How to Write a Pandas Dataframe to a txt File
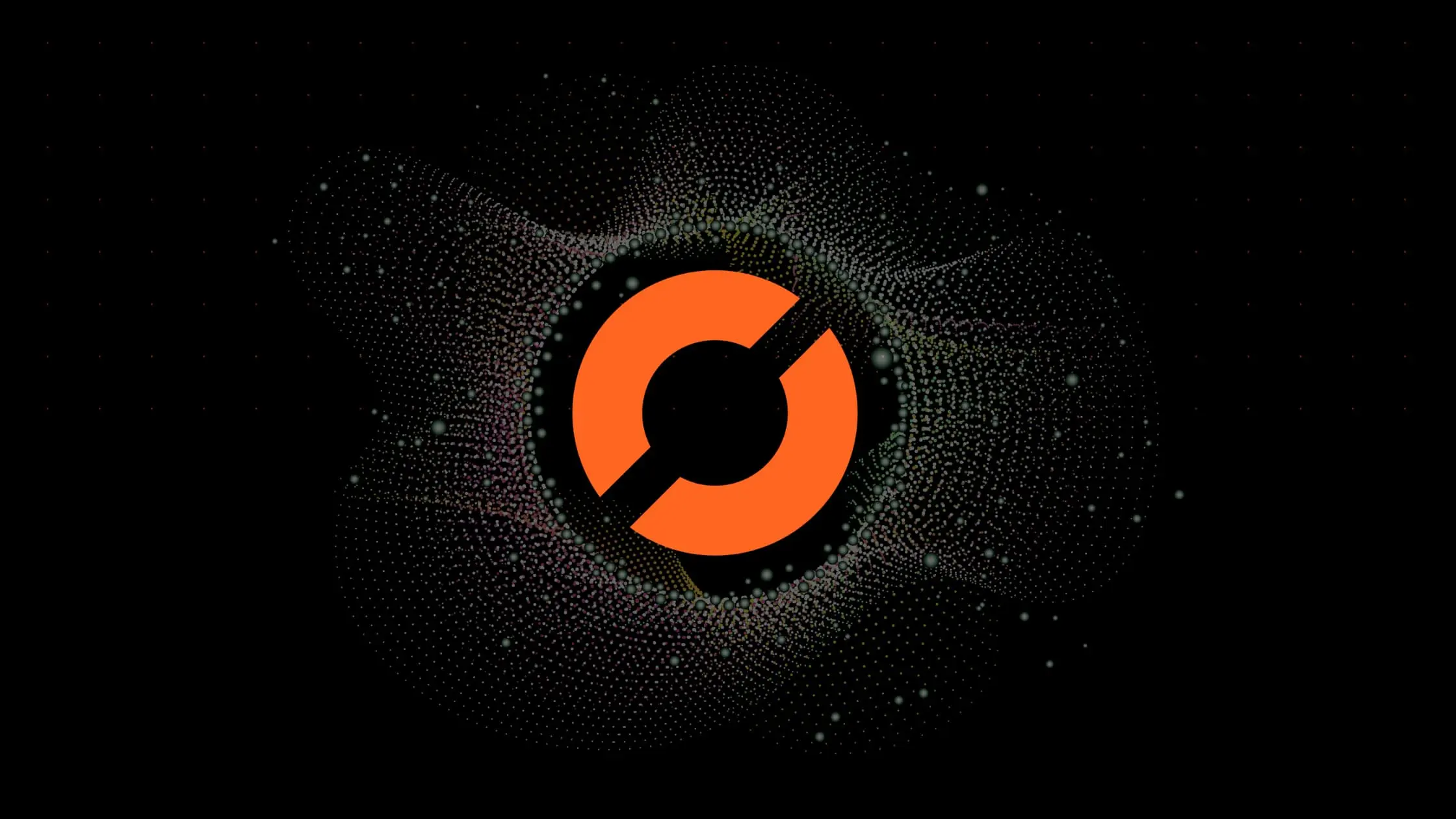
How to Write a Pandas Dataframe to a txt File
As a data scientist or software engineer, you may find yourself working with large datasets in Pandas. One of the common tasks is to save the data in a text file format, such as .txt, for further processing or analysis. In this article, we will discuss how to write a Pandas dataframe to a .txt file.
What is a Pandas Dataframe?
A Pandas dataframe is a two-dimensional table-like data structure with rows and columns. It is one of the most commonly used data structures in data science and machine learning. Pandas provides many functions to manipulate, clean, and analyze data in a dataframe.
Writing a Pandas Dataframe to a .txt File
To write a Pandas dataframe to a .txt file, we can use the to_csv()
function in Pandas. This function is versatile and can be used to write data to various file formats, including .txt.
Step 1: Create a Pandas Dataframe
Before we can write a Pandas dataframe to a .txt file, we need to create a dataframe first. For the purpose of this article, let’s create a simple dataframe with three columns: name, age, and gender.
import pandas as pd
data = {'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'gender': ['female', 'male', 'male']}
df = pd.DataFrame(data)
Step 2: Write the Dataframe to a .txt File
Now that we have a dataframe, we can write it to a .txt file using the to_csv()
function in Pandas. We need to specify the file path and name of the .txt file as the argument of the function.
df.to_csv('data.txt', sep='\t', index=False)
In the above example, we specify the file name as data.txt
. We also specify the separator as a tab character (\t
) using the sep
parameter. This will ensure that the data is written in a tab-separated format. Finally, we set the index
parameter to False
to exclude the row index from the output.
Conclusion
In this article, we have discussed how to write a Pandas dataframe to a .txt file using the to_csv()
function in Pandas. We created a simple dataframe with three columns, specified the file name and path, and set the delimiter to a tab character. Writing data to a text file is a common task in data science and machine learning. By following the steps in this article, you can easily write your Pandas dataframe to a .txt file for further processing or analysis.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.