Improving Tensorflow Image Classifier Accuracy
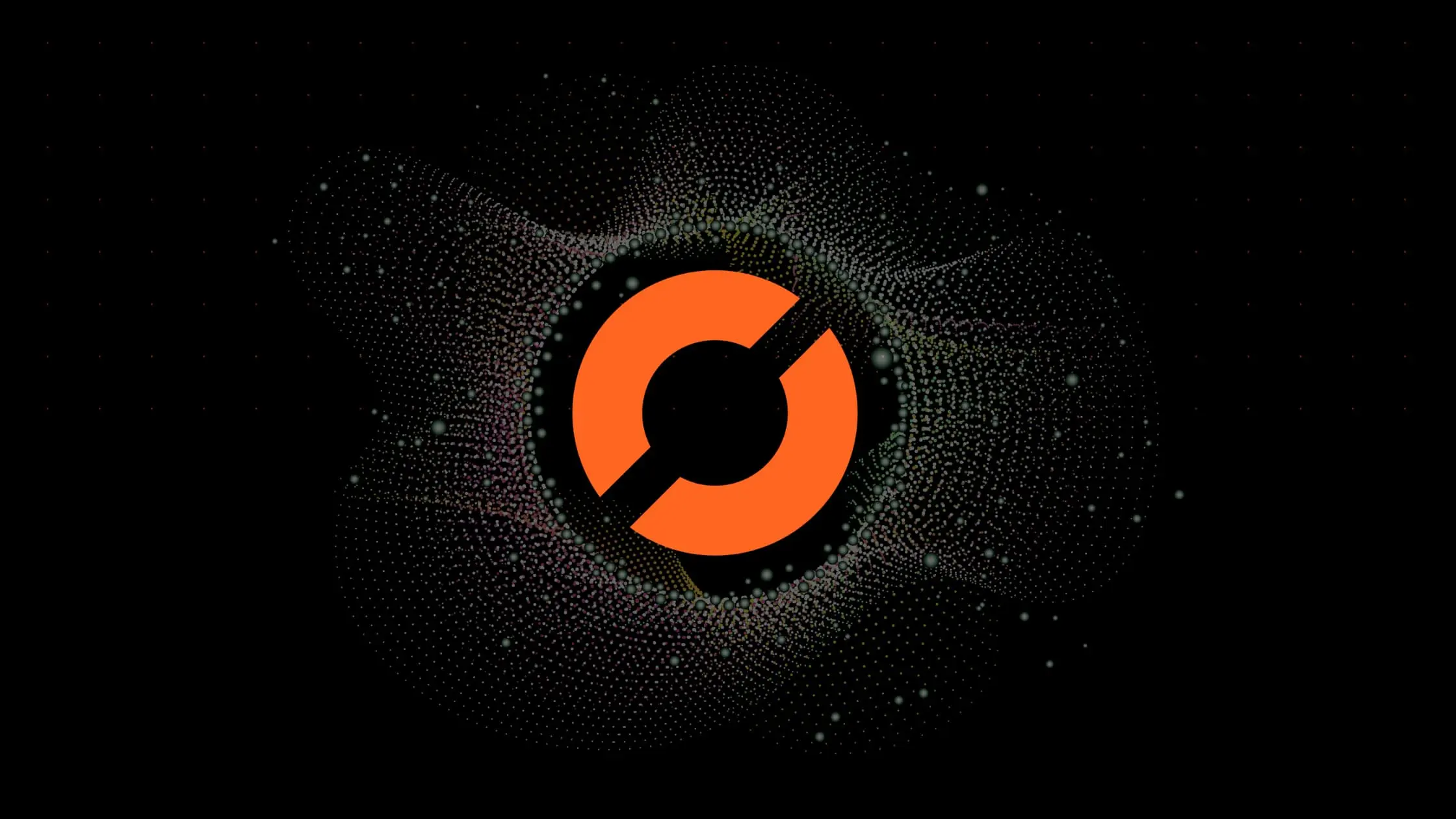
As a data scientist or software engineer, building image classifiers is a common task. Tensorflow, an open-source machine learning library, is a popular tool for building image classifiers. However, achieving high accuracy in image classification can be challenging. In this article, we will explore various techniques for improving the accuracy of Tensorflow image classifiers.
Table of Contents
- What Is Tensorflow Image Classifier?
- How to Improve Tensorflow Image Classifier Accuracy?
- Common Errors and Handling
- Conclusion
What Is Tensorflow Image Classifier?
