
x = data[['x']]
y = data['y']
Step 3: Create a Linear Regression Model using sklearn
Now that we have our data separated, we can create a linear regression model using the sklearn
library. sklearn
is a popular machine learning library that provides tools for data preprocessing, model selection, and evaluation.
from sklearn.linear_model import LinearRegression
model = LinearRegression()
Step 4: Train the Model and Evaluate its Performance
Train the model using the fit
method and evaluate it’s performance using the score
method, which returns the R-squared value.
# Train the model
model.fit(x, y)
# Evaluate the model
r2_score = model.score(x, y)
print(f"R-squared value: {r2_score}")
OUTPUT :
R-squared value: 0.6000000000000001
The R-squared
value measures how well the linear regression model fits the data, ranging from 0 to 1, where 1 indicates a perfect fit
.
Conclusion
In conclusion, linear regression is a powerful tool for predicting continuous variables. By following these four simple steps, you can easily perform linear regression on your data using pandas and sklearn
. Whether you are a data scientist or a software engineer, mastering linear regression is a valuable skill that will enhance your effectiveness as a data analyst.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.