Tensorflow Image Classifier is a machine learning model that can recognize and classify images. It is based on convolutional neural networks (CNNs), which are a type of deep neural network that can process images. Tensorflow Image Classifier uses a pre-trained CNN model, such as Inception v3 or ResNet, as a starting point. Then, it fine-tunes the model on a new set of images to improve its accuracy.
How to Improve Tensorflow Image Classifier Accuracy?
1. Increase the Size of the Training Data
The amount of training data is a crucial factor in determining the accuracy of a machine learning model. In the case of image classification, more images mean that the model has more examples to learn from, which can improve its accuracy. Therefore, increasing the size of the training data is one of the best ways to improve the accuracy of a Tensorflow image classifier. You can use data augmentation techniques, such as flipping, rotating, and cropping images, to increase the size of the training data.
Here is the code for Data Augmentation:
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# Create an ImageDataGenerator with augmentation parameters
datagen = ImageDataGenerator(
rotation_range=40,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
fill_mode='nearest'
)
# Load and preprocess an image for data augmentation
img_path = 'path/to/your/image.jpg'
img = image.load_img(img_path, target_size=(224, 224))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
# Generate augmented images
i = 0
for batch in datagen.flow(x, batch_size=1, save_to_dir='path/to/save', save_prefix='aug', save_format='jpeg'):
i += 1
if i > 5: # Generate 5 augmented images
break
2. Fine-Tune Pre-Trained Models for Task Optimization
A pre-trained CNN model, such as Inception v3 or ResNet, has already learned to recognize various features in images. However, it may not be optimized for the specific task of image classification that you are working on. Therefore, fine-tuning the pre-trained model on your dataset can significantly improve the accuracy of the Tensorflow image classifier. You can freeze the early layers of the pre-trained model and train only the last few layers on your dataset.
3. Leverage Transfer Learning for Improved Performance
Transfer learning is a technique that involves using a pre-trained model as a starting point for a new task. In the case of Tensorflow image classification, you can use a pre-trained model, such as Inception v3 or ResNet, as a starting point and then fine-tune it on your dataset. Transfer learning can significantly improve the accuracy of the Tensorflow image classifier, especially if you have limited training data.
import tensorflow as tf
from tensorflow.keras.applications import VGG16
from tensorflow.keras.preprocessing import image
from tensorflow.keras.applications.vgg16 import preprocess_input, decode_predictions
import numpy as np
# Load the pre-trained VGG16 model
base_model = VGG16(weights='imagenet')
# Load and preprocess an image for classification
img_path = 'path/to/your/image.jpg'
img = image.load_img(img_path, target_size=(224, 224))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
x = preprocess_input(x)
# Make predictions
predictions = base_model.predict(x)
decoded_predictions = decode_predictions(predictions)
# Display the top predictions
for i, (imagenet_id, label, score) in enumerate(decoded_predictions[0]):
print(f"{i + 1}: {label} ({score:.2f})")
4. Implement Regularization Techniques to Prevent OverfittingUse Regularization Techniques
Overfitting is a common problem in machine learning, where the model performs well on the training data but poorly on the test data. Regularization techniques, such as dropout and L2 regularization, can prevent overfitting and improve the generalization of the Tensorflow image classifier. You can add dropout layers or apply L2 regularization to the last few layers of the model.
5. Use Ensembling Techniques
Ensembling is a technique that involves combining multiple models to improve the accuracy of the final model. In the case of Tensorflow image classification, you can train multiple models with different architectures or hyperparameters and then combine their predictions to get the final prediction. Ensembling can significantly improve the accuracy of the Tensorflow image classifier, especially if you have limited training data.
Common Errors and Handling:
Error 1: Overfitting
Overfitting occurs when the model learns to perform well on the training data but fails to generalize to new, unseen data.
Solution:
Implement dropout layers to reduce overfitting.
Copy code
model = tf.keras.Sequential([
# ... other layers ...
tf.keras.layers.Dropout(0.5),
# ... other layers ...
])
Error 2: Learning Rate Issues
Choosing an inappropriate learning rate can lead to slow convergence or model divergence.
Solution:
Implement a learning rate schedule or use adaptive learning rate methods like Adam.
optimizer = tf.keras.optimizers.Adam(learning_rate=0.001)
Conclusion
Improving the accuracy of a Tensorflow image classifier requires a combination of techniques, such as increasing the size of the training data, fine-tuning the pre-trained model, using transfer learning, applying regularization techniques, and using ensembling techniques. By using these techniques, you can significantly improve the accuracy of your Tensorflow image classifier and make it more robust and reliable.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.